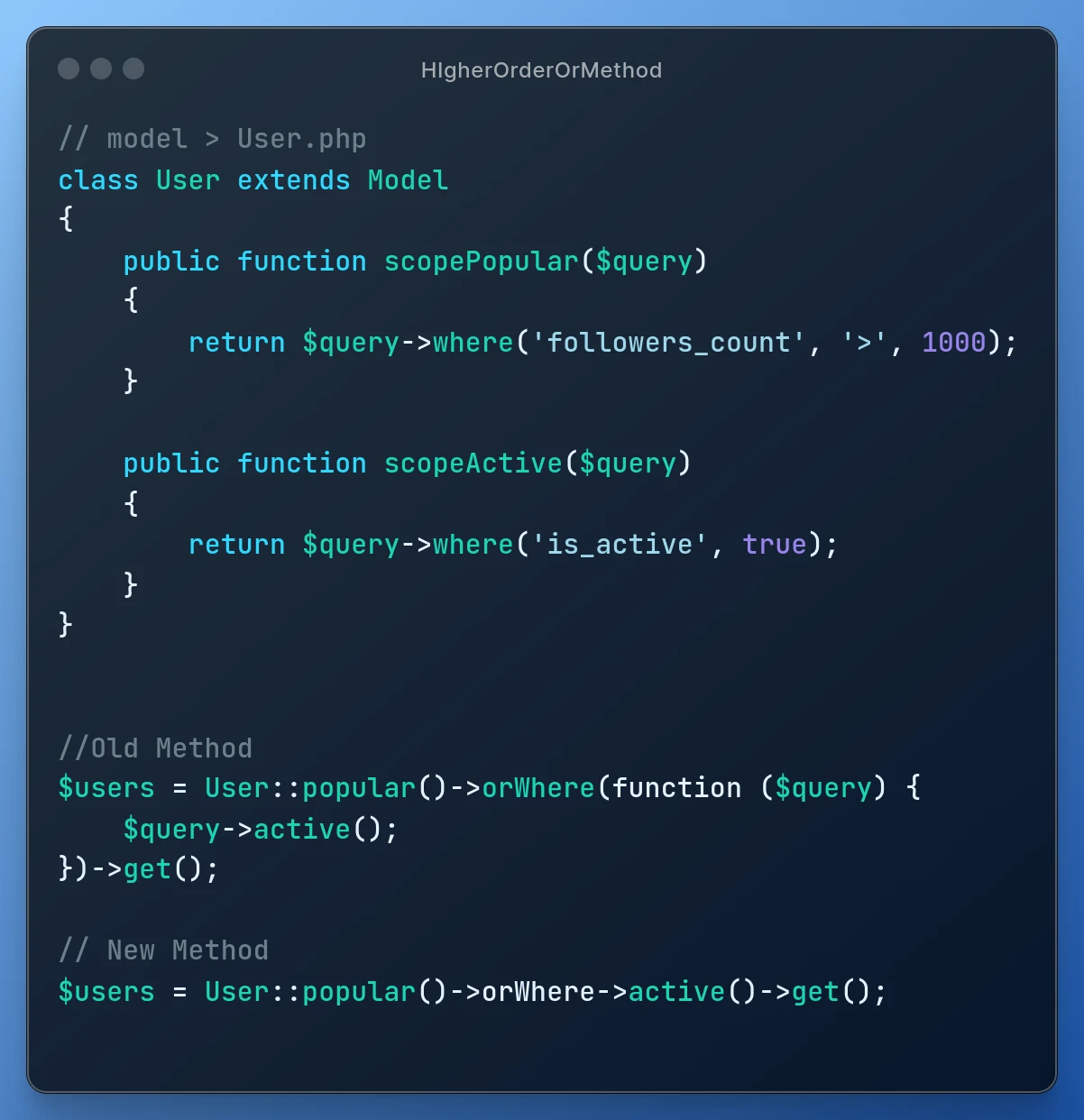
Simplifying Laravel Queries with "Higher Order" OrWhere Method
In the world of Laravel development, writing eloquent queries is like composing poetry. It's about crafting elegant and efficient solutions to fetch the data you need. Laravel provides various tools to streamline this process, and one such tool is the Higher Order OrWhere method.
In Laravel, scopes are a way to encapsulate common query constraints. They help keep your code organized and maintainable. But what if you need to combine multiple scopes conditionally?
Traditionally, you might resort to using closures within the orWhere
method.
However, Laravel offers a more concise and readable alternative: the Higher Order OrWhere method.
Let's dive into an example to illustrate the power of this method. Imagine you have a User model with scopes for fetching popular users and active users:
class User extends Model
{
public function scopePopular($query)
{
return $query->where('followers_count', '>', 1000);
}
public function scopeActive($query)
{
return $query->where('is_active', true);
}
}
Before Laravel introduced the Higher Order OrWhere method, combining these scopes conditionally required using a closure:
$users = User::popular()->orWhere(function ($query) {
$query->active();
})->get();
While this works perfectly fine, it can become verbose and less readable as your queries grow in complexity. Now, let's rewrite the same query using the Higher Order OrWhere method:
$users = User::popular()->orWhere->active()->get();
Isn't that much cleaner and easier to understand? By chaining ->orWhere->
, Laravel automatically applies the orWhere
condition to the subsequent scope, in this case, active()
.
This approach not only simplifies your code but also enhances readability, making it easier for you and your team to understand the query's intent at a glance.
Moreover, this method can be chained multiple times, allowing you to combine as many scopes as needed:
$users = User::popular()
->orWhere->active()
->orWhere->verified()
->get();
Each ->orWhere->
call applies the subsequent scope while maintaining a clear and concise syntax.
In addition to improving readability, the Higher Order OrWhere method also promotes code reusability. Since scopes encapsulate common query constraints, you can easily reuse them in different combinations without duplicating code.
Conclusion
To summarize, the Higher Order OrWhere method in Laravel offers a cleaner and more concise way to combine scopes conditionally. By chaining ->orWhere->
, you can streamline your queries, improve readability, and promote code reusability.
Happy coding!
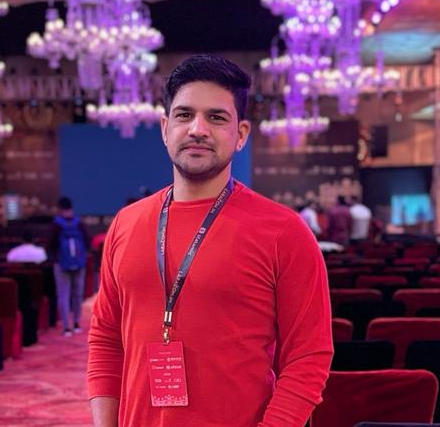
Author: Sonu Singh
With over 7 years of dedicated experience in web development, I've traversed through the dynamic landscape of digital technology, honing my skills and expertise across various frameworks and content management systems. My journey began with the exploration of frameworks like CodeIgniter, CakePHP, Yii, and eventually, I found my passion in Laravel - a framework that resonated deeply with my love for elegant, efficient code. Throughout my career, I've had the privilege to contribute to numerous projects, ranging from small-scale ventures to large, enterprise-level solutions. Each project has been a unique opportunity to push boundaries, solve intricate challenges, and deliver innovative solutions tailored to meet client needs. One of my proudest accomplishments includes the development of a SaaS-based project using Laravel and MySQL. This venture not only showcased my technical prowess but also demonstrated my ability to conceptualize, design, and execute scalable solutions that drive business growth. In addition to my proficiency in development, I've also delved into server deployment and query optimization, ensuring that every aspect of a project - from its codebase to its infrastructure - is optimized for peak performance and reliability.
Recent Posts
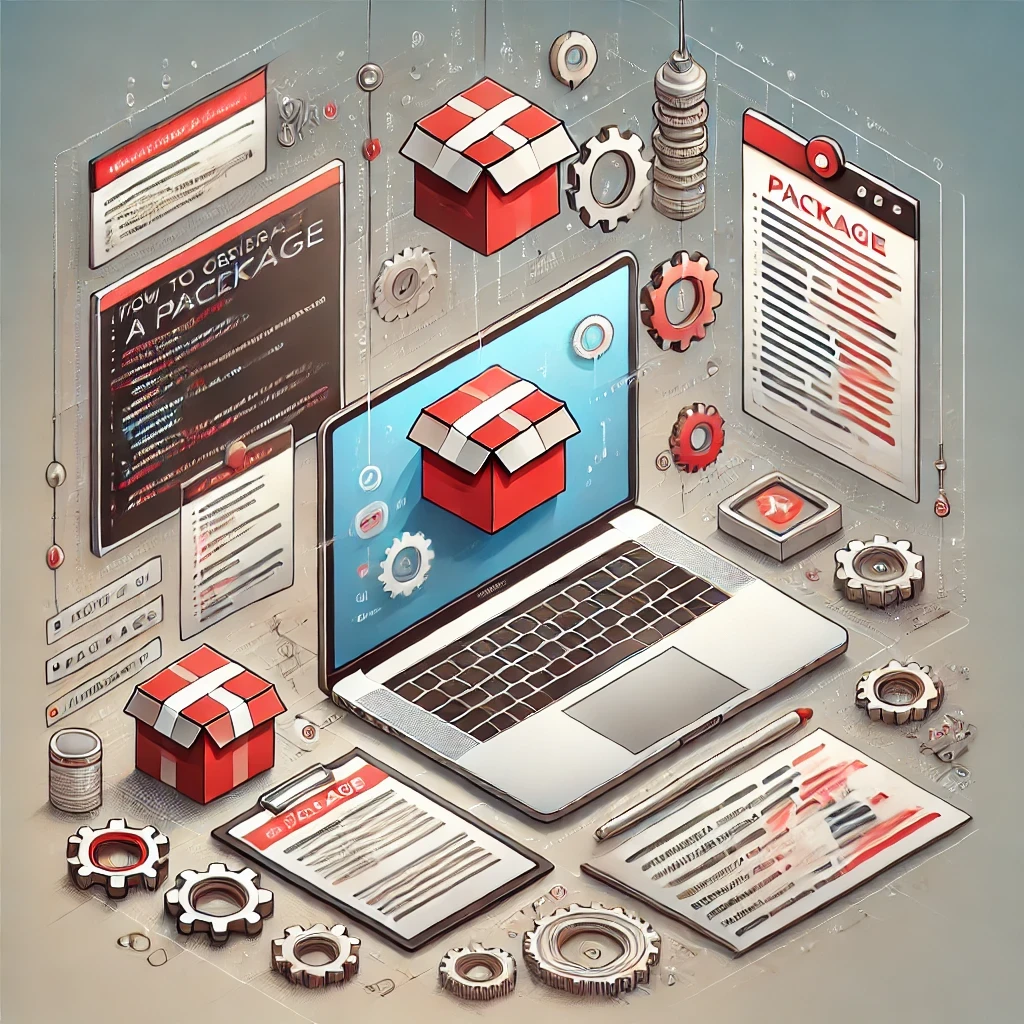
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
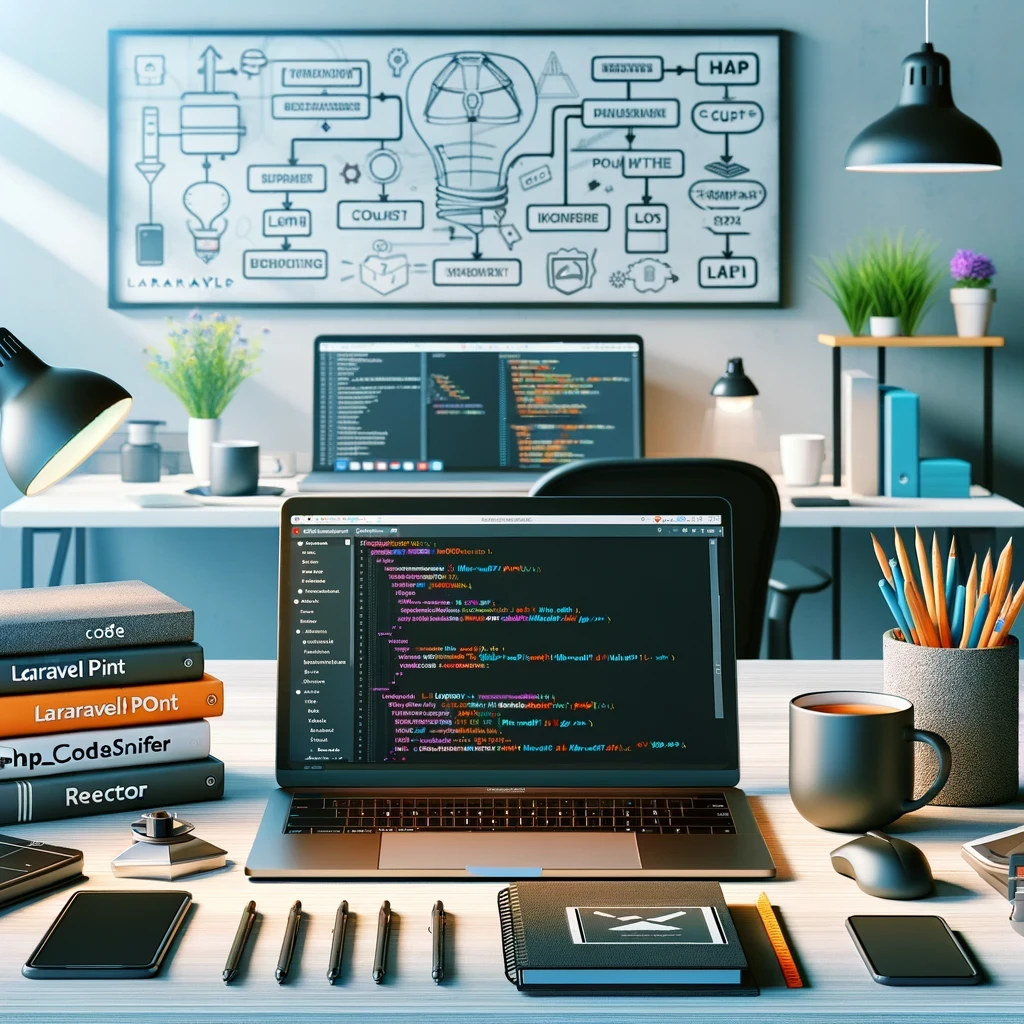
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
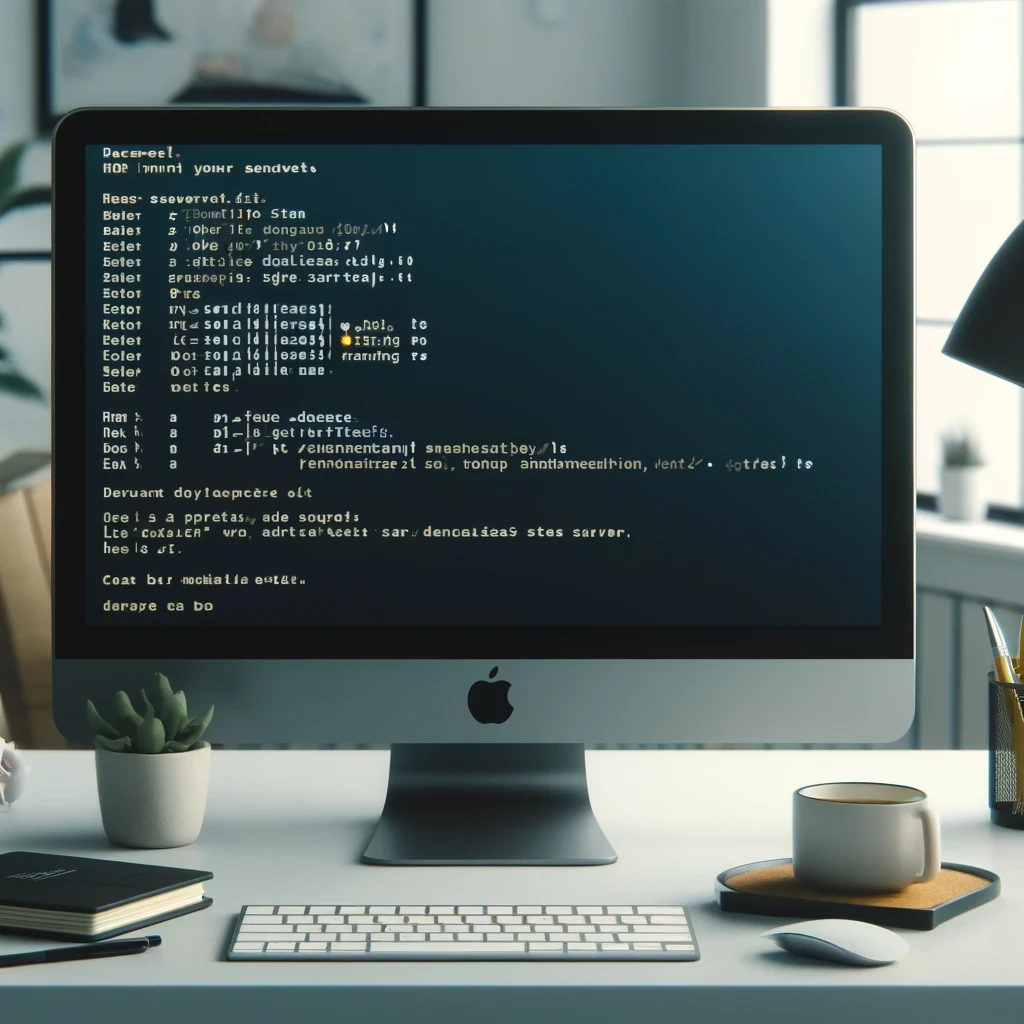
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
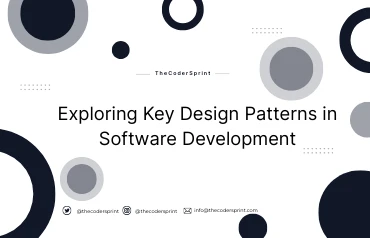
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
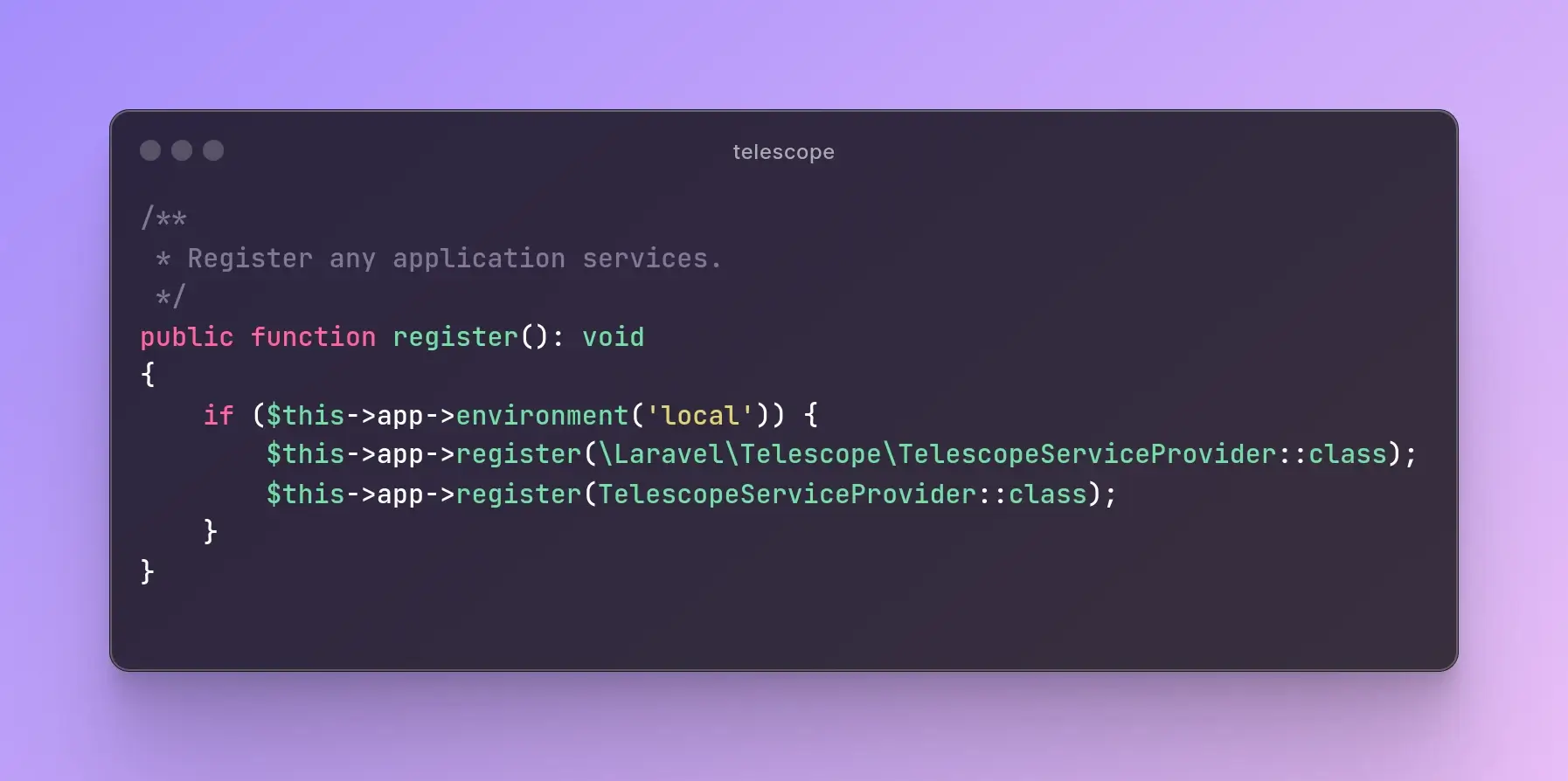
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles