Notifications Filament provides a Notification class that allows you to send notifications to your users. These notifications can be customized to meet the needs of your application.
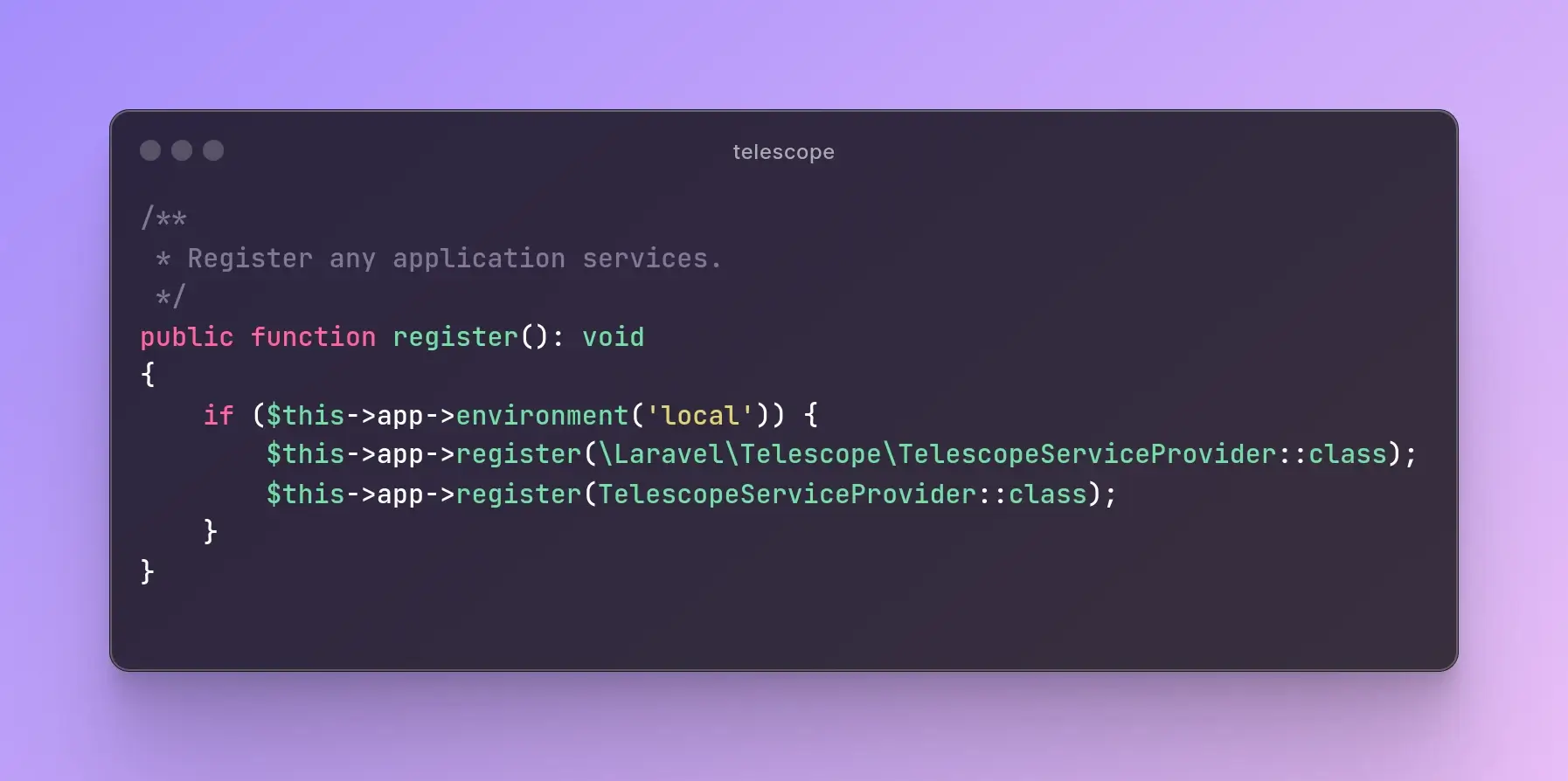
Laravel Telescope: Debugging Made Easy (Part - 2)
However, with great power comes great responsibility. Telescope, by default, is accessible in local development environments. This can be a security risk in production environments where unauthorized access could expose sensitive application data.
In our previous blog post, we explored the power of Laravel Telescope, a debugging and monitoring tool for your Laravel applications. We learned about its installation and basic configuration, allowing you to gain valuable insights into your application's inner workings.
This blog post dives into Laravel's robust authorization features to restrict access to the Telescope dashboard, ensuring only authorized users can utilize its functionalities.
Understanding Laravel Authorization
Laravel provides a powerful authorization system that allows you to define who can access specific resources within your application. This system utilizes gates
, which are closures that determine whether a user is authorized for a given action.
Securing Telescope with Gates
By leveraging gates, we can control access to the Telescope dashboard. Here's how:
- Defining the Gate:
Open your App\Providers\TelescopeServiceProvider
class and locate the boot
method. Inside this method, use Laravel's Gate::define
method to define a new gate named viewTelescope
. This gate will determine if a user is authorized to access Telescope.
public function boot()
{
parent::boot();
Gate::define('viewTelescope', function (User $user) {
// Your access control logic goes here
});
}
-
Implementing Access Control Logic:
Within the
viewTelescope
gate closure, you can define the criteria for granting access.
These are the main approaches:
-
Role-Based Access Control (RBAC):
Allow only administrators (
admin
role) to access Telescope:
Gate::define('viewTelescope', function (User $user) {
return $user->hasRole('admin');
});
Allow both administrators (admin
) and developers (developer
) roles to access Telescope:
Gate::define('viewTelescope', function (User $user) {
return $user->hasRole('admin') || $user->hasRole('developer');
});
- Permission-Based Access Control (PBAC):
Create a specific permission called view_telescope
.
// Define permission in your migration or seeder
Permission::create(['name' => 'view_telescope']);
// Assign permission to users
$user->givePermissionTo('view_telescope');
Assign this permission only to users who should access Telescope.
Gate::define('viewTelescope', function (User $user) {
return $user->hasPermission('view_telescope');
});
- Combining Roles and Permissions: You can combine role and permission checks for more granular control:
Gate::define('viewTelescope', function (User $user) {
return ($user->hasRole('admin') || $user->hasPermission('view_telescope')) && app()->environment('staging');
});
This example allows access to Telescope only on the staging
environment for users with either the admin
role or the view_telescope
permission.
- Restricting Access Based on User Data:
For more advanced scenarios, you might want to restrict access based on additional user data.
Here's a conceptual example (implement logic based on your requirements):
Gate::define('viewTelescope', function (User $user) {
if ($user->hasRole('admin')) {
return true;
}
if ($user->hasPermission('view_telescope')) {
// Check additional user data (e.g., department)
return $user->department === 'engineering';
}
return false;
});
By implementing authorization gates, you can ensure that only authorized users can access the Telescope dashboard in production environments. This safeguards your application's sensitive data while allowing authorized users to leverage Telescope's valuable debugging functionalities.
Simplifying Telescope Registration in Laravel 11
After running the telescope:install
command, you'll need to make a small adjustment to how Telescope registers itself within your Laravel application. This ensures Telescope is only accessible during local development for security reasons.
Here's a breakdown of the steps:
- Remove Unnecessary Registration:
- Open the
bootstrap/providers.php
file. - Locate the line that registers
TelescopeServiceProvider
. Remove this line.
<?PHP
return [
App\Providers\AppServiceProvider::class,
\Laravel\Telescope\TelescopeServiceProvider::class // Remove or Comment this line
];
- Register Telescope Only in Local Development:
- Open the
App\Providers\AppServiceProvider.php
file. - Find the
register
method. - Inside
register
, add the following code:
/**
* Register any application services.
*/
public function register(): void
{
if ($this->app->environment('local')) {
$this->app->register(TelescopeServiceProvider::class);
}
}
Fine-Tuning Telescope (Optional):
- After setting up Telescope, a configuration file (
config/telescope.php
) appears. - This file lets you adjust how Telescope collects data (called "watching").
- Descriptions within the file explain each option.
- If you don't want Telescope to collect data at all, simply set the
enabled
option tofalse
.
'enabled' => env('TELESCOPE_ENABLED', true),
- Telescope stores data, but it can grow large. To keep it tidy, run the
telescope:prune
command daily (e.g., using a scheduler). This cleans up old entries.
use Illuminate\Support\Facades\Schedule;
Schedule::command('telescope:prune')->daily();
By default, all entries older than 24 hours
will be pruned. You may use the hours
option when calling the command to determine how long to retain Telescope data.
use Illuminate\Support\Facades\Schedule;
Schedule::command('telescope:prune --hours=48')->daily(); // Older than 48 hours
- At Last, you should also prevent the Telescope package from being auto-discovered by adding the following to your
composer.json
file:
"extra": {
"laravel": {
"dont-discover": [
"laravel/telescope"
]
}
},
Conclusion
Laravel Telescope is an invaluable asset for Laravel developers. Its comprehensive debugging features and insightful monitoring capabilities streamline development and troubleshooting. Remember to prioritize security by keeping Telescope out of production environments and implementing proper authorization measures. With Telescope by your side, you can build robust and efficient Laravel applications.
Happy Coding!
- Tags:
- #Laravel
- #Debugging
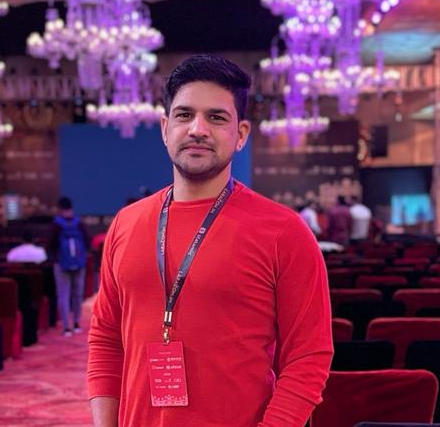
Author: Sonu Singh
With over 7 years of dedicated experience in web development, I've traversed through the dynamic landscape of digital technology, honing my skills and expertise across various frameworks and content management systems. My journey began with the exploration of frameworks like CodeIgniter, CakePHP, Yii, and eventually, I found my passion in Laravel - a framework that resonated deeply with my love for elegant, efficient code. Throughout my career, I've had the privilege to contribute to numerous projects, ranging from small-scale ventures to large, enterprise-level solutions. Each project has been a unique opportunity to push boundaries, solve intricate challenges, and deliver innovative solutions tailored to meet client needs. One of my proudest accomplishments includes the development of a SaaS-based project using Laravel and MySQL. This venture not only showcased my technical prowess but also demonstrated my ability to conceptualize, design, and execute scalable solutions that drive business growth. In addition to my proficiency in development, I've also delved into server deployment and query optimization, ensuring that every aspect of a project - from its codebase to its infrastructure - is optimized for peak performance and reliability.
Comments
Recent Posts
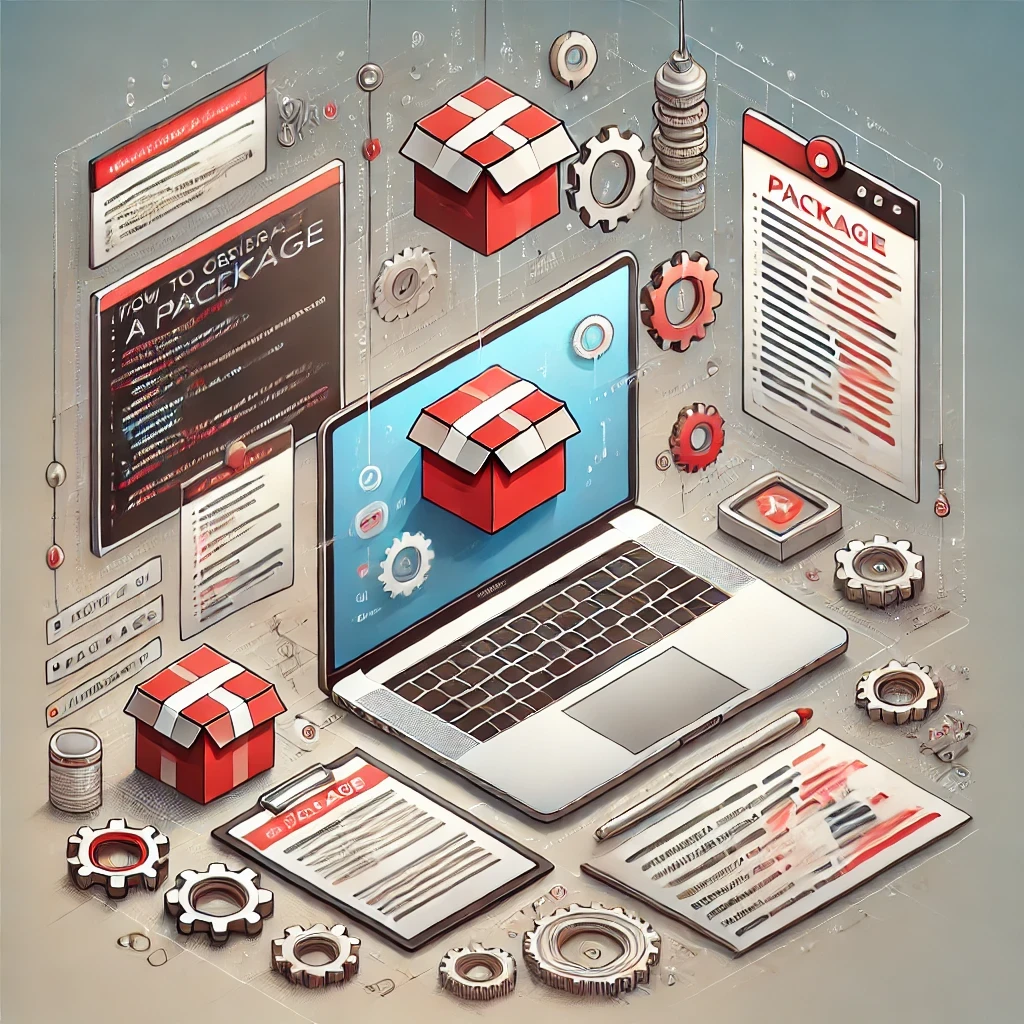
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
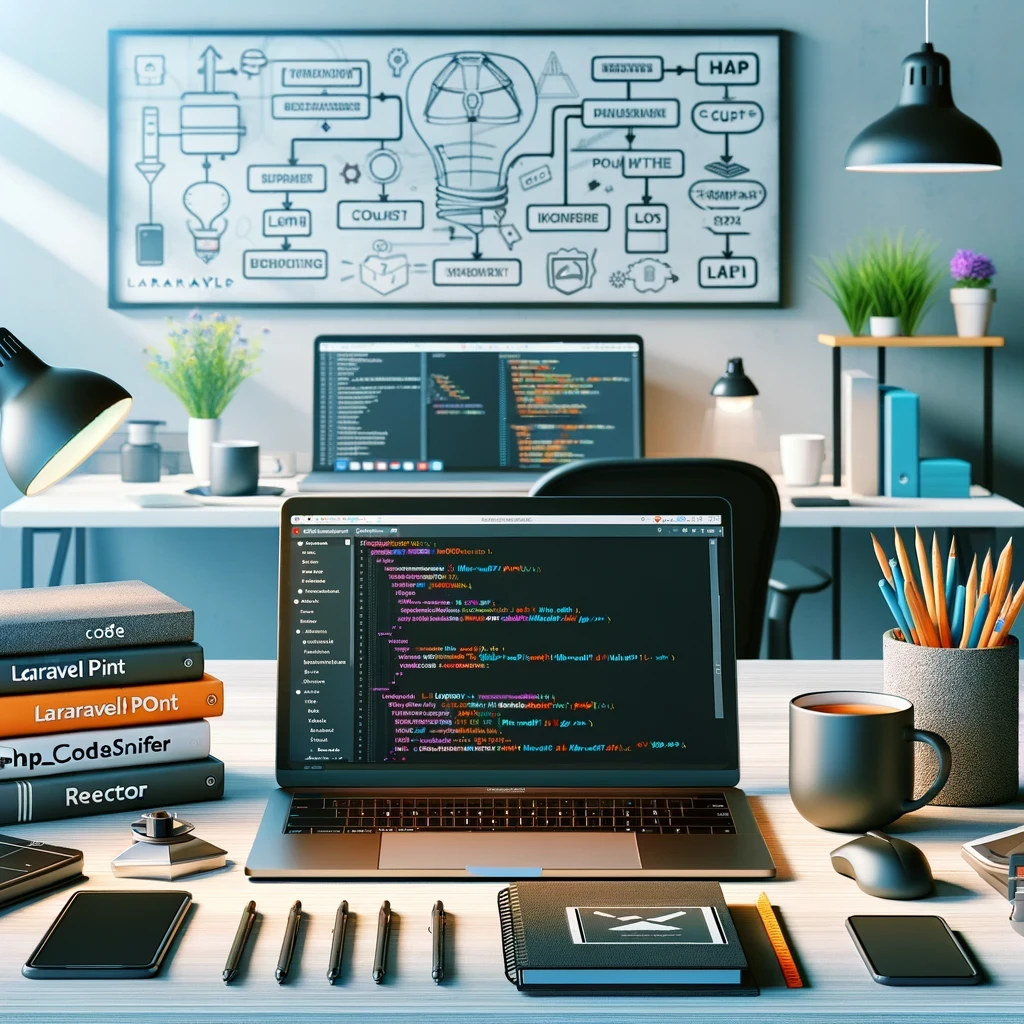
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
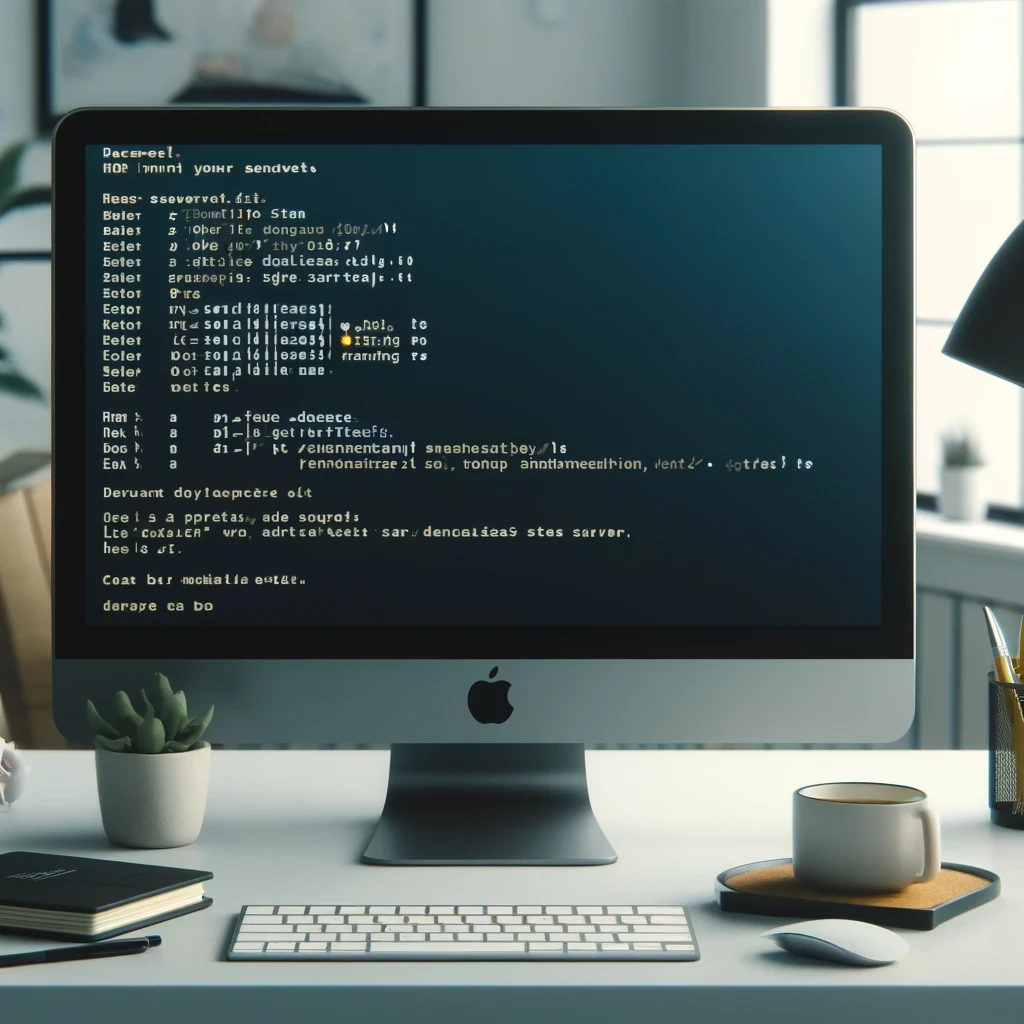
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
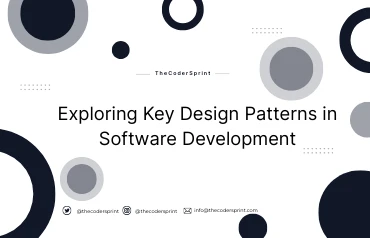
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
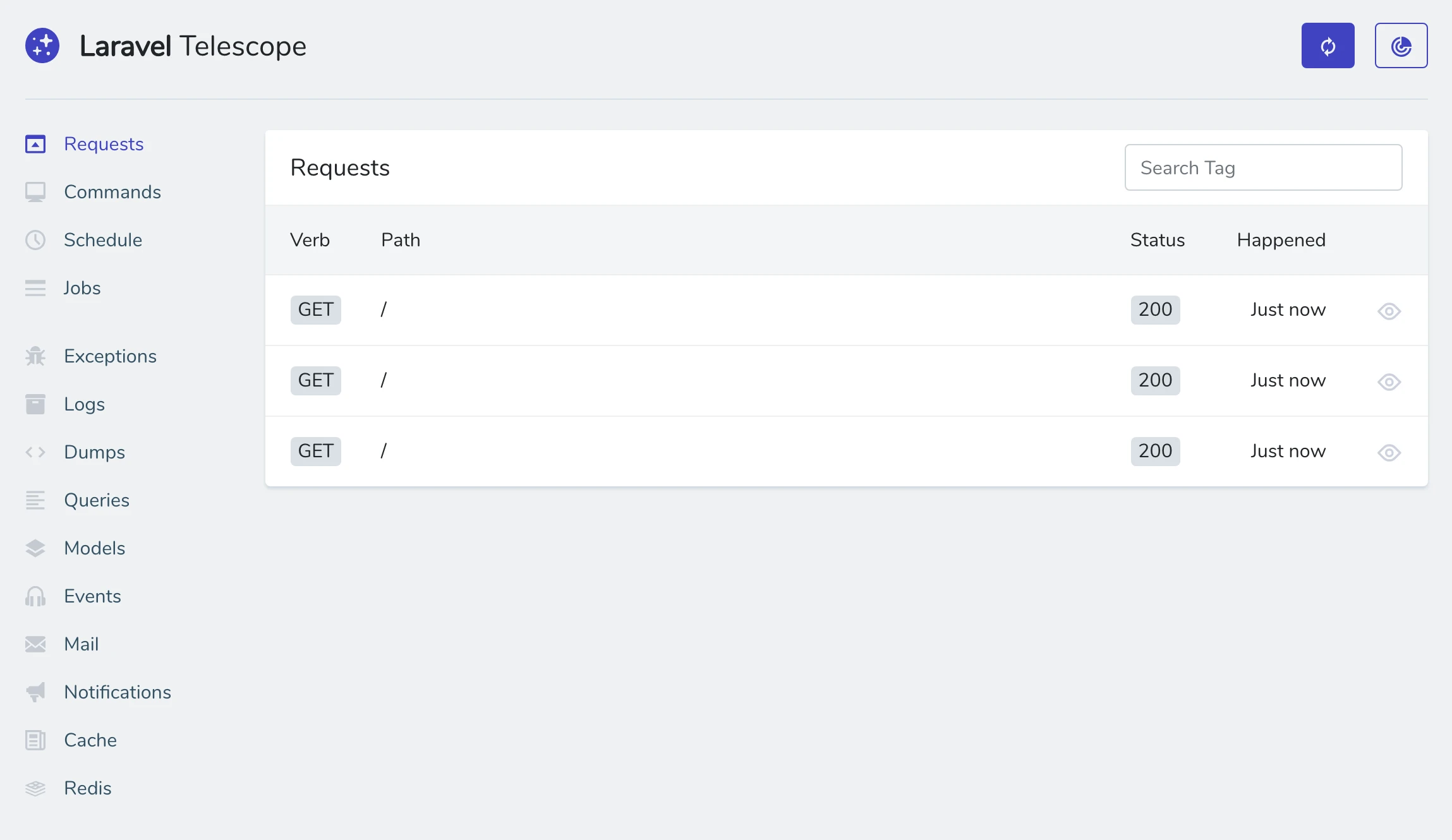
Laravel Telescope: Debugging Made Easy (Part - 1)
Sonu Singh
24 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles