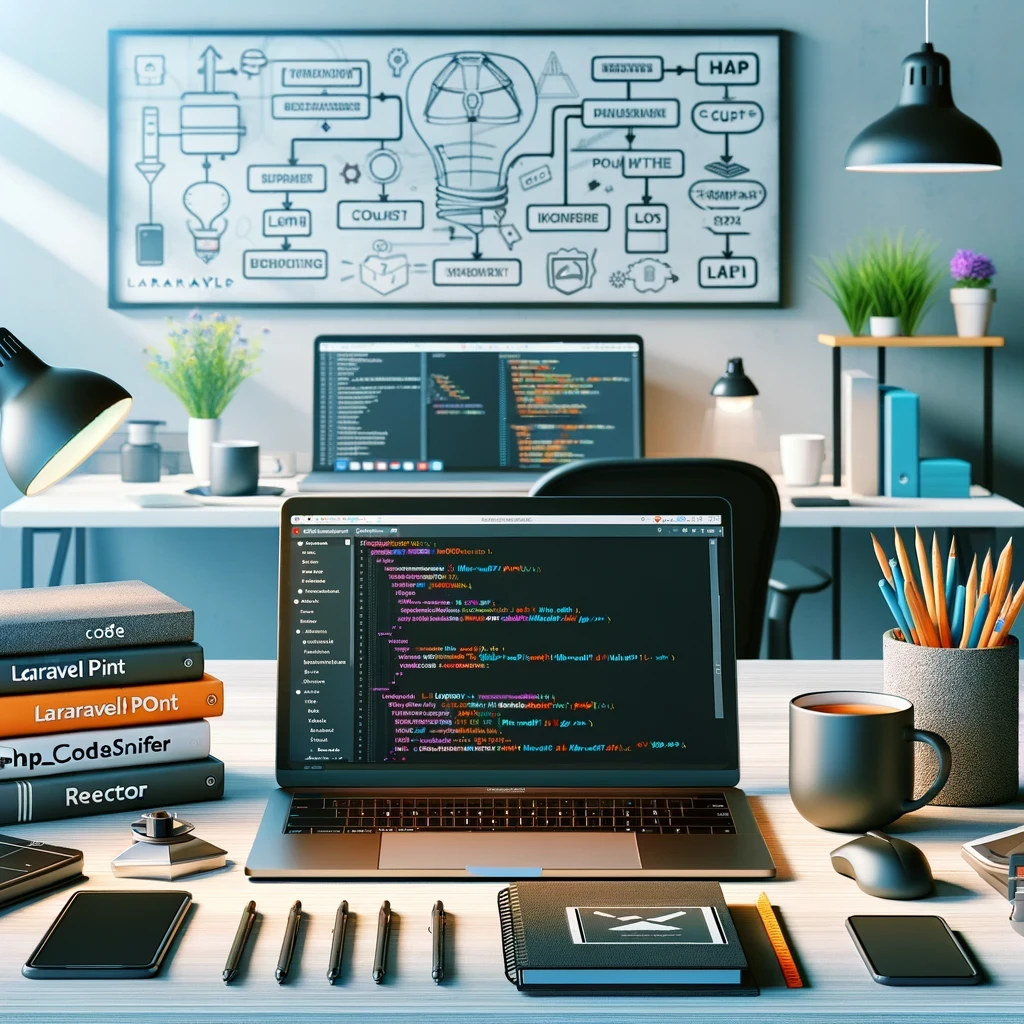
Writing Clean Code in Laravel: Principles and Tools
Introduction
Writing clean code is essential for maintaining and scaling any software project. In Laravel, clean code ensures that your application is easy to read, maintain, and extend. This blog will cover principles and practices for writing clean code in Laravel, as well as tools to help you maintain clean code.
1. Principles of Clean Code
Simplicity: Code should be simple and straightforward. Avoid unnecessary complexity.
Readability: Code should be easy to read and understand. Use meaningful names and keep functions small.
Maintainability: Code should be easy to modify and extend. Ensure your code is modular.
Consistency: Follow consistent coding standards throughout the project. Stick to Laravel's conventions.
2. Directory Structure and Organization
Laravel provides a well-defined directory structure. Use it effectively:
-
Controllers: Place controllers in the
app/Http/Controllers
directory. -
Models: Place models in the
app/Models
directory. -
Views: Place Blade templates in the
resources/views
directory. -
Services and Repositories: Create
Services
andRepositories
directories inapp
for business logic and database queries.
3. Naming Conventions
Use descriptive names for your classes, methods, and variables:
-
Classes and Files: Use PascalCase (e.g.,
UserController
). -
Methods: Use camelCase (e.g.,
getUser
). -
Variables: Use meaningful names (e.g.,
$user
,$postCount
).
4. Controllers
Keep controllers thin by delegating logic:
- Use Resource Controllers for RESTful actions.
- Move business logic to service classes or action classes.
5. Models and Eloquent
Keep your models focused on database interactions:
- Use meaningful method names (e.g.,
getActiveUsers
). - Avoid fat models by using query scopes and accessors/mutators.
- Utilize relationships and eager loading to optimize queries.
6. Services and Repositories
Separate business logic and database interactions:
- Service Classes: Handle complex business logic.
- Repositories: Abstract database queries.
- Use dependency injection for better testability.
7. Blade Templates
Keep your views clean and simple:
- Use Blade components and slots to reuse code.
- Avoid embedding complex logic in Blade templates.
8. Validation
Use Form Request classes for validation:
- Create Form Request classes using
php artisan make:request
. - Define validation rules and custom messages.
- Keep validation logic out of controllers.
9. Testing
Write tests to ensure code quality:
- Use PHPUnit for unit tests and feature tests.
- Utilize factories and seeders to create test data.
10. Tools for Clean Code
-
Larastan: A PHPStan wrapper for Laravel that brings static analysis to your Laravel codebase.
composer require --dev nunomaduro/larastan
-
PHPStan: A static analysis tool that helps you find bugs in your code.
composer require --dev phpstan/phpstan
-
Laravel Pint: An opinionated PHP code style fixer for Laravel.
composer require --dev laravel/pint
-
PHP_CodeSniffer: A tool to detect violations of a defined coding standard.
composer require --dev squizlabs/php_codesniffer
-
PHP CS Fixer: A tool to automatically fix PHP coding standards issues.
composer require --dev friendsofphp/php-cs-fixer
-
PHP Insights: Analyzes your code to provide actionable insights and improve your code quality.
composer require --dev nunomaduro/phpinsights
-
Rector: A tool for automated refactoring and upgrading of your PHP codebase.
composer require --dev rector/rector
11. Best Practices for Clean Code
Follow industry standards and tools:
- Use PSR standards.
- Use Composer and well-maintained Laravel packages.
- Perform code reviews and use static analysis tools.
12. Performance Optimization
Optimize your application for performance:
- Implement caching strategies using Redis or Memcached.
- Optimize database queries and use eager loading.
- Use tools like Laravel Debugbar and Telescope for debugging.
13. Documentation and Comments
Document your code effectively:
- Write meaningful comments and keep them updated.
- Use PHPDoc for method documentation.
- Maintain a README file with setup instructions and project overview.
Conclusion
Clean code is crucial for the success of any Laravel project. By following these practices and utilizing the mentioned tools, you can write code that is easy to read, maintain, and extend. Adopt these practices in your development workflow and strive for excellence in your code.
References
- Laravel Documentation
- PHP-FIG PSR Standards
- Clean Code: A Handbook of Agile Software Craftsmanship
- Larastan GitHub Repository
- PHPStan GitHub Repository
- Laravel Pint GitHub Repository
- PHP_CodeSniffer GitHub Repository
- PHP CS Fixer GitHub Repository
- PHP Insights GitHub Repository
- Rector GitHub Repository
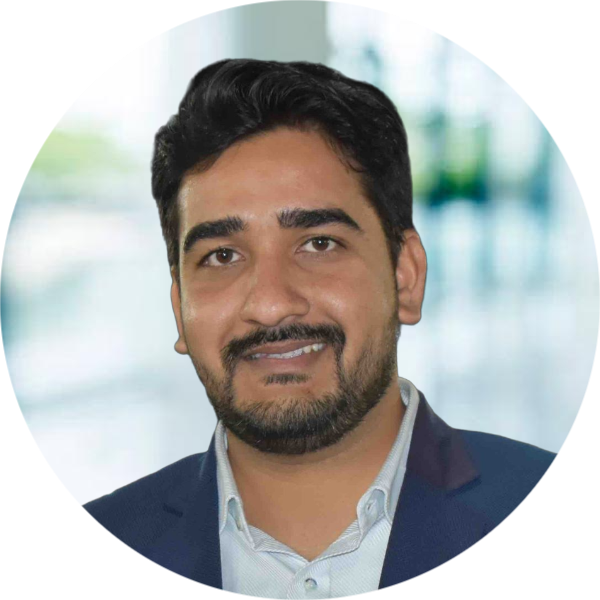
Author: Manish Kumar
I've been working with PHP for over 11 years and focusing on Laravel for the last 6 years. During this time, I've taken part in big projects and gotten to know tools like Laravel Livewire really well. Besides working on projects, I enjoy writing about Laravel on LinkedIn and Medium to share what I've learned. I'm always looking to learn more and share my knowledge with others. Right now, I'm diving into new areas like using Docker to make web apps run better and exploring how to build them using microservices. My goal is to keep learning and help others do the same in the world of web development.
Recent Posts
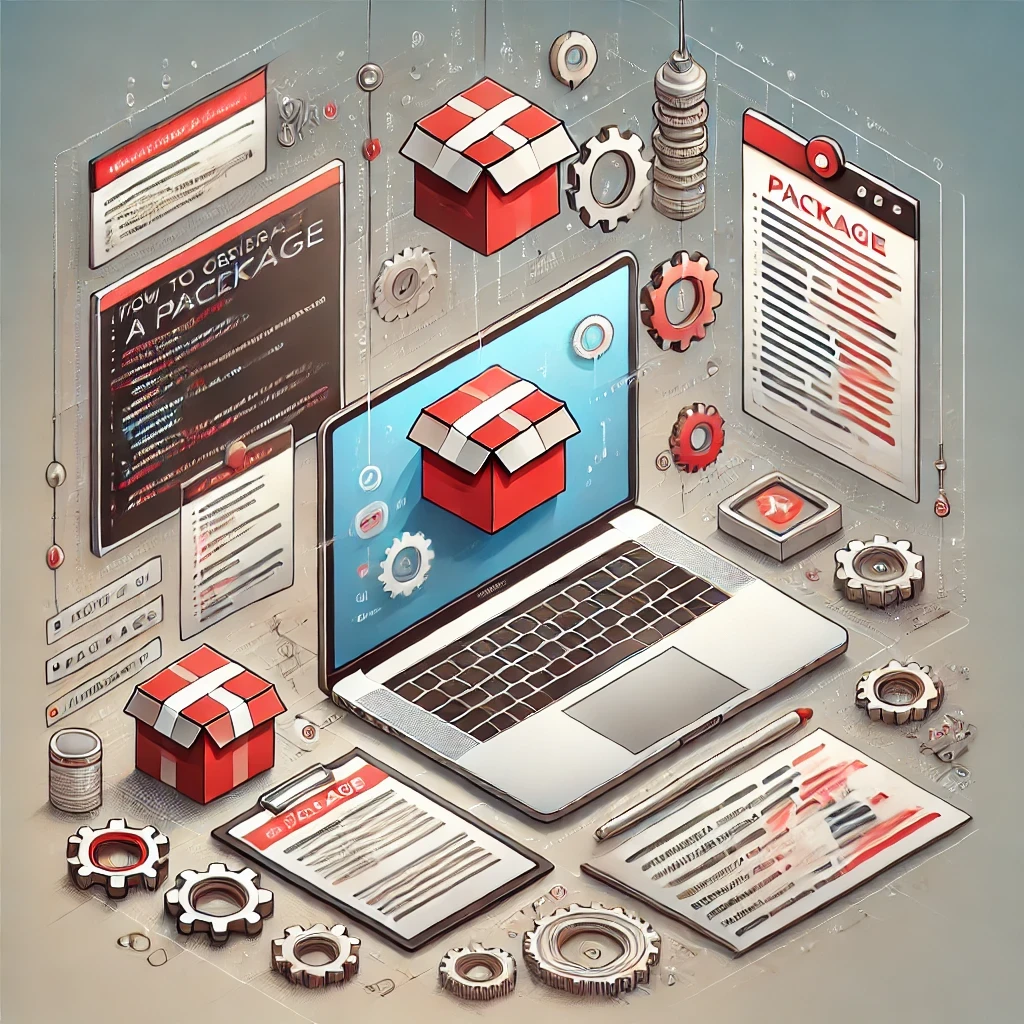
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
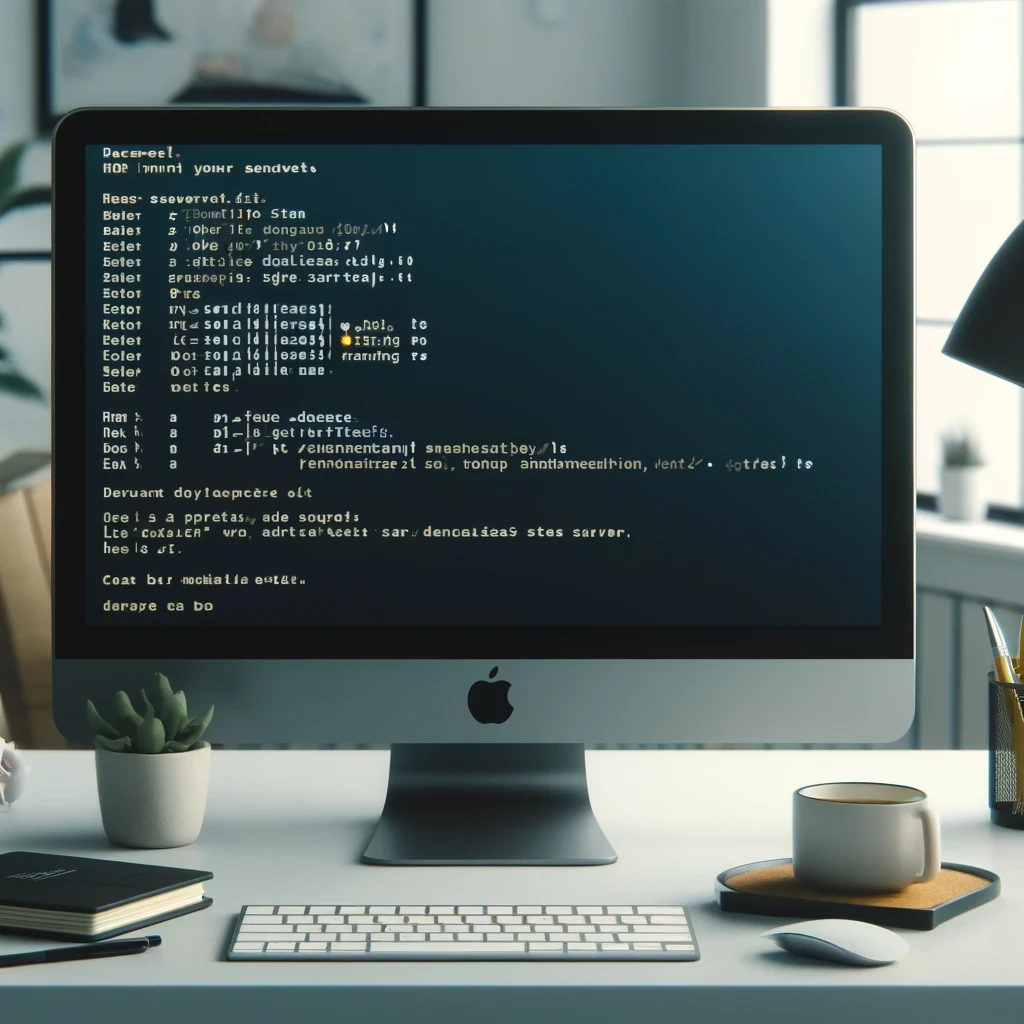
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
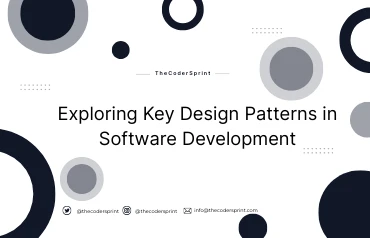
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
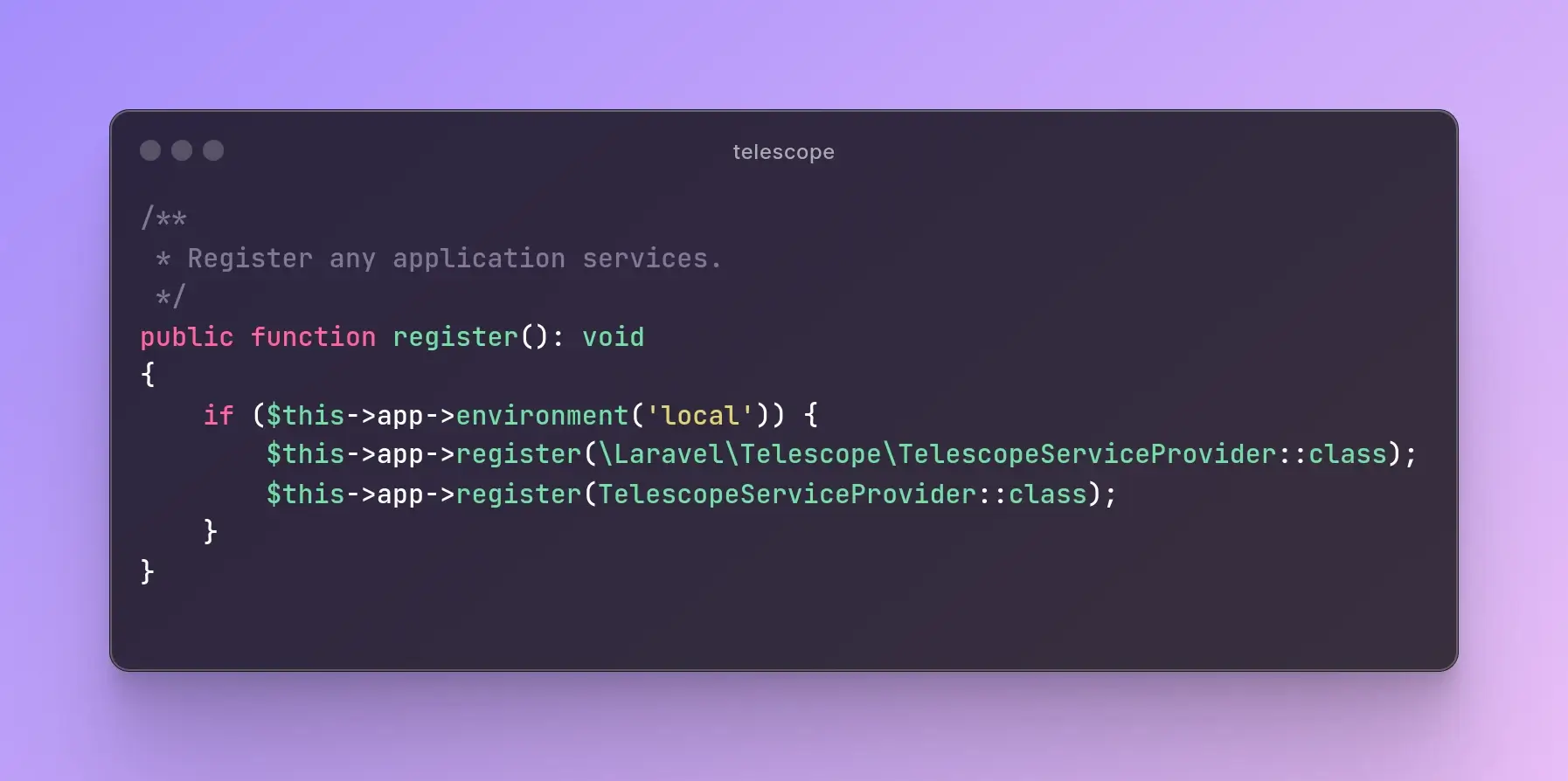
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
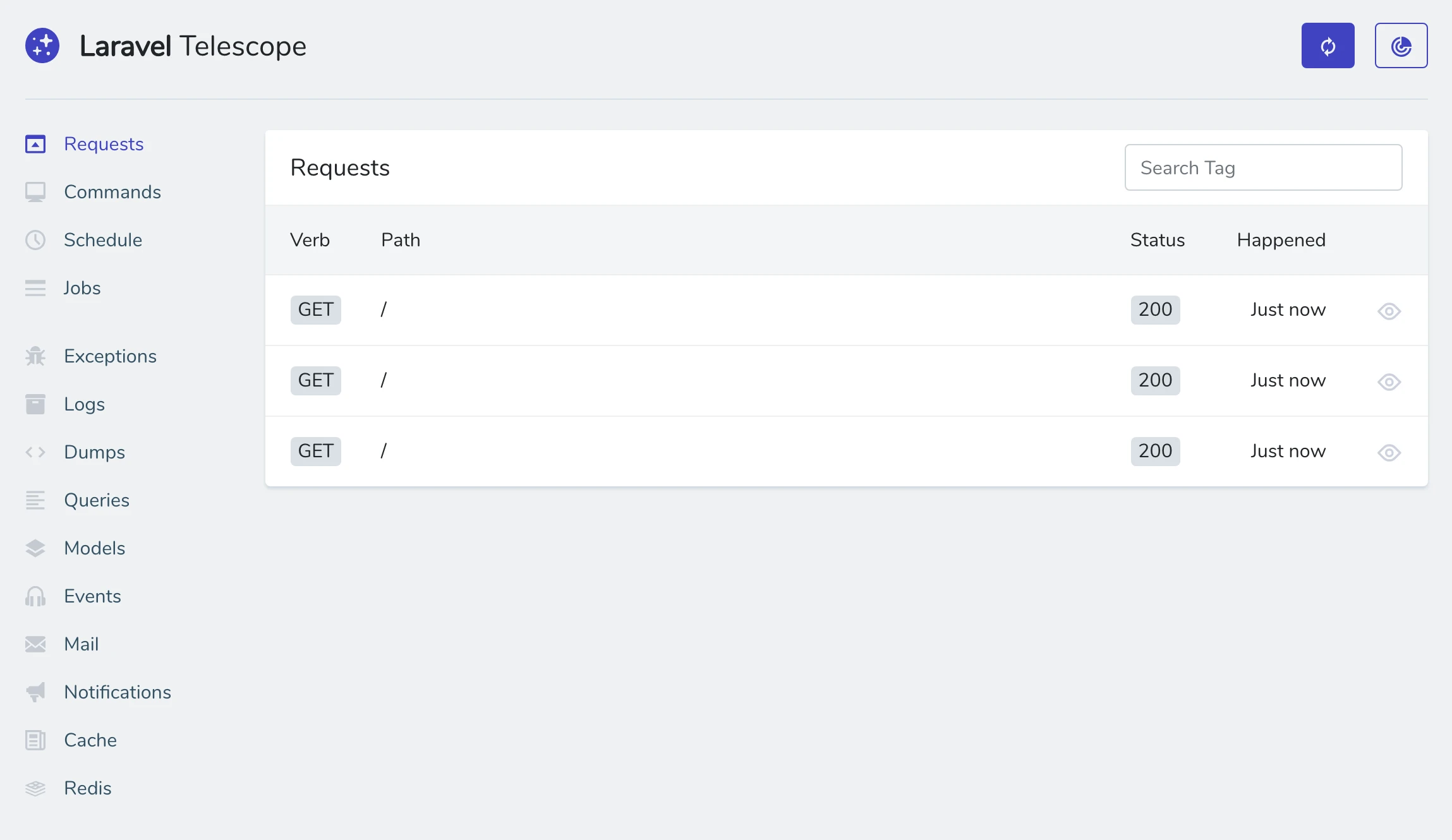
Laravel Telescope: Debugging Made Easy (Part - 1)
Sonu Singh
24 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles