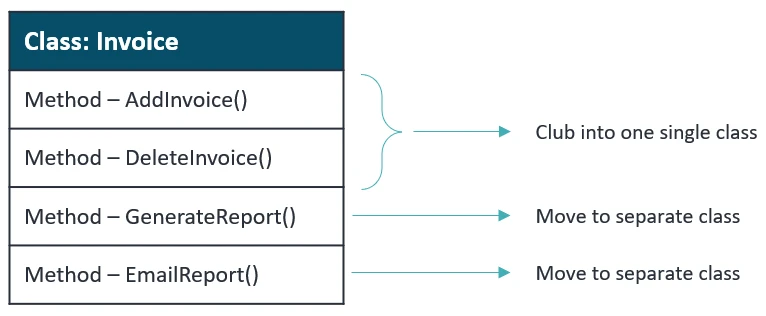
Simple Guide to Single Responsibility Principle in Laravel
Hey everyone! Today, we’re talking about something called the Single Responsibility Principle (SRP) and how to use it in Laravel. In simple words, SRP means that each part of your Laravel app should do one thing and do it well. This makes your app easier to handle and fix when needed.
- What is SRP in Laravel?
SRP is a smart way to organize your code. In Laravel, this means making sure things like Controllers and Models each have one job. For example, a Controller should only handle user requests, not also send emails or directly query the database.
- Avoiding Mistakes
Sometimes, we put too much into one part of our Laravel app. Like making a class that does too many things — handling user data, making decisions, and sending emails. That’s a no-no in SRP!
-
Applying SRP with Examples
-
- User Sign-Up
❌ Not the Best Way
A Controller does everything — checks user info, creates a user, and sends welcome emails.
class UserController extends Controller {
public function store(Request $request) {
$validatedData = $request->validate([
'name' => 'required',
'email' => 'required|email',
'password' => 'required'
]);
$user = User::create($validatedData);
Mail::to($user->email)->send(new WelcomeEmail($user));
return redirect()->route('users.index');
}
}
✅ A Better Way
Break it down. One class checks the user info, another handles user creation, and a third sends emails.
class UserController extends Controller {
protected $userService;
public function __construct(UserService $userService) {
$this->userService = $userService;
}
public function store(StoreUserRequest $request) {
$user = $this->userService->createUser($request->validated());
$this->userService->sendWelcomeEmail($user);
return redirect()->route('users.index');
}
}
class UserService {
public function createUser(array $data) {
return User::create($data);
}
public function sendWelcomeEmail(User $user) {
Mail::to($user->email)->send(new WelcomeEmail($user));
}
}
class StoreUserRequest extends FormRequest {
public function rules() {
return [
'name' => 'required',
'email' => 'required|email',
'password' => 'required'
];
}
}
-
- Making Reports
❌ Not the Best Way
A Controller that gets data, processes it, and makes a report.
class ReportController extends Controller {
public function downloadReport() {
$data = Report::getReportData();
$report = ReportGenerator::generate($data);
return response()->download($report);
}
}
✅ A Better Way
Use a service to prepare the report. Keep the Controller simple.
class ReportController extends Controller {
protected $reportService;
public function __construct(ReportService $reportService) {
$this->reportService = $reportService;
}
public function downloadReport() {
$report = $this->reportService->prepareReport();
return response()->download($report);
}
}
class ReportService {
public function prepareReport() {
$data = Report::getReportData();
return ReportGenerator::generate($data);
}
}
- Why SRP is Great
Using SRP makes your code cleaner and your life easier. When each part of your app has just one job, it’s easier to fix bugs and add new features.
Conclusion
So, that’s the Single Responsibility Principle in a nutshell! By making sure each part of your Laravel app does just one thing, you’ll have a much easier time managing your code. Give it a try in your next project and feel free to share how it goes in the comments!
- Tags:
- #Laravel
- #Debugging
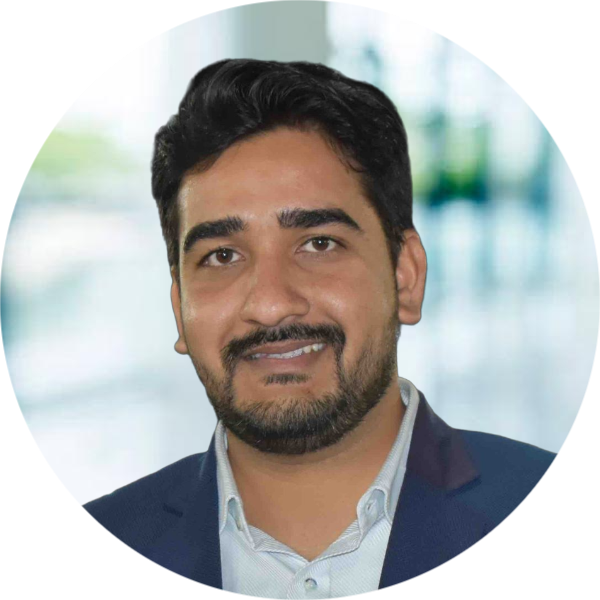
Author: Manish Kumar
I've been working with PHP for over 11 years and focusing on Laravel for the last 6 years. During this time, I've taken part in big projects and gotten to know tools like Laravel Livewire really well. Besides working on projects, I enjoy writing about Laravel on LinkedIn and Medium to share what I've learned. I'm always looking to learn more and share my knowledge with others. Right now, I'm diving into new areas like using Docker to make web apps run better and exploring how to build them using microservices. My goal is to keep learning and help others do the same in the world of web development.
Recent Posts
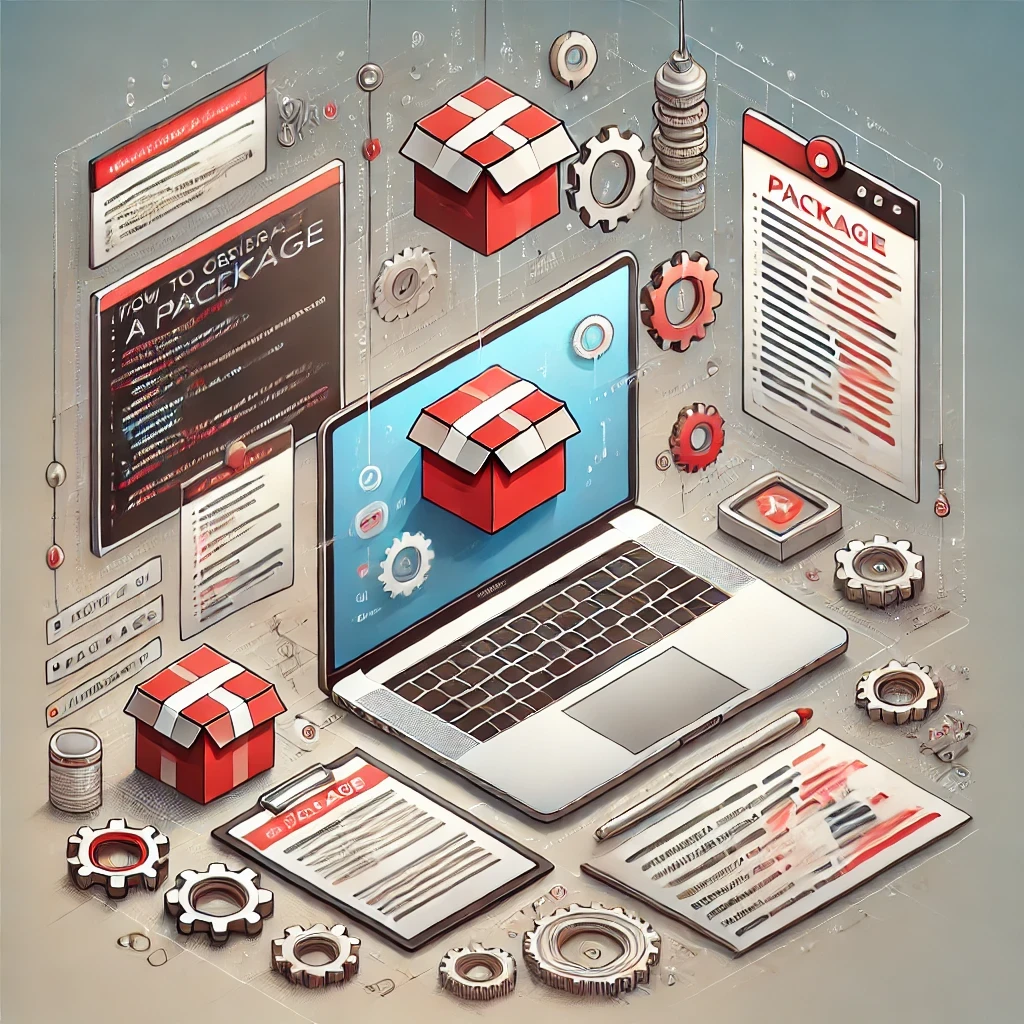
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
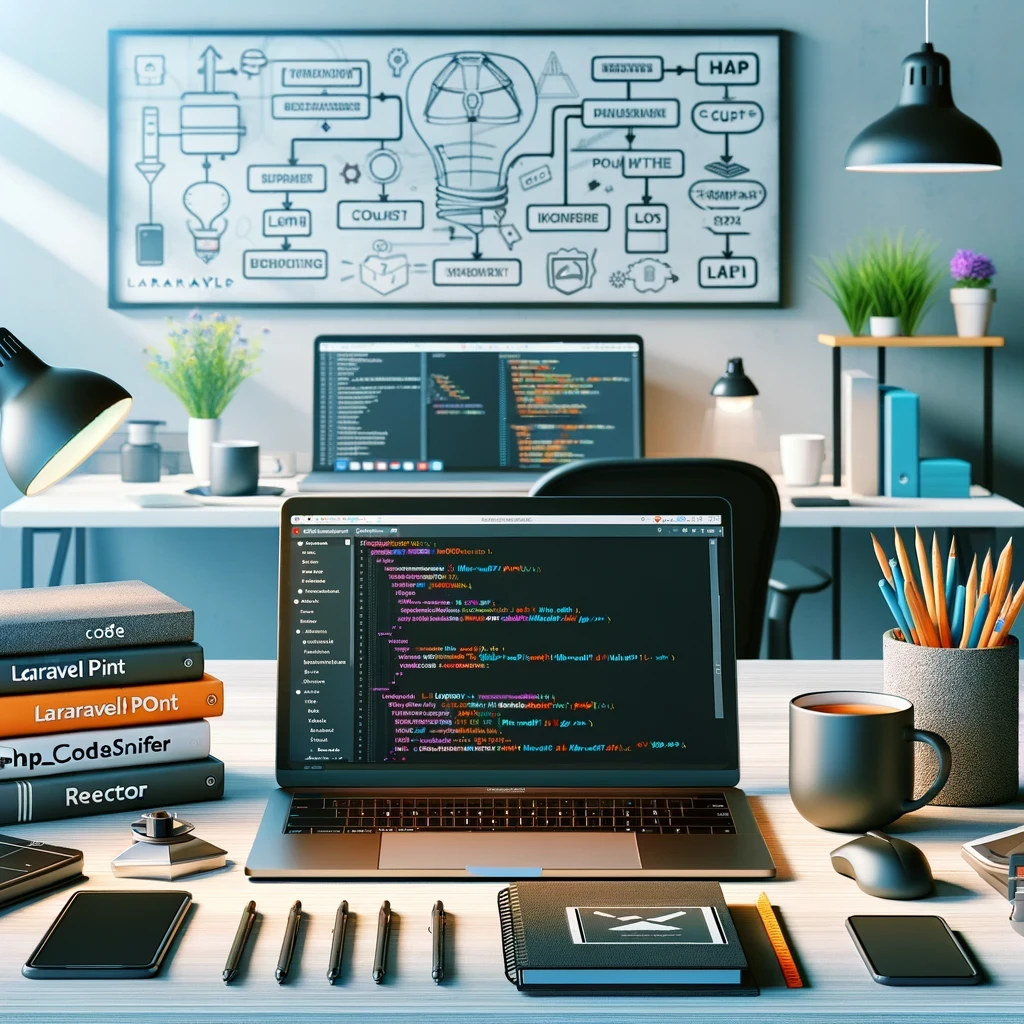
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
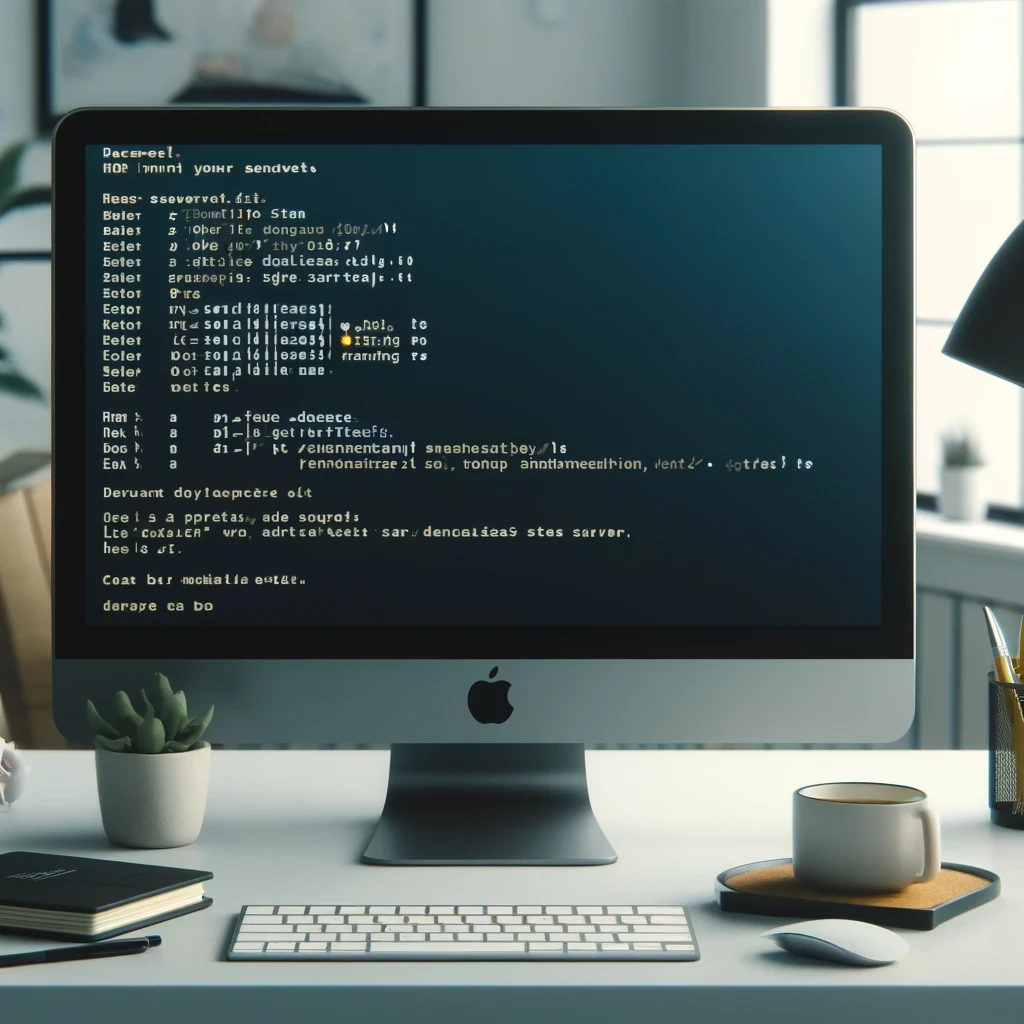
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
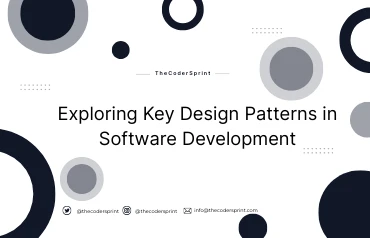
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
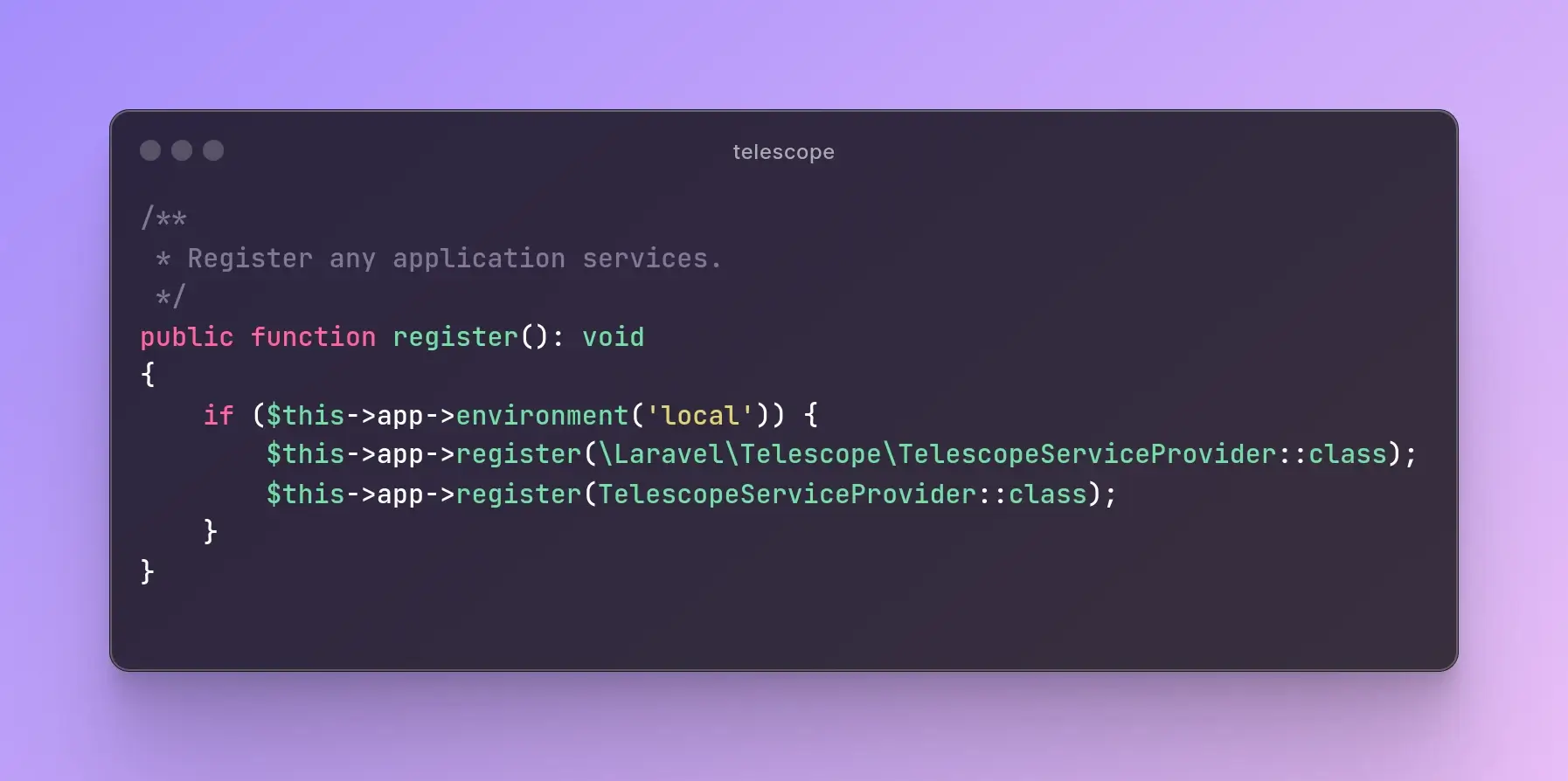
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles