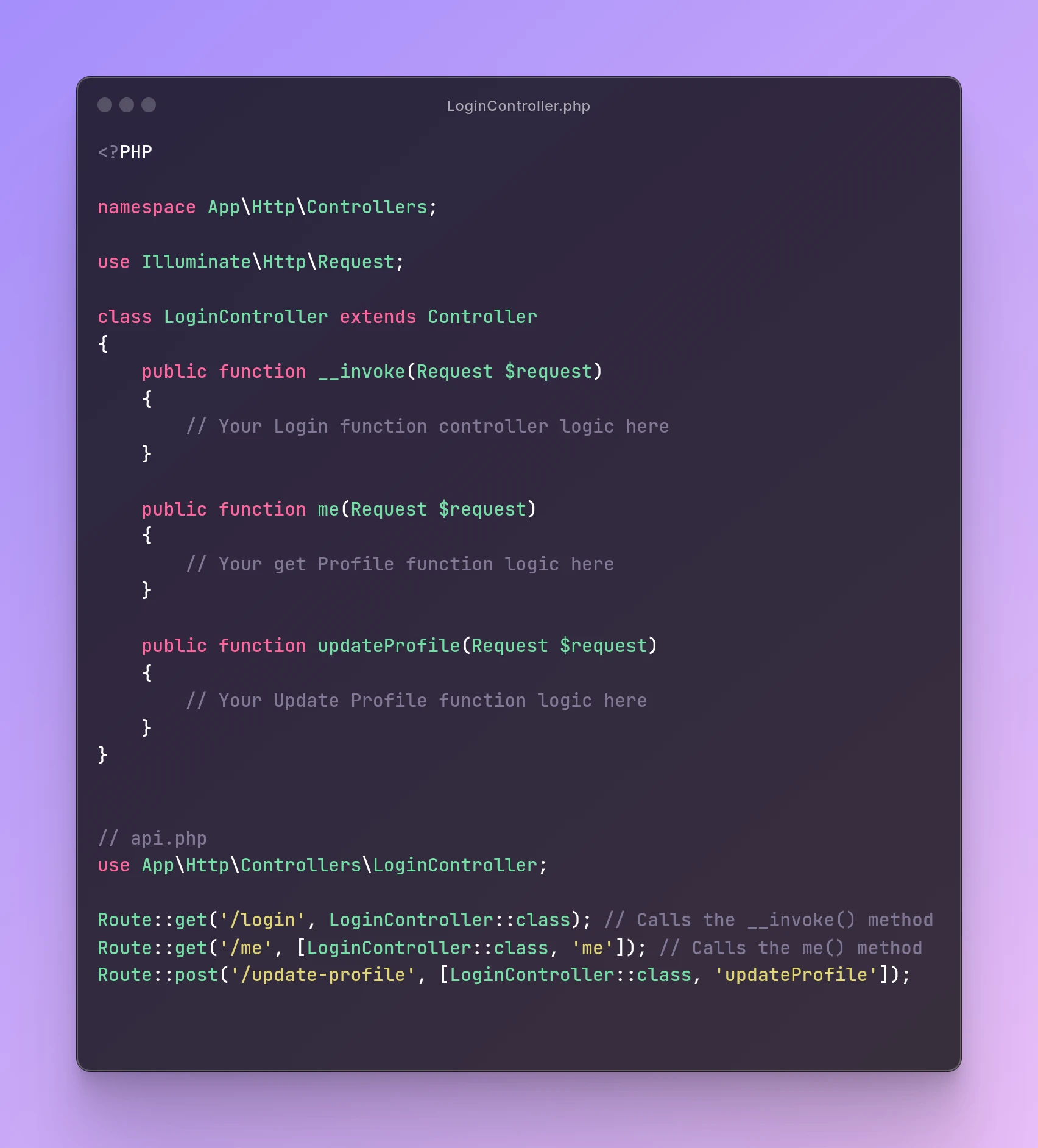
Invocable Controllers in Laravel
Are you ready to take your Laravel skills to the next level? Let's talk about invocable controllers! They're like magic tools that simplify building APIs and handling data in your web apps. Let's explore how they work and how they can make your coding life easier!
What are Invocable
Controller?
In Laravel, an invocable controller is a controller class that implements the __invoke()
magic method. When a class implements __invoke()
, it allows you to treat an object of that class as if it were a function.
Here's how it works in the context of Laravel controllers:
-
Controller Class: You create a controller class like you normally would in Laravel, but instead of defining methods like
index()
,show()
,store()
, etc., you implement the__invoke()
method. -
Routing: You can then route requests directly to the controller without specifying a method. Laravel automatically calls the
__invoke()
method when a request matches the route associated with the controller.
For example, let's say you have an invocable controller called LoginController
:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class LoginController extends Controller
{
public function __invoke(Request $request)
{
// Your controller logic here
}
}
And in your routes file (web.php
or api.php
), you can define a route to this invocable controller like so:
use App\Http\Controllers\LoginController;
Route::get('/login', LoginController::class);
In this example, when a GET request is made to /invoke
, Laravel will automatically invoke the __invoke()
method of LoginController
.
Using invocable controllers can be useful when you have simple controllers that only need one action, as it reduces boilerplate code and keeps your codebase clean and concise.
Additional Function in Invocable Controller
If you want to add additional functions to an invocable controller in Laravel, you can simply define them as regular methods within the controller class. The __invoke()
method will still be invoked when the controller is called directly, but you can also define other methods that can be accessed through routing.
Here's an example:
<?PHP
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class LoginController extends Controller
{
public function __invoke(Request $request)
{
// Your Login function controller logic here
}
public function me(Request $request)
{
// Your get Profile function logic here
}
public function updateProfile(Request $request)
{
// Your Update Profile function logic here
}
}
In your routes file, you can define routes to both the __invoke()
method and the me()
method:
use App\Http\Controllers\LoginController;
Route::get('/login', LoginController::class); // Calls the __invoke() method
Route::get('/me', [LoginController::class, 'me']); // Calls the me() method
Route::post('/update-profile', [LoginController::class, 'updateProfile']); // Calls the updateProfile() method
Conclusion
Overall, invocable controllers can be important in Laravel for simplifying code structure, improving readability, and enhancing developer productivity, especially in applications with a large number of controllers and routes. They are particularly useful for implementing RESTful APIs or handling CRUD operations where each controller action corresponds to a specific HTTP method on a resource.
- Tags:
- #Laravel
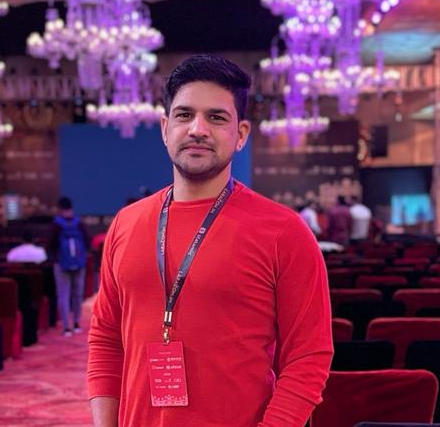
Author: Sonu Singh
With over 7 years of dedicated experience in web development, I've traversed through the dynamic landscape of digital technology, honing my skills and expertise across various frameworks and content management systems. My journey began with the exploration of frameworks like CodeIgniter, CakePHP, Yii, and eventually, I found my passion in Laravel - a framework that resonated deeply with my love for elegant, efficient code. Throughout my career, I've had the privilege to contribute to numerous projects, ranging from small-scale ventures to large, enterprise-level solutions. Each project has been a unique opportunity to push boundaries, solve intricate challenges, and deliver innovative solutions tailored to meet client needs. One of my proudest accomplishments includes the development of a SaaS-based project using Laravel and MySQL. This venture not only showcased my technical prowess but also demonstrated my ability to conceptualize, design, and execute scalable solutions that drive business growth. In addition to my proficiency in development, I've also delved into server deployment and query optimization, ensuring that every aspect of a project - from its codebase to its infrastructure - is optimized for peak performance and reliability.
Recent Posts
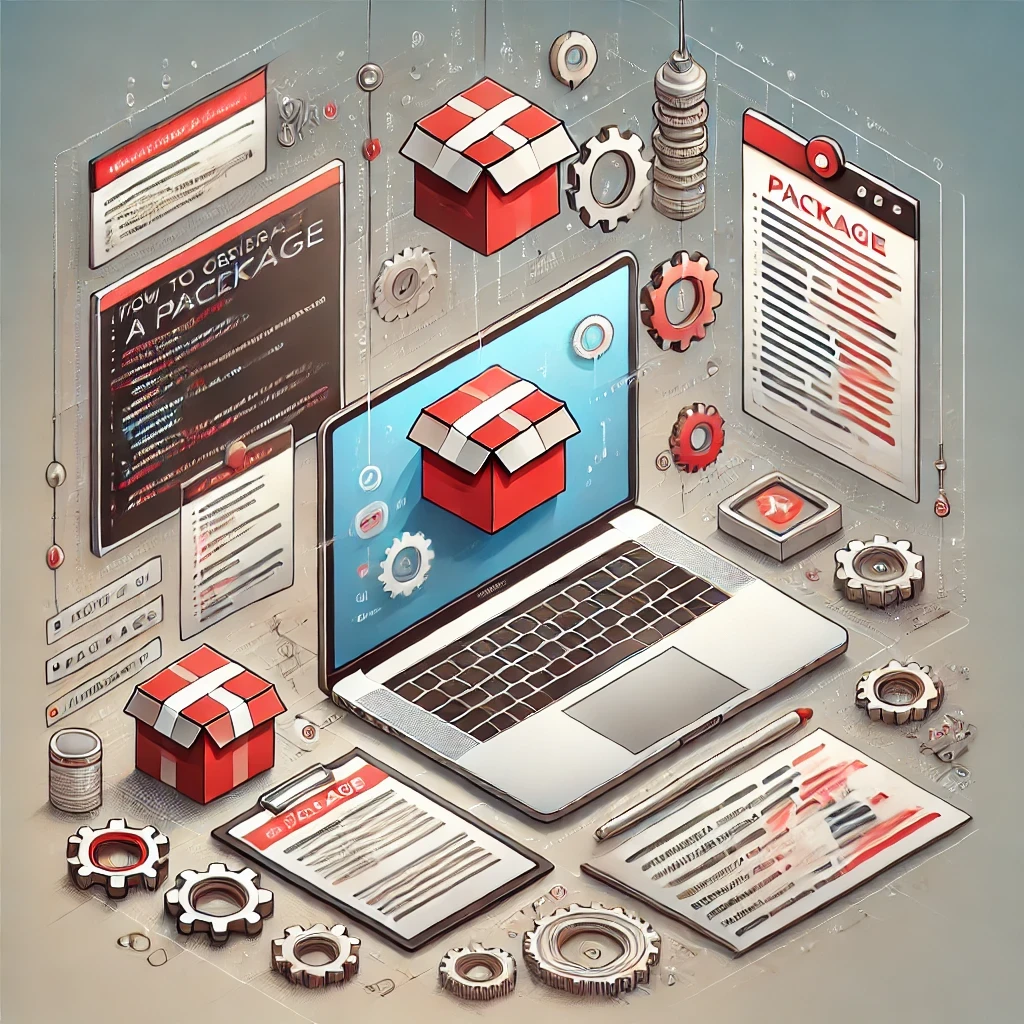
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
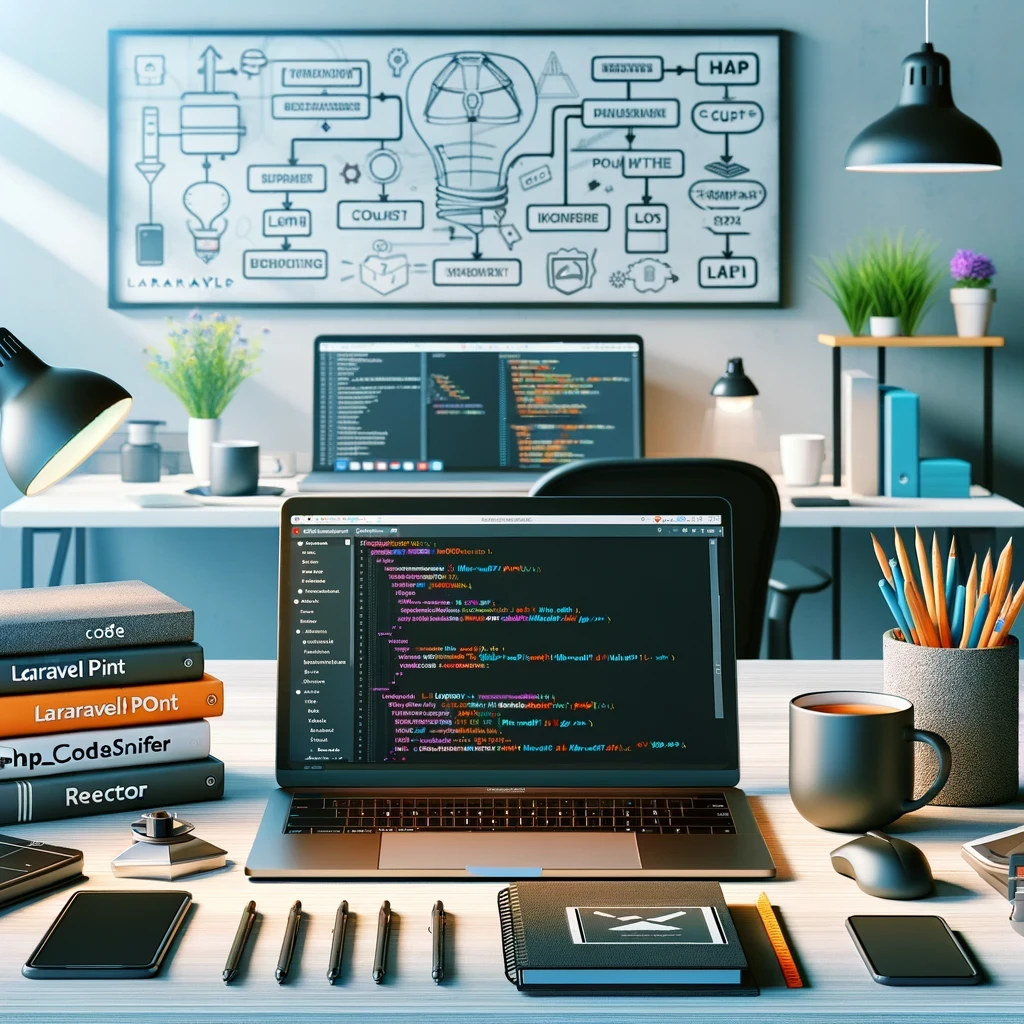
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
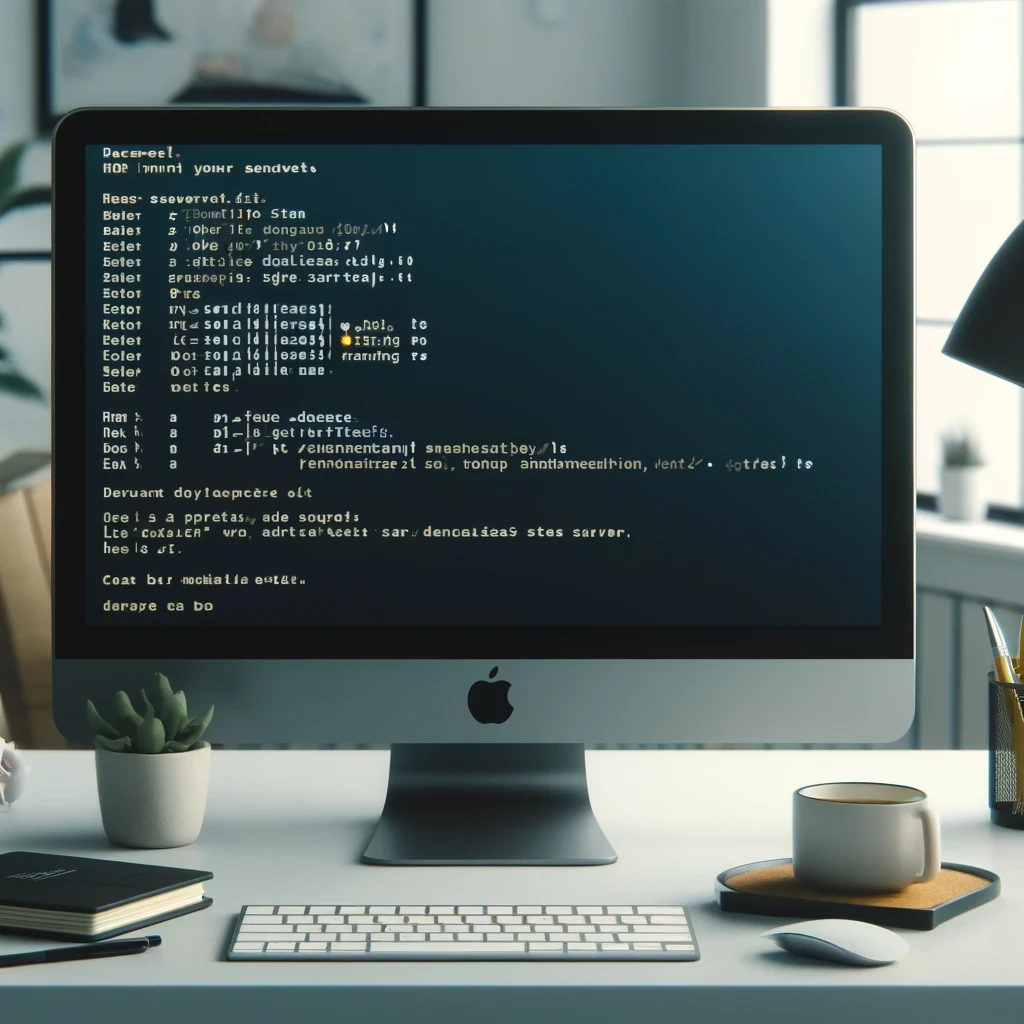
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
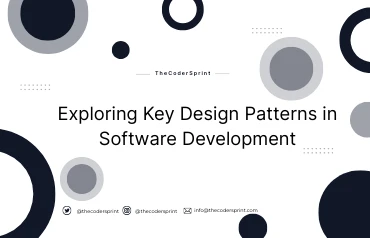
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
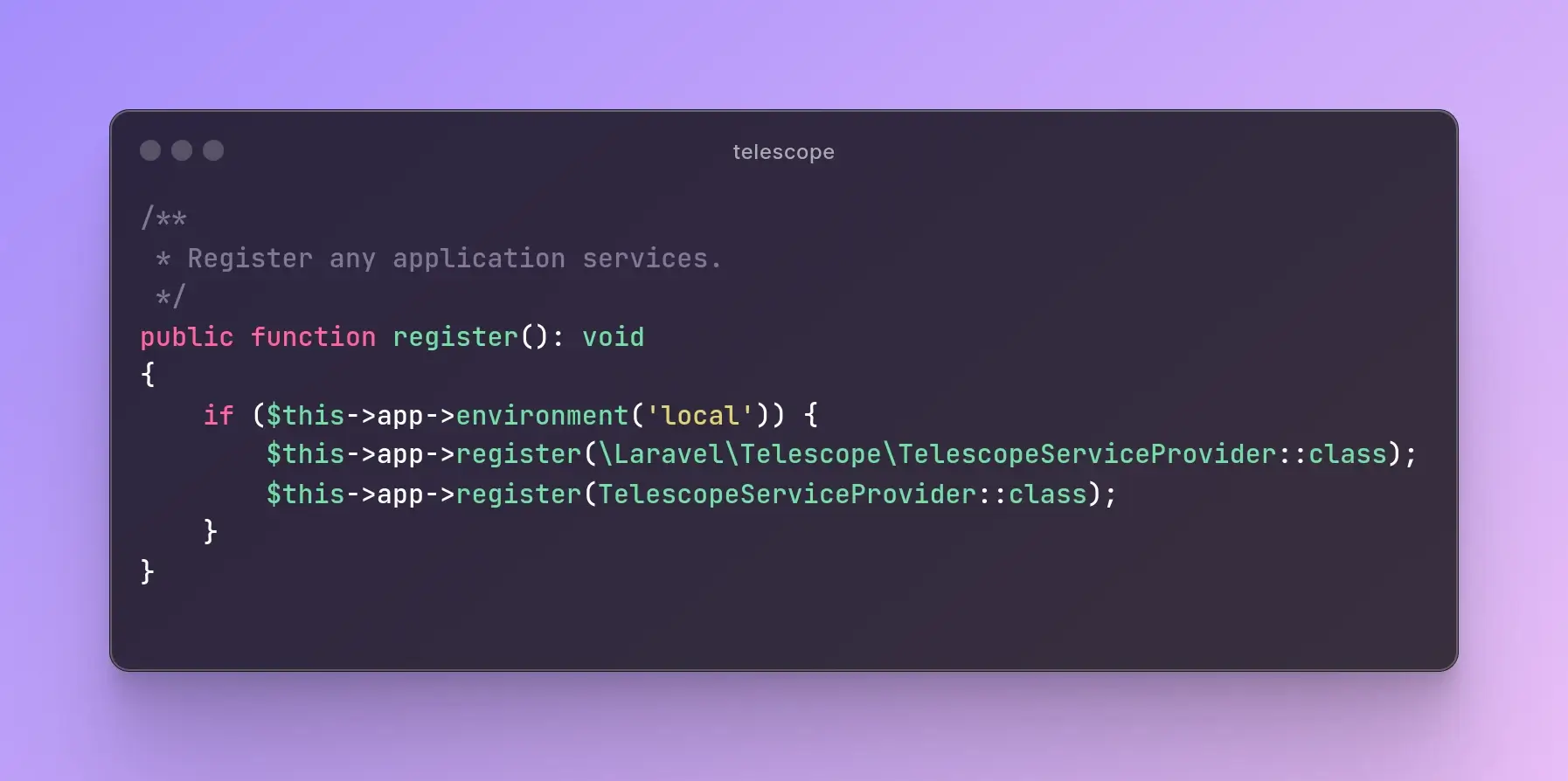
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles