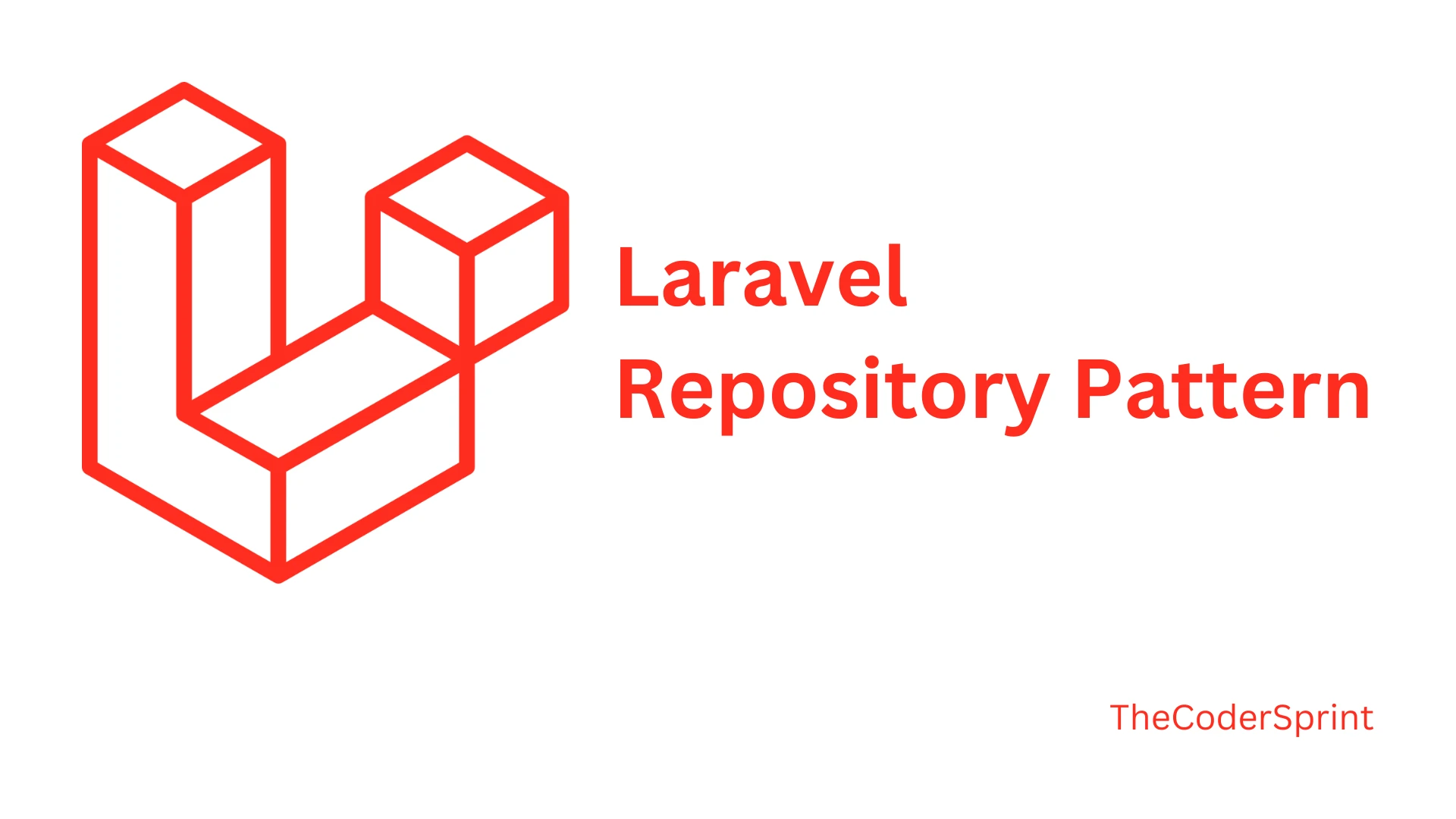
Introduction to the Repository Pattern in Laravel
Design patterns are like templates or proven ways to solve common problems in software development. They help developers organize their code in a way that makes it easier to manage, update, and understand.
Understanding the Repository Pattern in Laravel
Think of the Repository Pattern as a middleman or an intermediary in your Laravel application. Its job is to manage the conversations between the two parts of your app: one part that asks for data (like your web pages) and the other part that knows how to get that data (like your database).
How It Works
Normally, when your application needs data, it asks the database directly. This is fine for small projects, but as your app grows, this direct chatting can get messy. Imagine every time you need something, you have to go through a huge, complex warehouse to find it. What if the warehouse changes how things are organized? You'd have to relearn where everything is, right?
The Repository Pattern introduces a simpler way. Instead of going directly to the database, your application talks to a repository. The repository knows how to talk to the database.
Benefits of Using the Repository Pattern
Easier Code Maintenance: When your code for accessing data from the database is in one place (the repository), it's much easier to maintain. Imagine if you have to change something about how you access data. Instead of searching through your entire codebase for every place you've queried the database, you just make the change in one spot. It's like having a single remote control for all your electronic devices at home. You don't need to learn how to operate different remotes; you just use the one.
Keeping Code Clean by Separating Concerns: In programming, "separating concerns" means organizing your code so that each part deals with a specific job. The Repository Pattern helps by taking the responsibility of fetching data away from the models (which represent your data) and controllers (which handle user inputs). This separation makes your code cleaner and easier to read, like organizing your clothes into drawers. Each drawer holds a specific type of clothing, so it's easier to find what you're looking for.
Making Your Code Easier to Test: Testing is a way to check if your code works as expected. With the Repository Pattern, you can test how your application handles data without actually querying the database. You can use a fake repository that mimics the real one. This approach is faster and ensures your tests aren't affected by your database's state. It's like rehearsing a play with stand-ins before the actual actors go on stage.
Reducing Duplicate Code: When you find yourself using the same code in multiple places, it's usually a good idea to move it to a single location. This practice is known as the DRY principle (Don't Repeat Yourself). The Repository Pattern encourages this by allowing you to have common data access logic in one place. If multiple parts of your application need to perform the same query, they can do so through the repository. Think of it as buying in bulk. Instead of everyone in the family buying their own pack of toilet paper, you buy a large pack that everyone uses, reducing the cost and storage space needed.
Understanding Code Structure Without the Repository Pattern
Before we dive into the implementation of the Repository Pattern, let's take a moment to understand the typical structure of Laravel applications without using this pattern. Often, you might come across Laravel code that directly interacts with the model within the controller. Here's a common example:
class UsersController extends Controller
{
public function index()
{
$users = User::all();
return view('users.index', ['users' => $users]);
}
}
This approach, while straightforward, tightly couples your controller to the model, making it directly dependent on the database layer. At first glance, it seems efficient for small projects or rapid prototyping. However, imagine a scenario where the client decides to change the underlying data storage - perhaps moving from MySQL to a database not supported by Eloquent, Laravel's default ORM. Adapting to such a change becomes cumbersome and risky because you'd need to sift through the entire application to update data access logic, increasing the chance of errors.
Moreover, this method contradicts the principles of good software design, which advocate for separation of concerns and modularity. By intertwining data access logic with controller logic, we hinder the application's testability and flexibility.
The Power of Interfaces in Laravel
A more resilient approach involves using interfaces to abstract the interaction between controllers and the model layer. Consider this improved version of our UsersController:
class UsersController extends Controller
{
private $userRepository;
public function __construct(UserRepositoryInterface $userRepository)
{
$this->userRepository = $userRepository;
}
public function index()
{
$users = $this->userRepository->all();
return view('users.index', ['users' => $users]);
}
}
By injecting a UserRepositoryInterface into the controller, we decouple it from the specific data access mechanism. This abstraction layer allows us to change the underlying implementation of the repository without altering the controller's code. Should the need arise to switch data storage solutions, we simply develop a new repository that implements the interface, seamlessly integrating the new data source with minimal impact on the broader application.
Implementing PHP Repository Pattern in Laravel
Setting Up Your Environment
First things first, you need to have Laravel installed. If you haven't done this yet, go to the Laravel website and follow the installation instructions for your operating system. Think of this as setting up your workspace before starting a project. You need your tools and materials organized and ready to go.
Step 1: Creating the Repository Structure
Create a Repository Folder: Inside your Laravel app's app folder, create a new folder named Repository. This is where all your repositories will live. It's like dedicating a section of your workspace to a specific type of tool. You know exactly where to go when you need something.
Create Subfolders for Your Repositories: If you're planning to use Eloquent (Laravel's default ORM), you might create an Eloquent subfolder. This helps keep your repository implementations organized.
Step 2: Defining Interfaces
Interfaces are contracts that ensure your repositories will always have a certain set of methods.
Create an Interface for Each Repository: For example, if you have a User model, create a UserRepositoryInterface.php file in the Repository folder. This interface might declare methods like all(), find($id), and create(array $attributes).
Step 3: Implementing the Base and Model-Specific Repositories
BaseRepository: This is a general repository class that can be extended by your model-specific repositories. It should implement the common operations every model needs, like finding a model by its ID or creating a new model.
namespace App\Repository\Eloquent;
use App\Repository\EloquentRepositoryInterface;
use Illuminate\Database\Eloquent\Model;
class BaseRepository implements EloquentRepositoryInterface {
protected $model;
public function __construct(Model $model) {
$this->model = $model;
}
public function create(array $attributes): Model {
return $this->model->create($attributes);
}
public function find($id): ?Model {
return $this->model->find($id);
}
}
Model-Specific Repository: For example, a UserRepository that extends BaseRepository and implements UserRepositoryInterface.
namespace App\Repository\Eloquent;
use App\Model\User;
use App\Repository\UserRepositoryInterface;
use Illuminate\Support\Collection;
class UserRepository extends BaseRepository implements UserRepositoryInterface {
public function __construct(User $model) {
parent::__construct($model);
}
public function all(): Collection {
return $this->model->all();
}
}
Step 4: Setting Up the Service Provider
Laravel uses service providers to bind interfaces to their implementations. Create a RepositoryServiceProvider.php file and register your bindings there.
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use App\Repository\UserRepositoryInterface;
use App\Repository\Eloquent\UserRepository;
class RepositoryServiceProvider extends ServiceProvider {
public function register() {
$this->app->bind(UserRepositoryInterface::class, UserRepository::class);
}
}
Don't forget to register your new service provider in config/app.php under the providers array.
Step 5: Using Your Repository
Now, you can inject your repository interface into controllers, and Laravel will automatically resolve it to your implementation. Here's an example using UserRepositoryInterface:
namespace App\Http\Controllers;
use App\Repository\UserRepositoryInterface;
class UsersController extends Controller {
private $userRepository;
public function __construct(UserRepositoryInterface $userRepository) {
$this->userRepository = $userRepository;
}
public function index() {
$users = $this->userRepository->all();
return view('users.index', ['users' => $users]);
}
}
When Not to Use the Repository Pattern
Small or Simple Projects:
For small applications or projects with limited complexity, introducing the Repository Pattern can add unnecessary layers and abstraction. If your project consists mainly of CRUD operations and doesn't anticipate significant growth or complexity, sticking to Laravel's Eloquent ORM directly in your controllers or services may be more straightforward and efficient. It's like using a sledgehammer to crack a nut—overkill for the task at hand.
Rapid Prototyping
When the goal is to quickly validate an idea or feature, the speed of development is often more critical than architectural purity. In such cases, directly using models in controllers can expedite development and feedback cycles. Once the prototype has proven its value, you can then consider refactoring towards more scalable patterns like the Repository Pattern if necessary.
Projects with Limited Data Access Variation
If your application interacts with a single type of database and doesn't require abstracting the data layer (for example, switching between SQL and NoSQL databases based on environment or configuration), implementing the Repository Pattern might not provide substantial benefits. In applications where data access is straightforward and unlikely to change, the added abstraction can introduce unnecessary complexity.
Learning Curve and Development Overhead
Implementing the Repository Pattern requires a solid understanding of design patterns, interface abstraction, and dependency injection. For teams new to these concepts or working under tight deadlines, the learning curve and additional development overhead can be a deterrent. It's important to weigh the benefits of implementing such a pattern against the time and resources available for learning and development.
Considerations Before Deciding
Project Scope and Scale: Assess the complexity and future needs of your project. The Repository Pattern shines in applications that demand high scalability, testability, and flexibility in data access mechanisms.
Team Expertise: Consider the team's familiarity with design patterns and Laravel's advanced features. A steep learning curve can impact development timelines.
Performance Implications: While generally minimal, the extra layer of abstraction can have performance implications, especially in highly optimized applications where every millisecond counts.
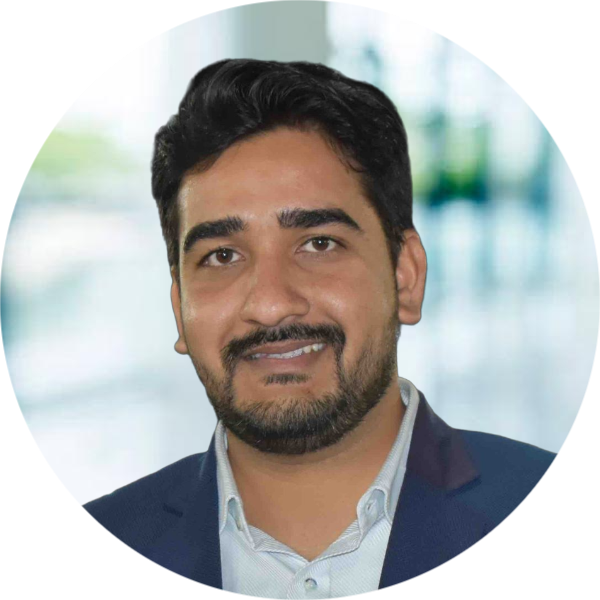
Author: Manish Kumar
I've been working with PHP for over 11 years and focusing on Laravel for the last 6 years. During this time, I've taken part in big projects and gotten to know tools like Laravel Livewire really well. Besides working on projects, I enjoy writing about Laravel on LinkedIn and Medium to share what I've learned. I'm always looking to learn more and share my knowledge with others. Right now, I'm diving into new areas like using Docker to make web apps run better and exploring how to build them using microservices. My goal is to keep learning and help others do the same in the world of web development.
Recent Posts
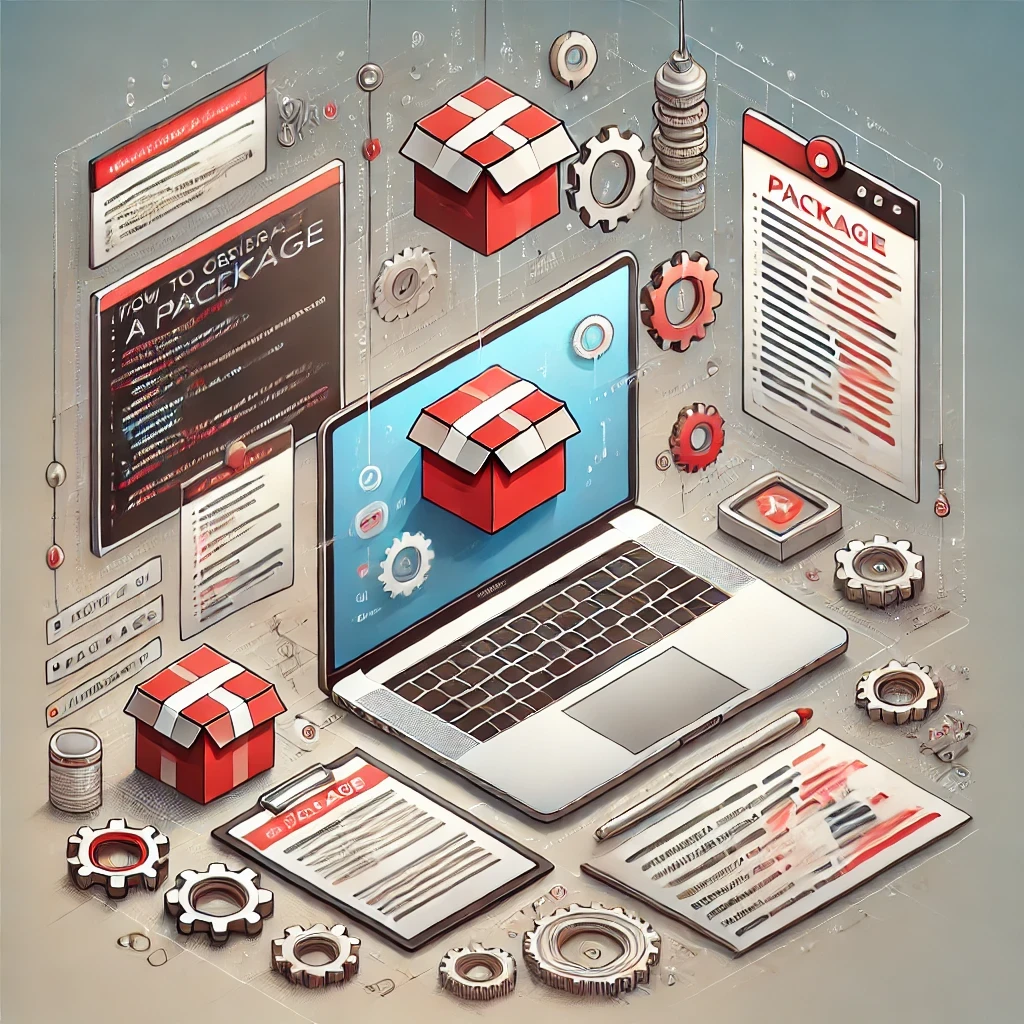
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
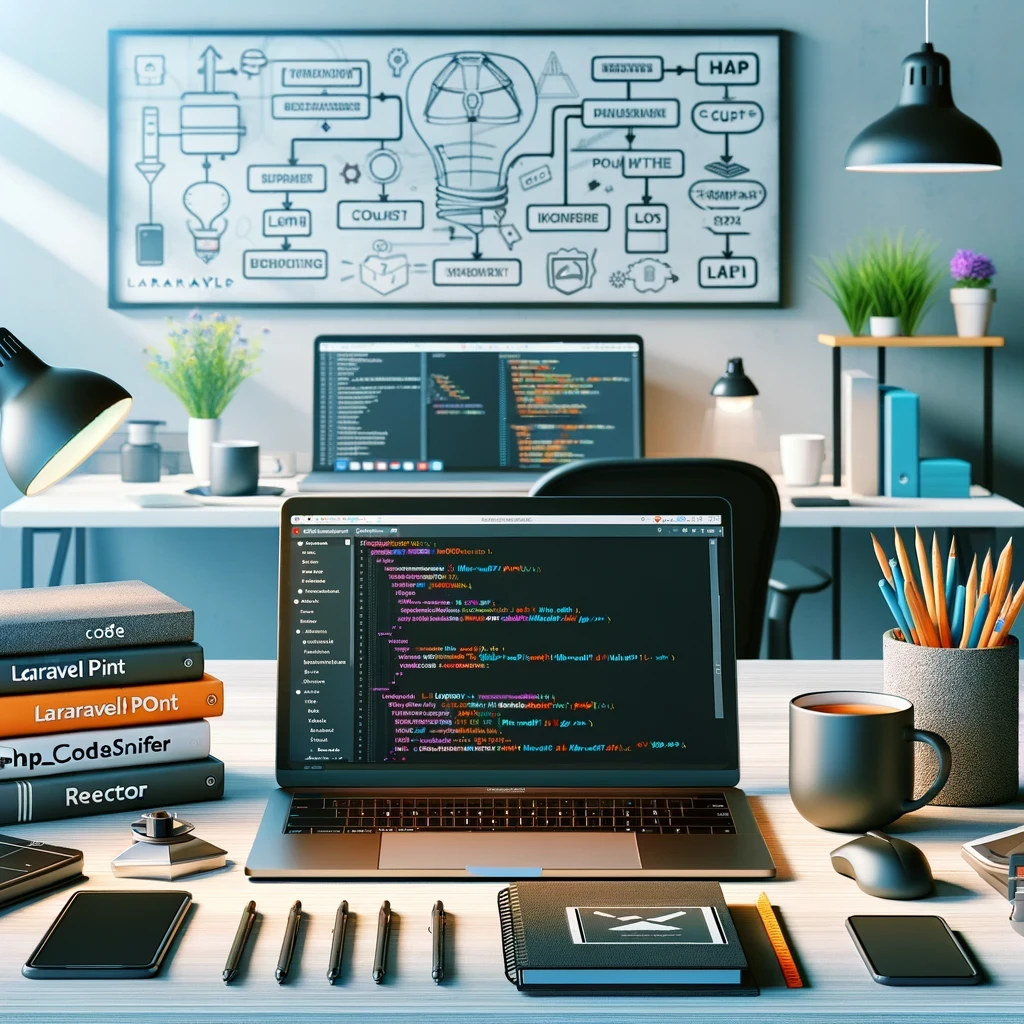
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
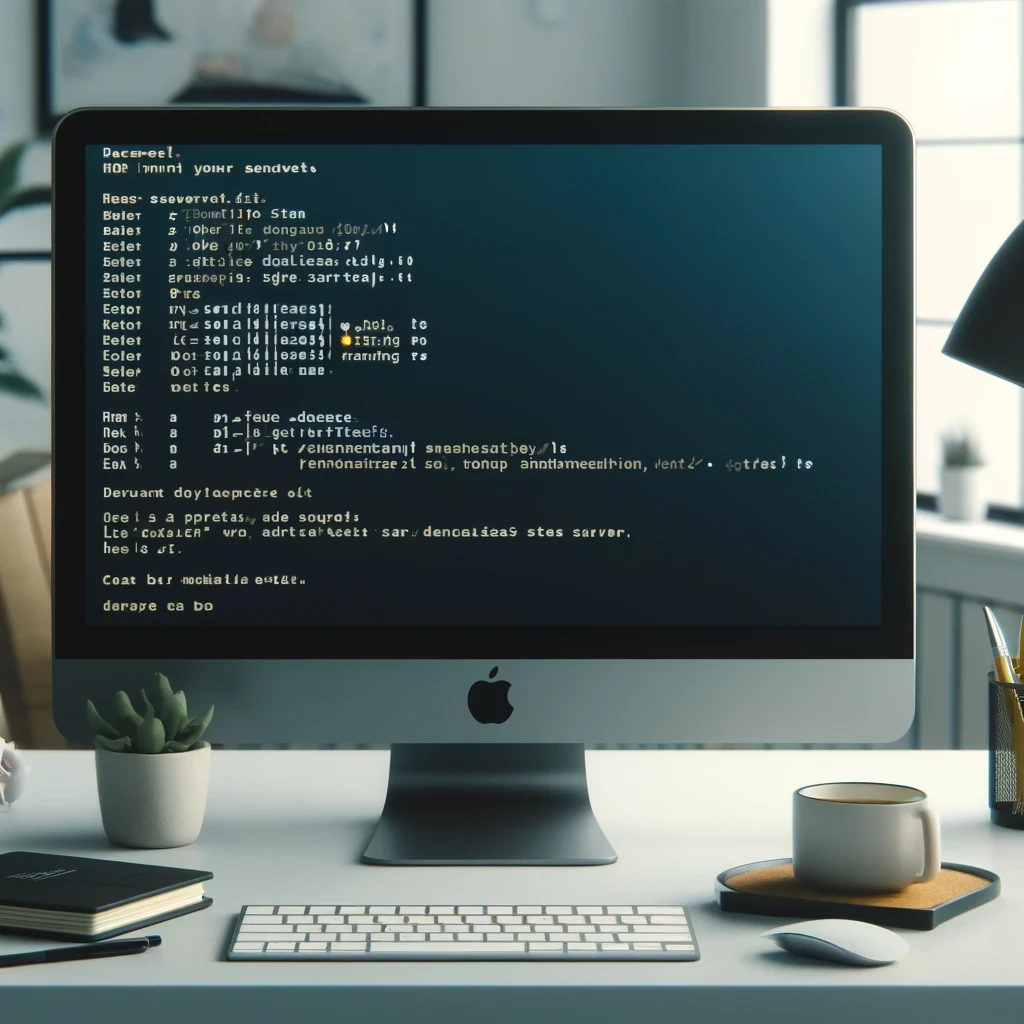
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
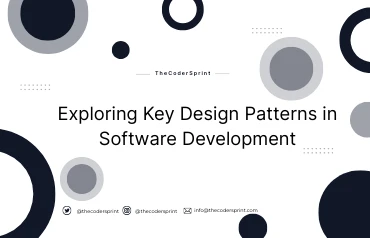
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
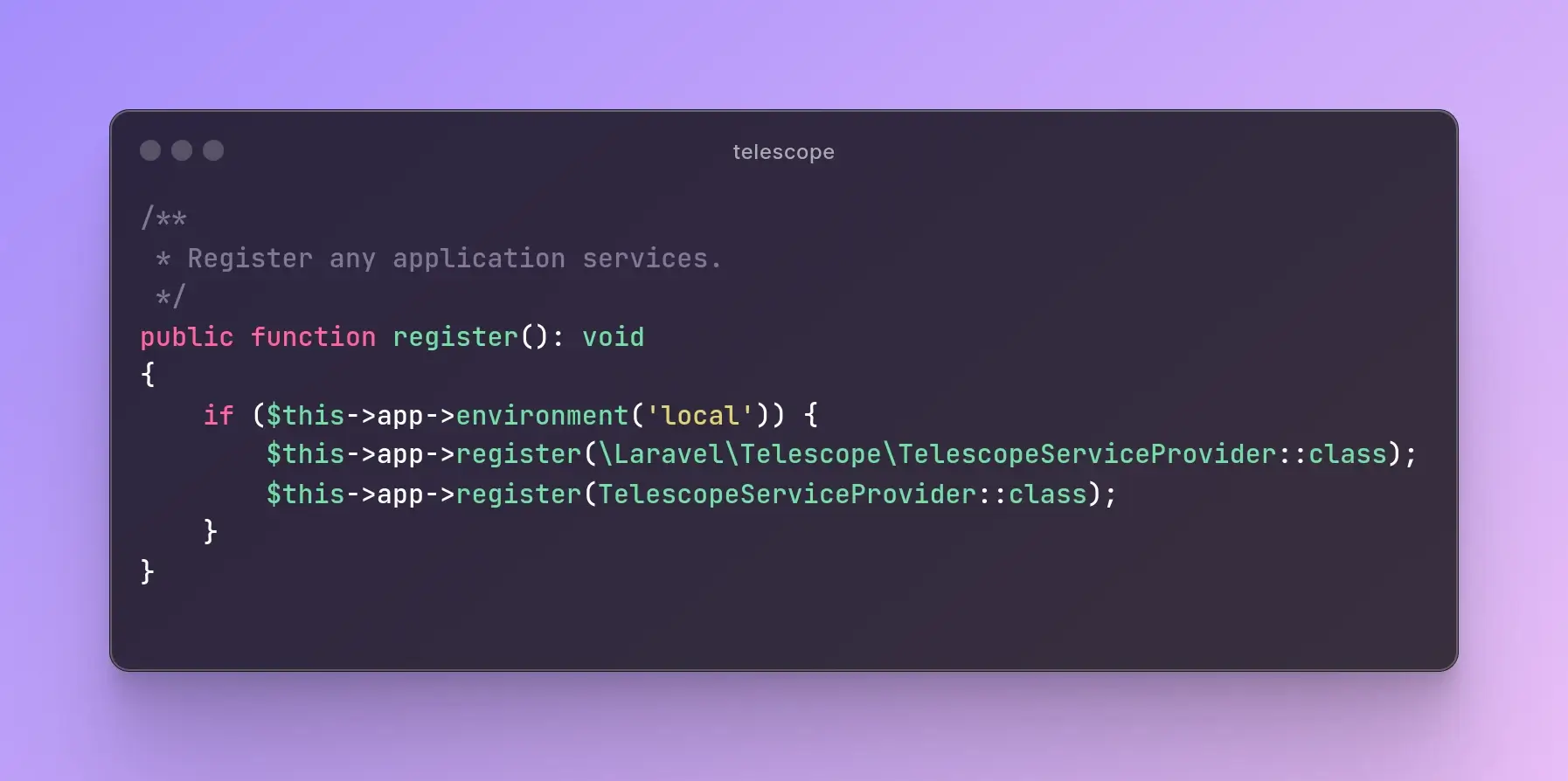
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles