
Email Attachments in Laravel: No Need to Save Files
In web development, sending email attachments is a common requirement, whether it's for sending invoices, reports, or any other document. Laravel, being a versatile PHP framework, provides native support for sending emails. However, many developers often assume that they need to store files on disk before attaching them to an email. Contrary to this belief, Laravel offers a convenient way to attach files without the need for file storage. In this guide, we'll explore how to achieve this with example code and highlight key points along the way.
Key Points:
- No File Storage Required: You can attach files directly from memory without saving them to disk, reducing unnecessary overhead in file management.
- Stream Attachments: Laravel provides functionality to stream file contents directly into an email attachment, eliminating the need for temporary file storage.
- Efficiency: Streamlining the attachment process enhances the efficiency of your application by reducing disk I/O operations and minimizing storage requirements.
Example Code:
use Illuminate\Support\Facades\Mail;
use Illuminate\Support\Facades\Storage;
use Illuminate\Support\Str;
use Illuminate\Mail\Mailable;
class SendEmailWithAttachment extends Mailable
{
protected $fileContents;
public function __construct($fileContents)
{
$this->fileContents = $fileContents;
}
public function build()
{
return $this->view('emails.attachment')
->attachData($this->fileContents, 'document.pdf', [
'mime' => 'application/pdf',
]);
}
}
In this example, we create a Mailable class SendEmailWithAttachment
responsible for composing the email. The constructor accepts the file contents as a parameter. In the build method, we specify the view for the email (emails.attachment)
and attach the file contents using attachData
method. We pass the file contents, file name (document.pdf)
, and MIME type (application/pdf)
as arguments.
To use this Mailable class and send an email with an attachment, you can do the following:
use App\Mail\SendEmailWithAttachment;
$fileContents = $request->file('attachment');
Mail::to('[email protected]')
->send(new SendEmailWithAttachment($fileContents));
Replace $fileContents
with the actual contents of the file you want to attach. This could be fetched from a database, generated dynamically, or retrieved from an external API.
Conclusion:
By leveraging Laravel's built-in features, you can effortlessly attach files to emails without the need for file storage. This approach not only simplifies your code but also enhances the performance of your application by reducing unnecessary disk operations. Whether you're sending invoices, reports, or any other documents, this method provides a streamlined solution for email attachments in Laravel.
Happy Coding!
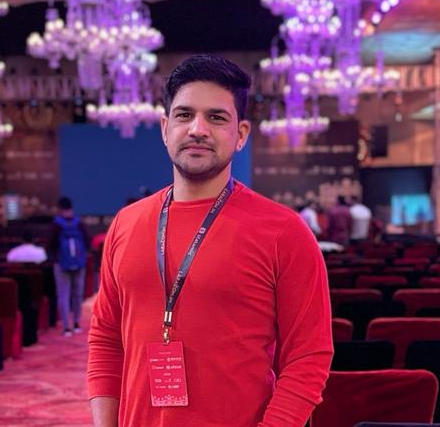
Author: Sonu Singh
With over 7 years of dedicated experience in web development, I've traversed through the dynamic landscape of digital technology, honing my skills and expertise across various frameworks and content management systems. My journey began with the exploration of frameworks like CodeIgniter, CakePHP, Yii, and eventually, I found my passion in Laravel - a framework that resonated deeply with my love for elegant, efficient code. Throughout my career, I've had the privilege to contribute to numerous projects, ranging from small-scale ventures to large, enterprise-level solutions. Each project has been a unique opportunity to push boundaries, solve intricate challenges, and deliver innovative solutions tailored to meet client needs. One of my proudest accomplishments includes the development of a SaaS-based project using Laravel and MySQL. This venture not only showcased my technical prowess but also demonstrated my ability to conceptualize, design, and execute scalable solutions that drive business growth. In addition to my proficiency in development, I've also delved into server deployment and query optimization, ensuring that every aspect of a project - from its codebase to its infrastructure - is optimized for peak performance and reliability.
Recent Posts
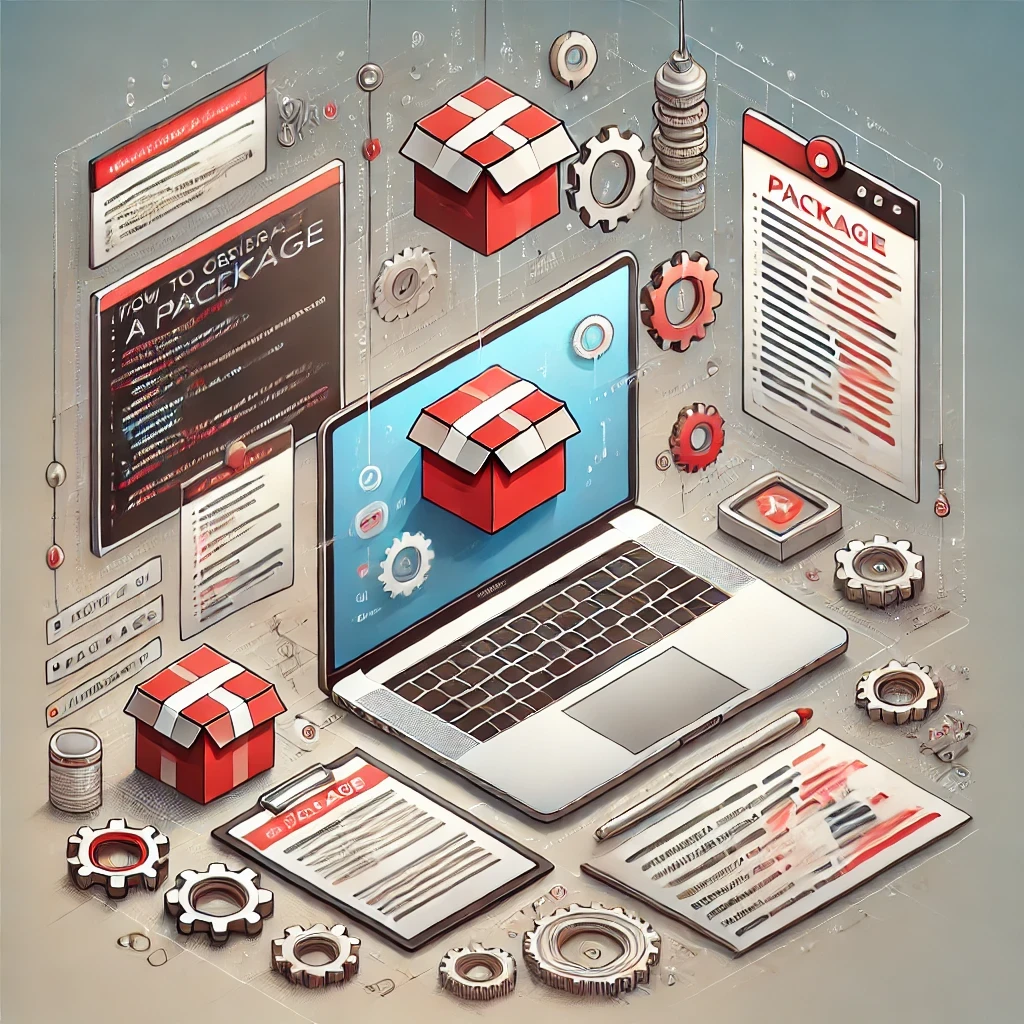
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
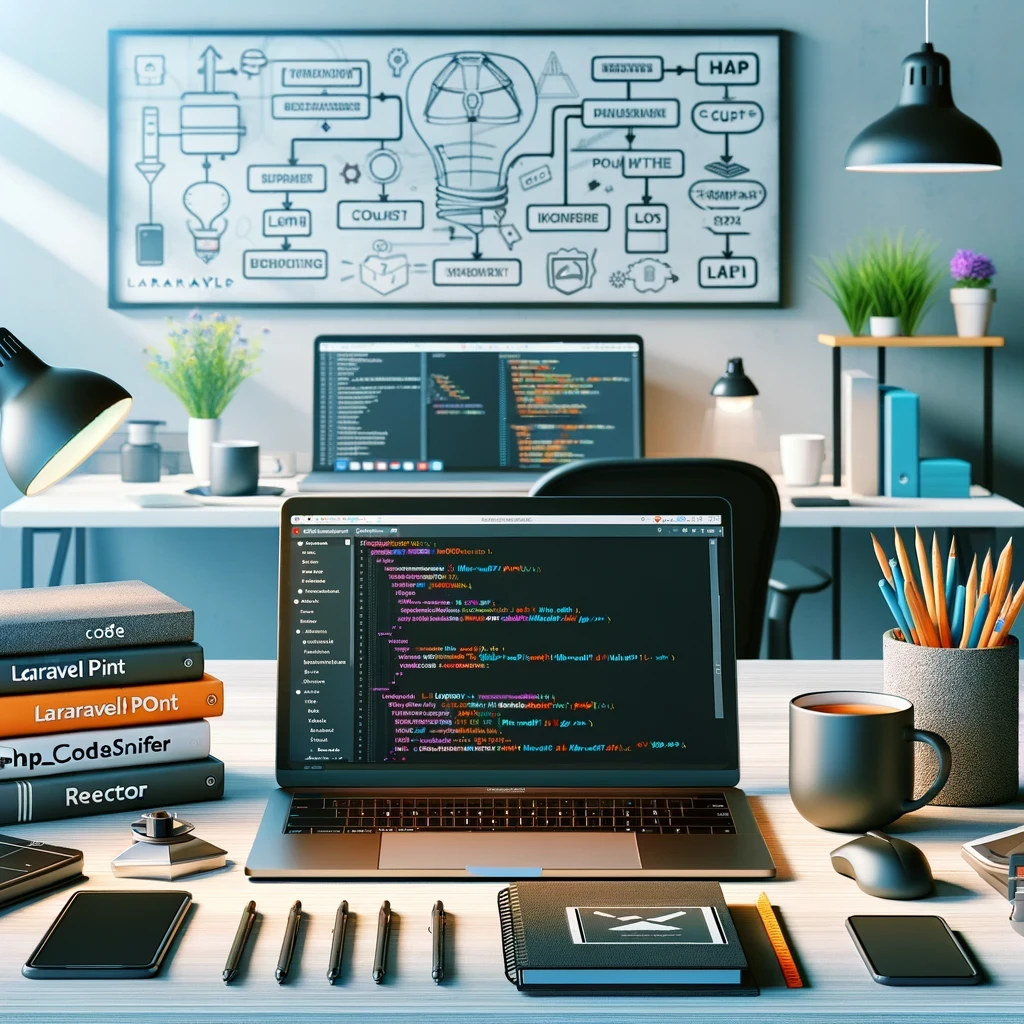
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
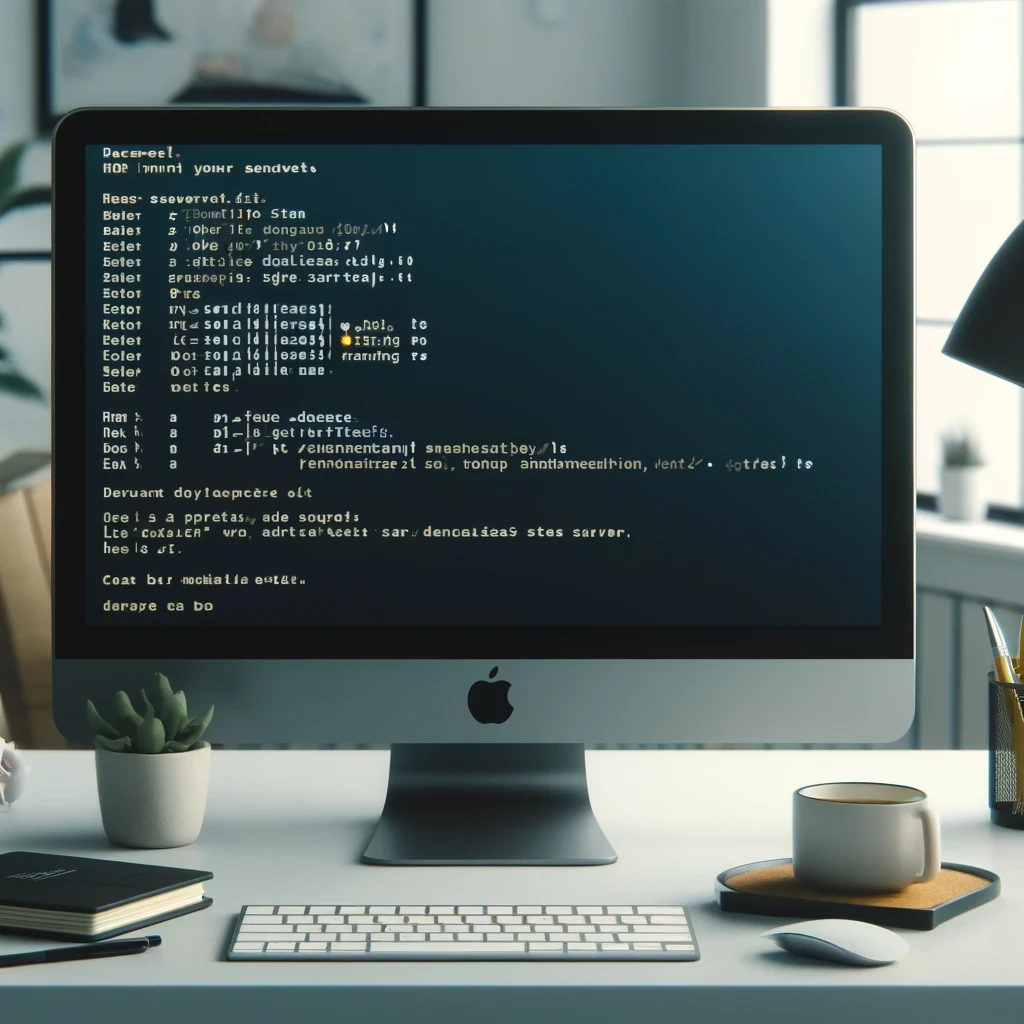
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
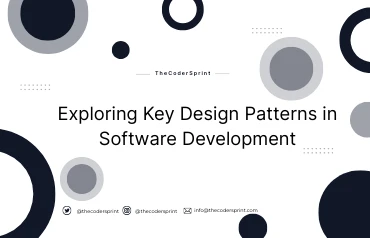
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
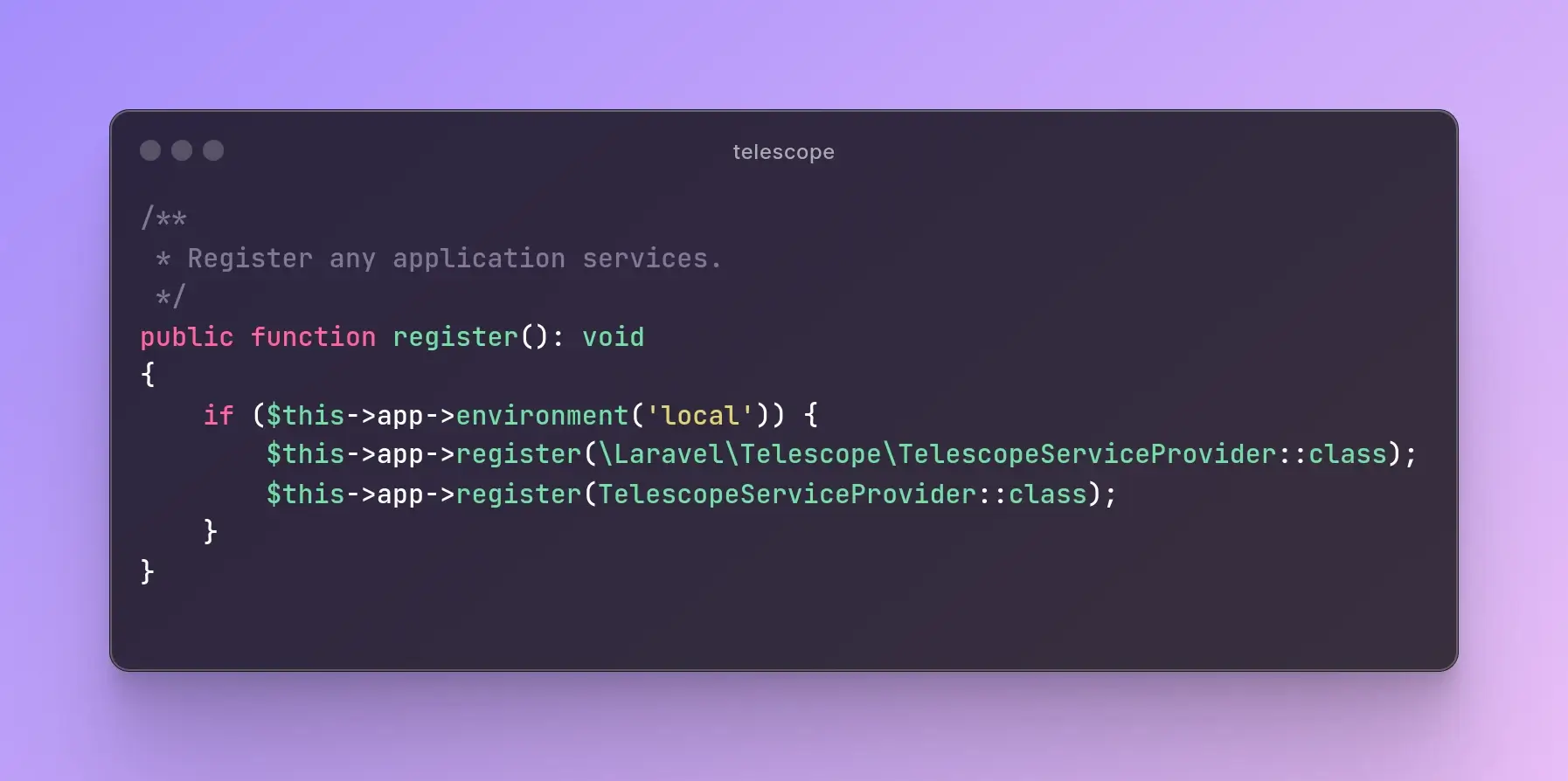
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles