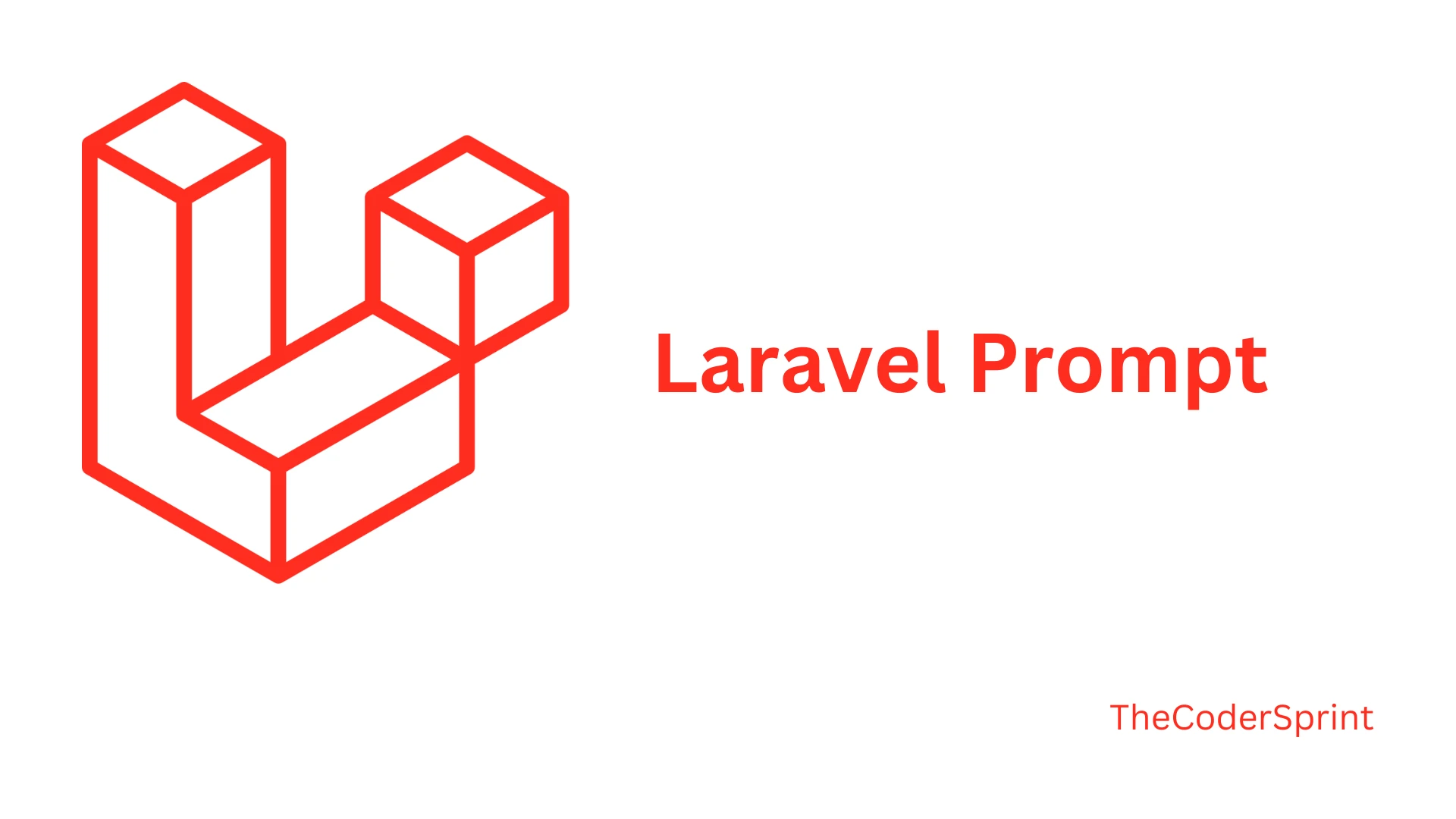
Enhancing CLI Interactivity in Laravel with Laravel Prompts
Are you tired of building boring command-line interfaces (CLIs) in your Laravel applications? Do you wish to add some spice and interactivity to your console commands? Look no further! In this article, we're going to explore how to enhance your CLI experience using Laravel Prompts, a package that brings interactive prompts to your Laravel applications effortlessly.
What are Laravel Prompts?
Laravel Prompts is a package that simplifies the process of adding interactive prompts to your Laravel Artisan commands. Whether you want to gather user input, display options for selection, or provide a more engaging experience for your CLI users, Laravel Prompts has got you covered.
Installation
Laravel Prompts is already included with the latest release of Laravel. Laravel Prompts may also be installed in your other PHP projects by using the Composer package manager:
composer require laravel/prompts
Part 1: Command Setup
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use function Laravel\Prompts\{text,select};
class Calculator extends Command
{
protected $signature = 'app:calculator';
protected $description = 'Perform basic arithmetic operations';
In this part, we define a new Artisan command called app:calculator
. It extends the Command class provided by Laravel. We also import two functions from Laravel Prompts: text()
and select()
.
Part 2: Command Handling
public function handle()
{
// Code for handling user input and performing arithmetic operations goes here
}
The handle()
method is where the main logic of our command resides. This method is automatically executed when the app:calculator
command is run. Inside this method, we'll gather user input and perform arithmetic operations.
Part 3: Gathering User Input
$a = text(
label: "Enter number 1",
required: true,
validate: ['value' => 'required|numeric']
);
$b = text(
label: "Enter number 2",
required: true,
validate: ['value' => 'required|numeric']
);
Here, in the handle method, we use the text()
function provided by Laravel Prompts to prompt the user to enter two numbers. We specify a label for each input field, making it clear what the user is expected to input. We also mark both inputs as required and validated.
Part 4: Selecting Arithmetic Operation
$type = select(
label: 'What action do you want to perform?',
options: ['Addition', 'Subtraction', 'Multiplication', 'Division'],
required: true
);
Using the select()
function, we present the user with a list of arithmetic operations to choose from. The user can select one of the options provided: Addition, Subtraction, Multiplication, or Division. This input is also marked as required.
Part 5: Performing Arithmetic Operations
// Perform the selected arithmetic operation
$result = match ($type) {
'Addition' => $a + $b,
'Subtraction' => $a - $b,
'Multiplication' => $a * $b,
'Division' => $b != 0 ? $a / $b : null, // Check for division by zero
default => null
};
Based on the user's choice of arithmetic operation ($type
), we perform the corresponding operation on the two numbers ($a
and $b
). We use a match expression to determine which operation to perform and calculate the result accordingly.
Part 6: Displaying the Result
// Display the result
$this->info("Result is " . $result);
Finally, we display the result of the arithmetic operation to the user. We use the info() method provided by Laravel to output the result in a user-friendly manner.
Let's put it all together now
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use function Laravel\Prompts\{text,select};
class Calculator extends Command
{
protected $signature = 'app:calculator';
protected $description = 'Perform basic arithmetic operations';
public function handle()
{
// Prompt the user to enter the first number
$a = text(
label: "Enter number 1",
required: true,
validate: ['value' => 'required|numeric']
);
// Prompt the user to enter the second number
$b = text(
label: "Enter number 2",
required: true,
validate: ['value' => 'required|numeric']
);
// Ask the user to select an arithmetic operation
$type = select(
label: 'What action do you want to perform?',
options: ['Addition', 'Subtraction', 'Multiplication', 'Division'],
required: true
);
// Perform the selected arithmetic operation
$result = match ($type) {
'Addition' => $a + $b,
'Subtraction' => $a - $b,
'Multiplication' => $a * $b,
'Division' => $b != 0 ? $a / $b : null, // Check for division by zero
default => null
};
// Display the result
$this->info("Result is " . $result);
}
}
Running the Command
To run our app:calculator
command, simply execute the following Artisan command in your terminal:
php artisan app:calculator
or if you are using sail then Run:
./vendor/bin/sail artisan app:calculator
You'll be prompted to enter two numbers and choose an arithmetic operation. Once you provide the input, the command will display the result of the operation as below.
Conclusion
With Laravel Prompts, adding interactive prompts to your CLI commands becomes a breeze. Whether you're building a simple calculator like we did in this article or creating more complex interactive commands, Laravel Prompts empowers you to provide a richer experience for your users.
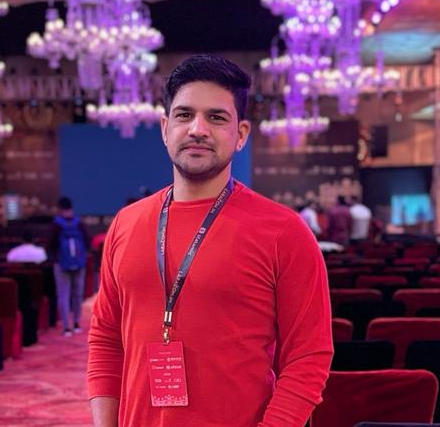
Author: Sonu Singh
With over 7 years of dedicated experience in web development, I've traversed through the dynamic landscape of digital technology, honing my skills and expertise across various frameworks and content management systems. My journey began with the exploration of frameworks like CodeIgniter, CakePHP, Yii, and eventually, I found my passion in Laravel - a framework that resonated deeply with my love for elegant, efficient code. Throughout my career, I've had the privilege to contribute to numerous projects, ranging from small-scale ventures to large, enterprise-level solutions. Each project has been a unique opportunity to push boundaries, solve intricate challenges, and deliver innovative solutions tailored to meet client needs. One of my proudest accomplishments includes the development of a SaaS-based project using Laravel and MySQL. This venture not only showcased my technical prowess but also demonstrated my ability to conceptualize, design, and execute scalable solutions that drive business growth. In addition to my proficiency in development, I've also delved into server deployment and query optimization, ensuring that every aspect of a project - from its codebase to its infrastructure - is optimized for peak performance and reliability.
Recent Posts
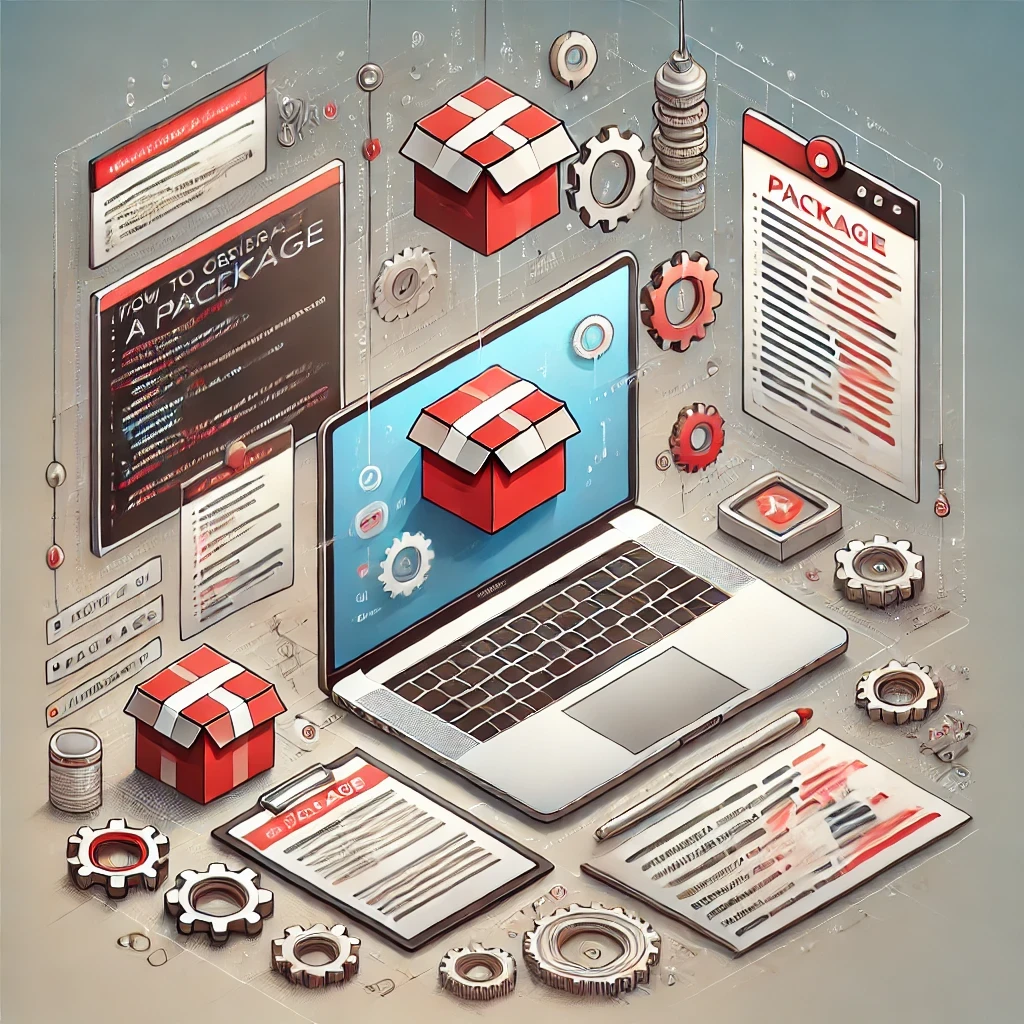
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
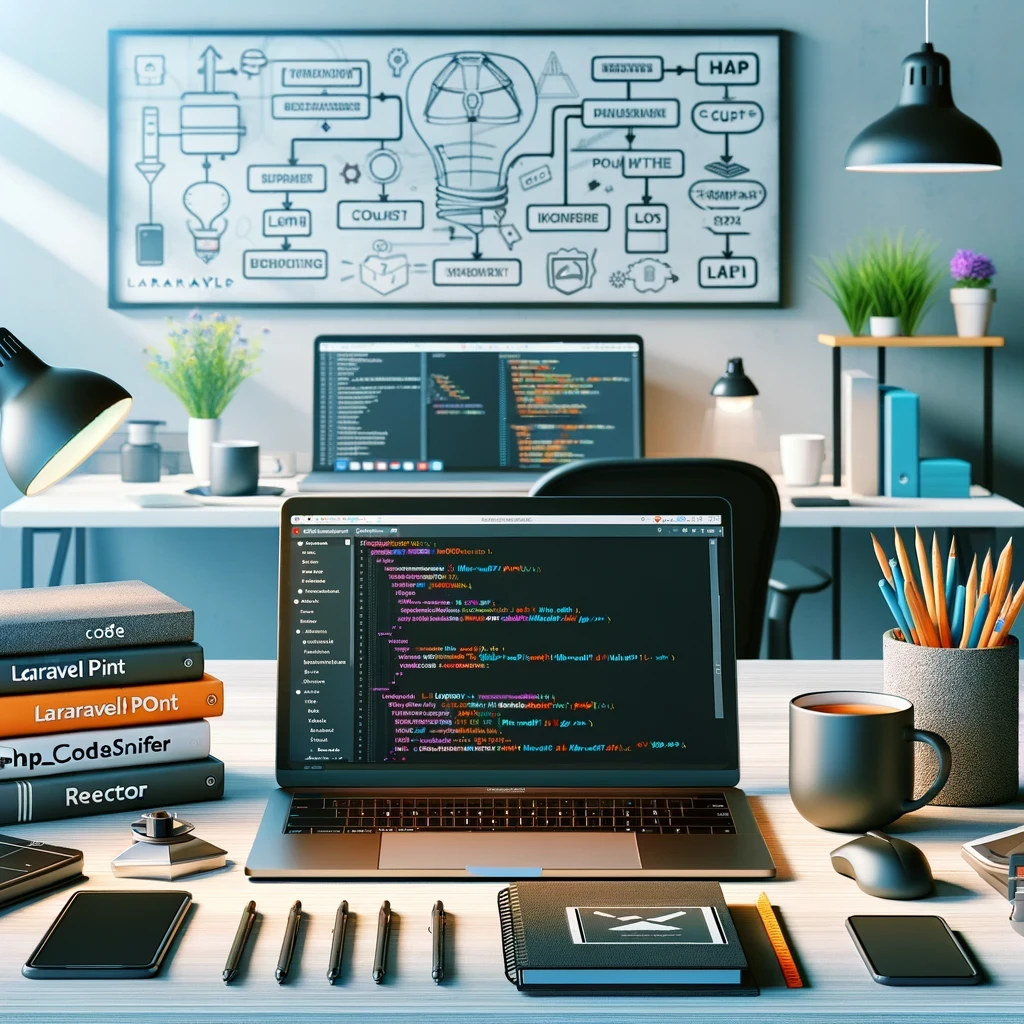
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
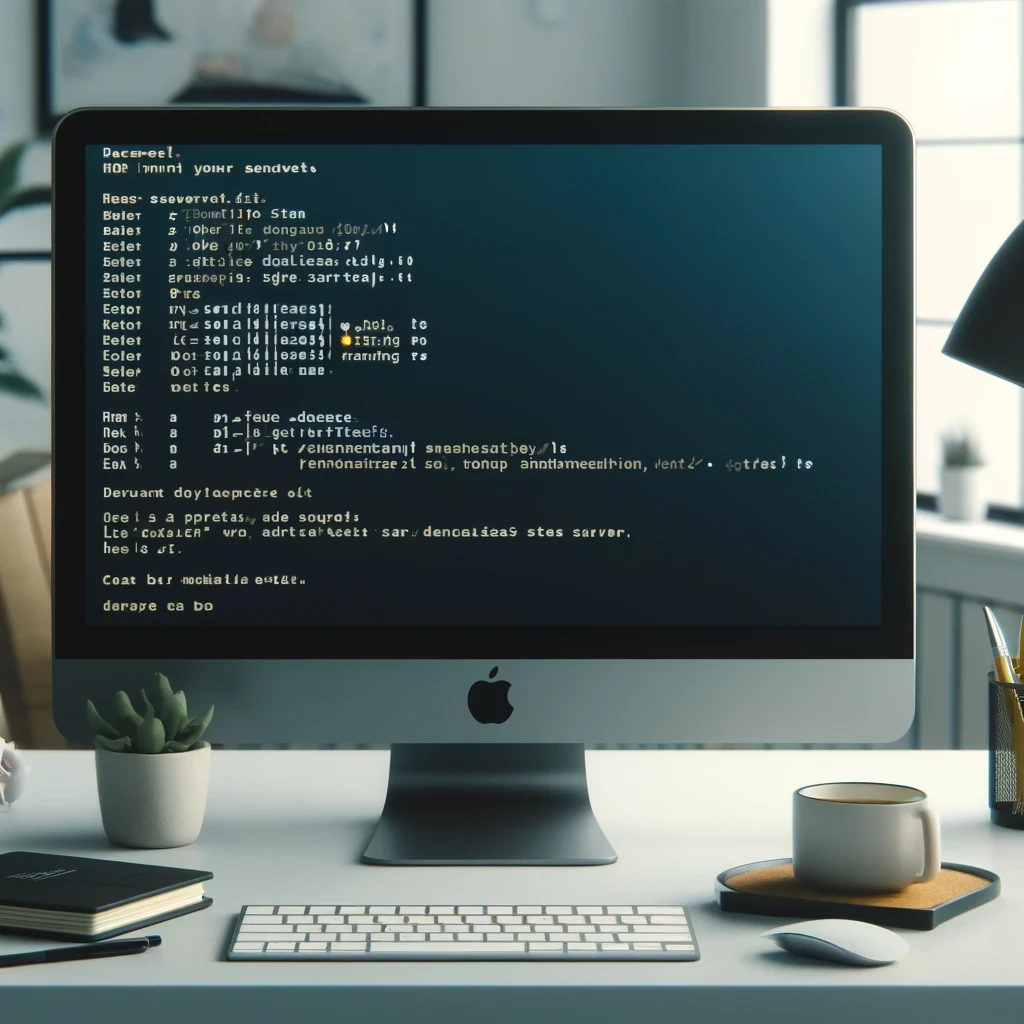
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
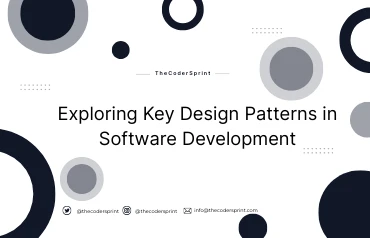
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
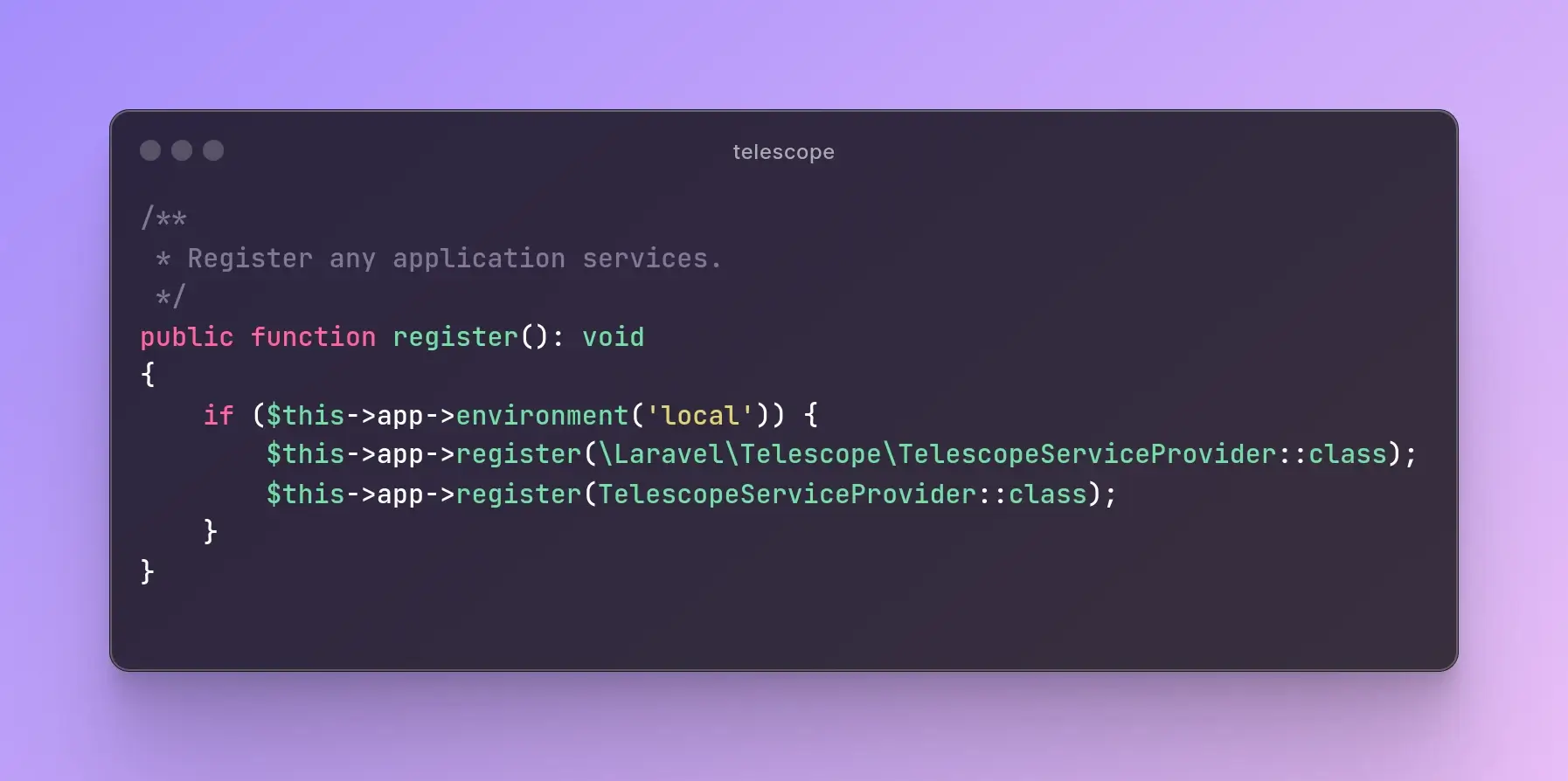
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles