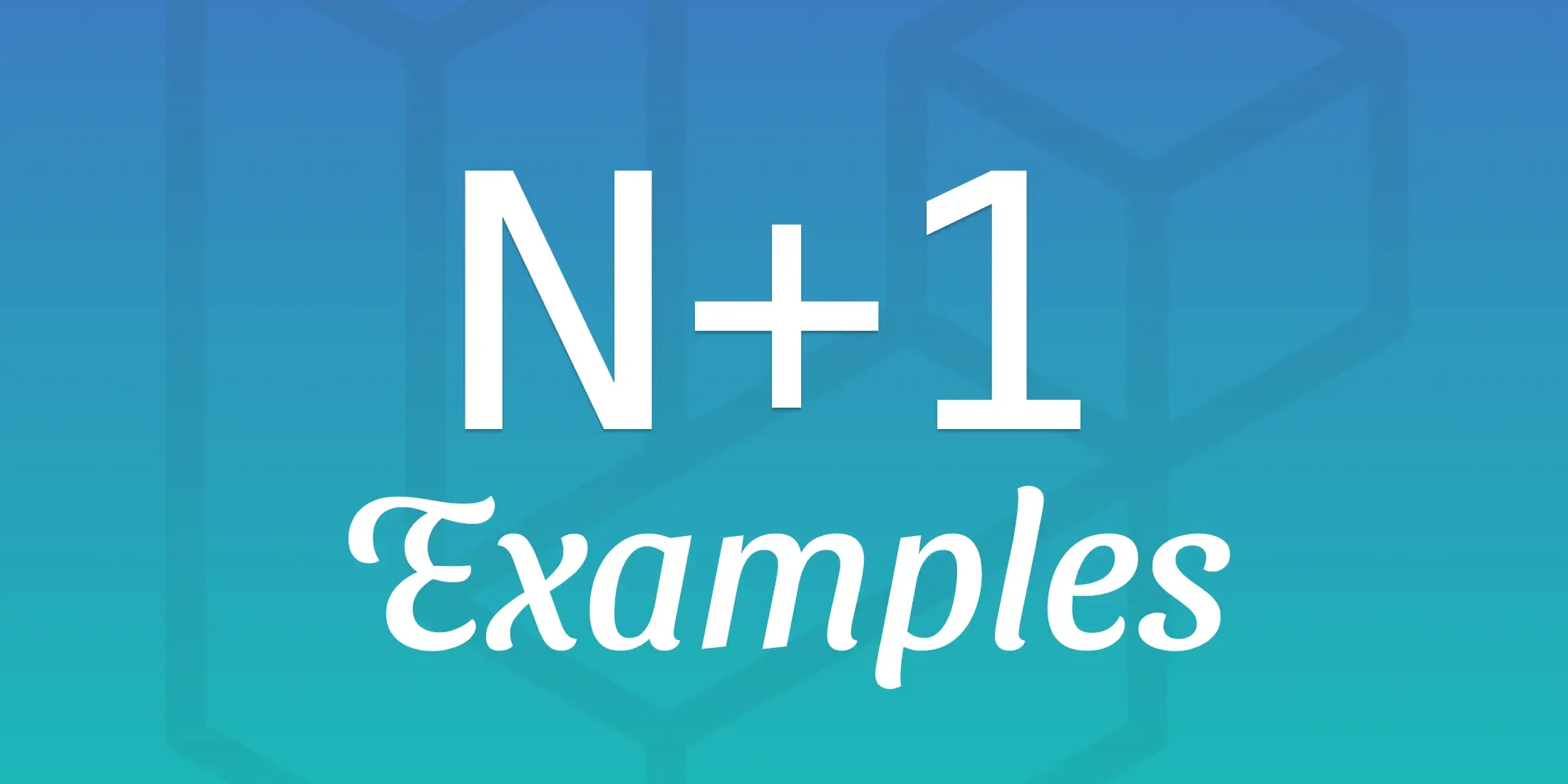
N+1 Queries In Laravel
N+1 queries are a frequent problem in Laravel that make your app slower because of unnecessary database calls. Imagine you’re at a grocery store with a shopping list. Instead of grabbing all the items in one go, you go to the checkout and back for each item. This is inefficient, right? That’s what happens in your app with N+1 queries. By identifying and solving these issues, your Laravel app becomes much faster.
What is N+1 Queries in Laravel?
N+1 queries happen when your code asks the database for data in an inefficient way.
For example, if you have a list of 100 blog posts, and you also want to show the author’s name with each post. If you don’t use eager loading, Laravel will first make one query to retrieve all 100 blog posts. Then, for each of these posts, it makes an additional query to fetch the author’s name. This results in a total of 101 queries (1 initial query for all posts + 100 individual queries for each post’s author).
Implementing N+1 Queries in Laravel with Examples
❌ Wrong Way
$posts = Post::all();
foreach ($posts as $post) {
echo $post->author->name;
}
This code makes too many database calls.
✅ Right Way:
$posts = Post::with('author')->get();
foreach ($posts as $post) {
echo $post->author->name;
}
This ‘eager loading’ approach reduces the number of queries significantly.
Benefits of handling N+1 Queries
Correctly handling N+1 queries makes your Laravel application faster and more efficient. It reduces the load on your database and speeds up the page load times for your users.
How to Disable N+1 Queries
Disabling N+1 queries in Laravel is pretty straightforward. It’s all about stopping ‘lazy loading’, which is what causes these extra queries.
Adding to AppServiceProvider: To prevent lazy loading, we add a line of code in the AppServiceProvider.php file. This file is like the control center for your application's service providers.
Code to Add: Inside the boot() method of your AppServiceProvider, add the following line:
use Illuminate\Database\Eloquent\Model;
class AppServiceProvider extends ServiceProvider
{
public function boot(): void
{
Model::preventLazyLoading();
}
}
This code tells Laravel: “Hey, let’s not do lazy loading.” It forces you to use eager loading, which is more efficient.
Making it Environment-Specific
However, you might not want this feature turned on all the time, especially on your live website. It’s usually best to use it during development. To make sure it only works in your local environment, modify the code like this:
use Illuminate\Database\Eloquent\Model;
class AppServiceProvider extends ServiceProvider
{
public function boot(): void
{
Model::preventLazyLoading(! app()->isProduction());
}
}
It enables the prevention of lazy loading only when your application is not in the ‘production’ environment. In other words, it turns on this feature when you’re developing locally, but not when your app is live and being used by real users. This way, you can catch lazy loading during development without affecting your live site.
Handling N+1 Queries in Older Laravel Versions
If you’re working with an older version of Laravel that doesn’t have built-in features to prevent N+1 queries, don’t worry. There’s a handy package called Laravel Query Detector that can help you out.
It’s a package developed by BeyondCode that detects N+1 query problems in your Laravel application. It works similarly to the built-in features of newer Laravel versions, helping you identify inefficient database queries.
Installation:
You can easily install this package via Composer. Just run the following command in your project:
composer require beyondcode/laravel-query-detector --dev
This command installs the package as a development dependency, meaning it won’t be included in your production environment.
How It Works:
Once installed, the Laravel Query Detector automatically checks for N+1 query issues during your app’s execution. If it finds any, it will alert you. This way, you can spot and fix these issues before they affect your application’s performance.
For more detailed information on how to configure and use this package, you can visit its GitHub page: Laravel Query Detector on GitHub.
Conclusion
Understanding N+1 query issues in Laravel and learning how to avoid them is crucial for any developer. It ensures your applications run smoothly and efficiently. Give these practices a try in your projects and feel free to share your experiences or ask questions!
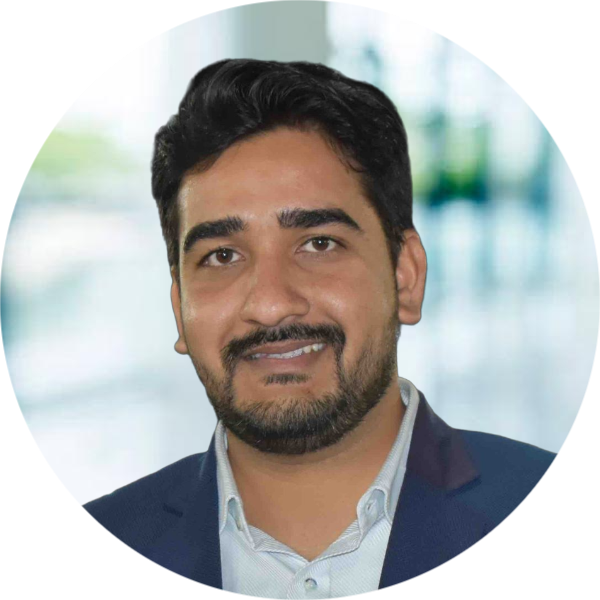
Author: Manish Kumar
I've been working with PHP for over 11 years and focusing on Laravel for the last 6 years. During this time, I've taken part in big projects and gotten to know tools like Laravel Livewire really well. Besides working on projects, I enjoy writing about Laravel on LinkedIn and Medium to share what I've learned. I'm always looking to learn more and share my knowledge with others. Right now, I'm diving into new areas like using Docker to make web apps run better and exploring how to build them using microservices. My goal is to keep learning and help others do the same in the world of web development.
Recent Posts
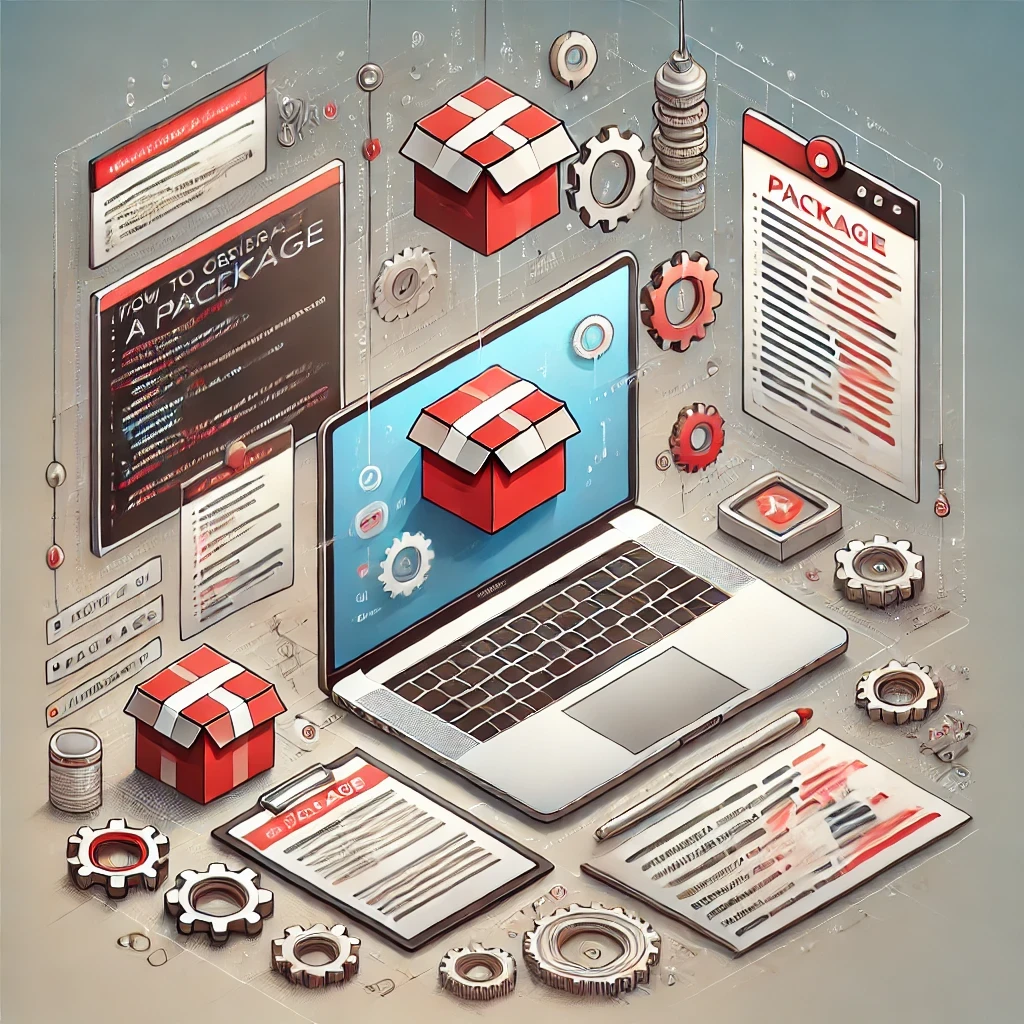
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
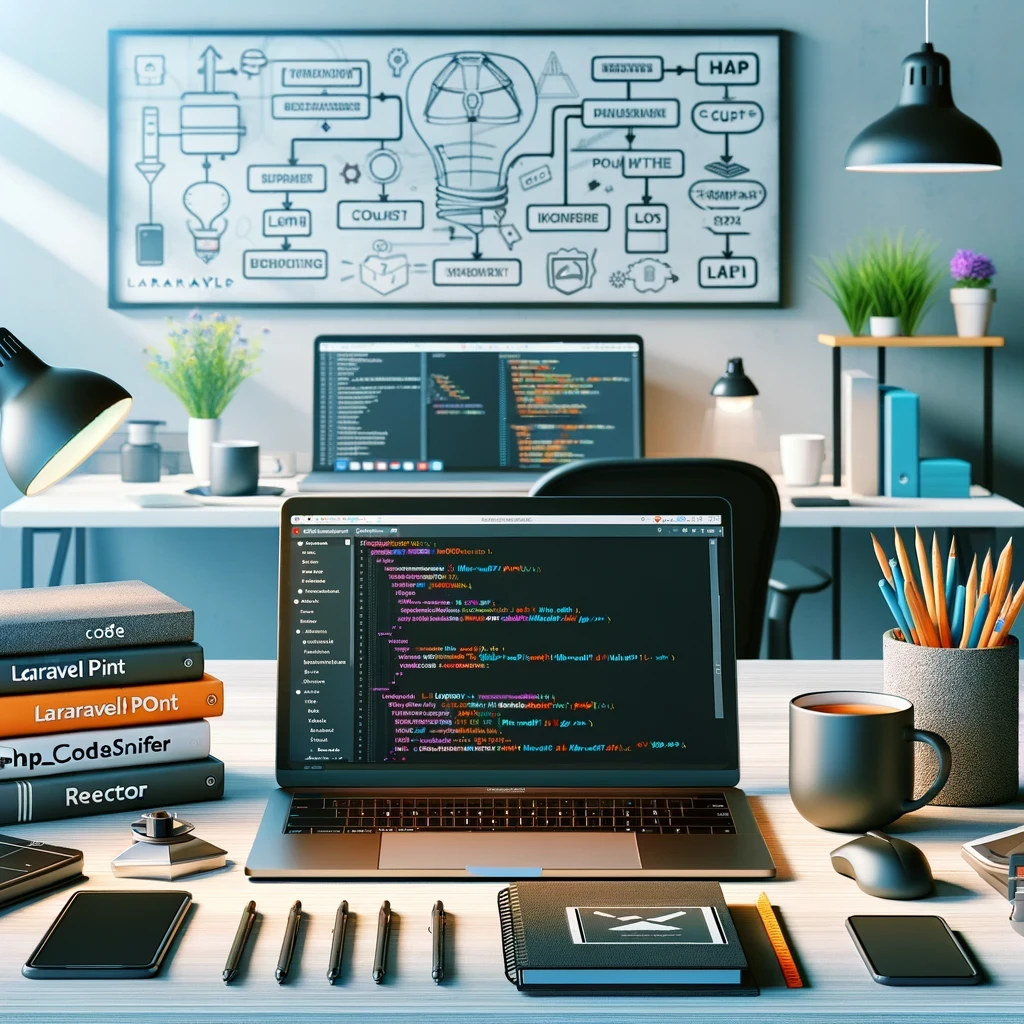
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
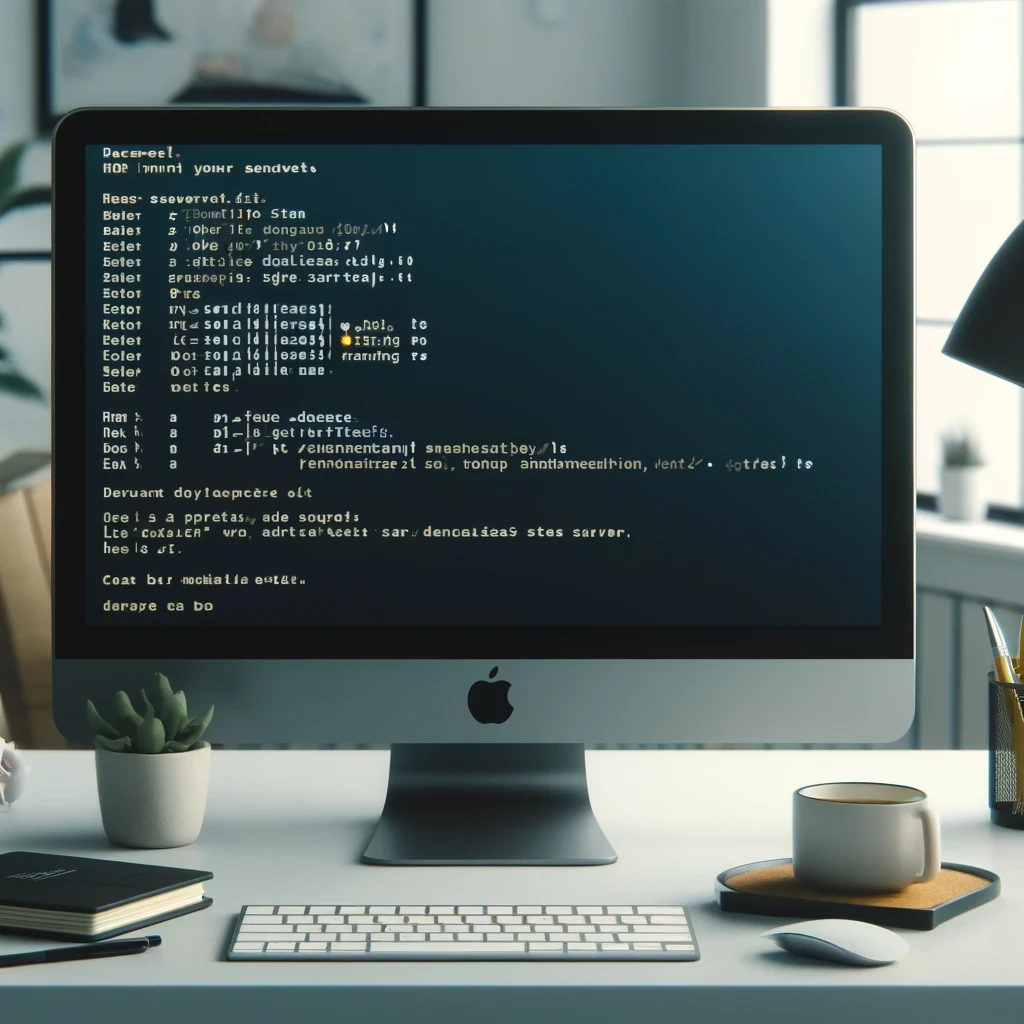
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
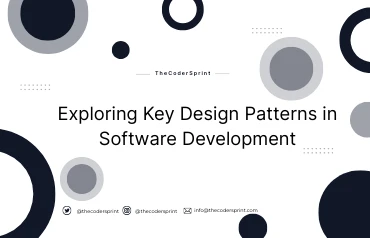
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
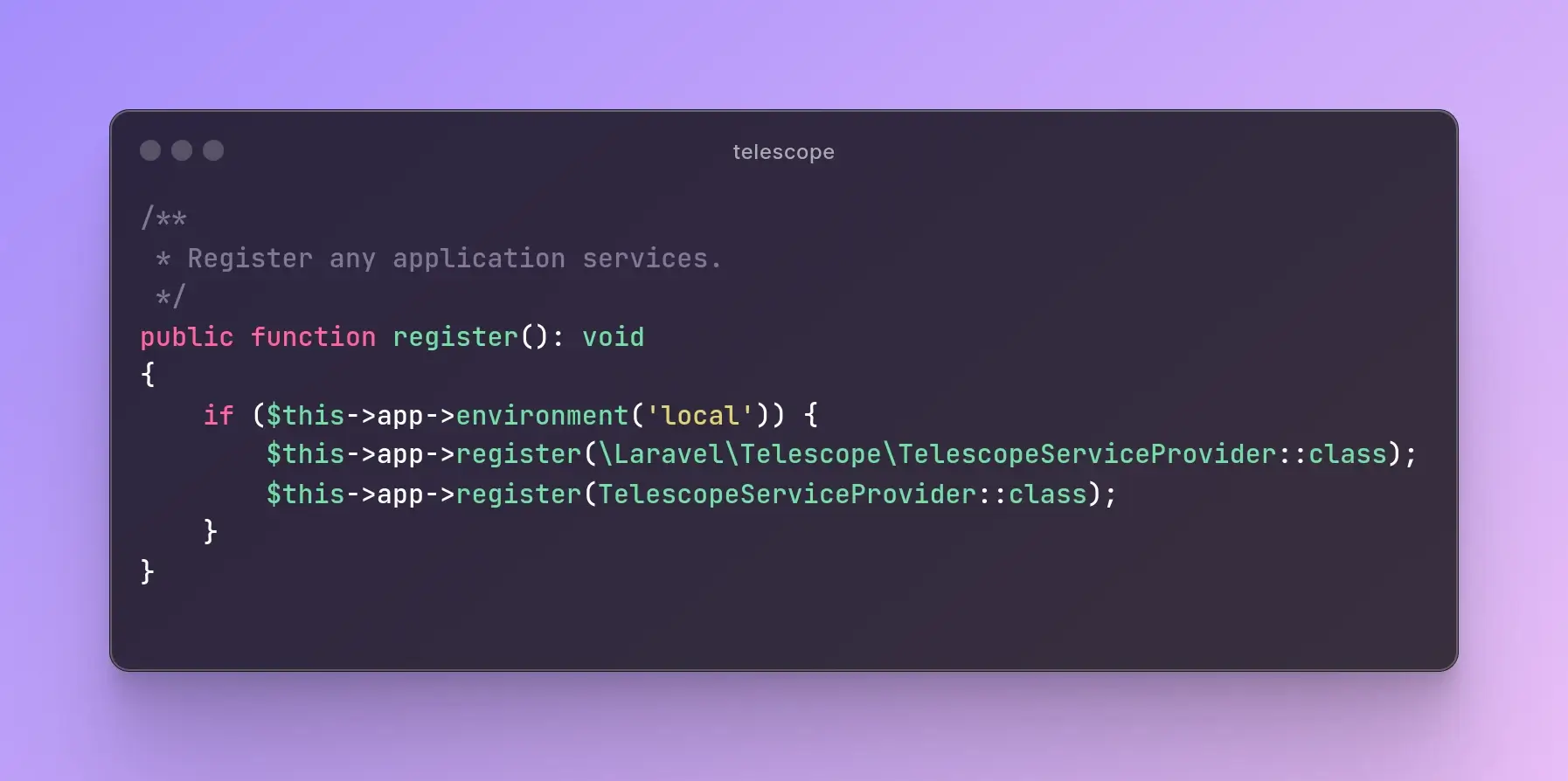
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles