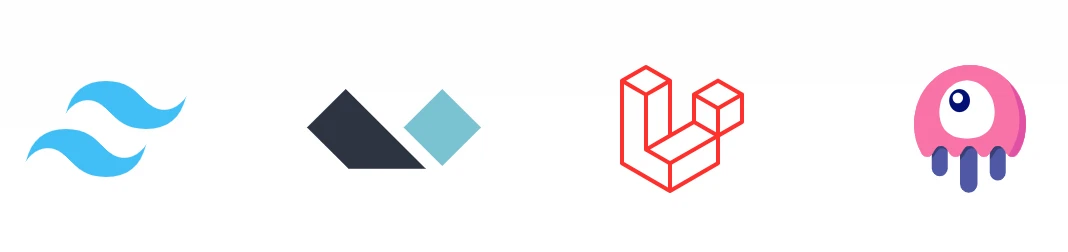
The Magic of TallStack Technology: A Step-by-Step Tutorial
TallStack is an acronym for Tailwind CSS, Alpine.js, Laravel, and Livewire. This guide will give you an insight into each component of the stack, how they work together, and show you a simple working example.
Understanding TallStack
The magic of TallStack lies in its synergy. Each part of the stack complements the others, culminating in a comprehensive and robust tech stack for modern web applications.
-
Tailwind CSS is a utility-first CSS framework used for creating UIs swiftly and with less coding.
-
Alpine.js offers a minimalist framework for client-side JavaScript. It provides similar functionalities as Vue or React but with a much smaller footprint.
-
Laravel is a PHP web framework for server-side rendering, providing a clean and elegant syntax.
-
Livewire is a full-stack framework for Laravel that enables you to create dynamic, JavaScript-rich applications using Laravel Blade as your templating language.
Setting Up The Development Environment
Installing Laravel: First, you need to install Laravel. Assuming you have PHP and Composer (a PHP dependency manager) installed, you can create a new Laravel project by running the following command in your terminal:
composer create-project - prefer-dist laravel/laravel blog
Installing Livewire: Next, we install Livewire using Composer:
composer require livewire/livewire
Installing Tailwind CSS and Alpine.js: The easiest way to install both Tailwind CSS and Alpine.js is via Laravel Mix. Laravel Mix is a webpack wrapper included with Laravel, which simplifies the API. First, install Laravel Mix dependencies:
npm install
Then, install Tailwind CSS and Alpine.js:
npm install tailwindcss @tailwindcss/forms @tailwindcss/typography alpinejs
Building A Simple Blog
Now that our environment is set up, let’s create a simple blog application.
- Laravel Migrations
Let’s start by creating a migration for our posts table:
Go to the new migration file and update the up()
method:
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('body');
$table->timestamps();
});
}
Next, run the migration:
php artisan migrate
- Creating the Post Model and Controller
Create a new Laravel Model and Controller:
php artisan make:model Post -mc
The -mc
flag creates both a model and a controller. Update your Post model and controller according to your needs.
- Livewire Component
Let’s create a Livewire component to display our posts:
php artisan make:livewire post-component
This will create two new files: a class file and a view file. In the class file, fetch the posts in the render()
method:
use App\Models\Post;
public function render()
{
return view('livewire.post-component', [
'posts' => Post::latest()->get(),
]);
}
In the view file, loop over the posts and display them:
<div>
@foreach($posts as $post)
<h2>{{ $post->title }}</h2>
<p>{{ $post->body }}</p>
@endforeach
</div>
- Alpine.js Interactivity
Let’s add some interactivity with Alpine.js. For this example, we’ll add a “Show More” button that displays the full post:
<div>
@foreach($posts as $post)
<h2>{{ $post->title }}</h2>
<p x-data="{ open: false }">
<span x-show="!open">{{ Str::limit($post->body, 100) }}</span>
<span x-show="open">{{ $post->body }}</span>
<button @click="open = !open">Show More</button>
</p>
@endforeach
</div>
- Styling with Tailwind CSS
Finally, let’s use Tailwind CSS to add some styling:
<div class="p-4">
@foreach($posts as $post)
<div class="mb-4">
<h2 class="text-xl font-bold">{{ $post->title }}</h2>
<p x-data="{ open: false }" class="text-gray-700">
<span x-show="!open">{{ Str::limit($post->body, 100) }}</span>
<span x-show="open">{{ $post->body }}</span>
<button class="text-blue-500" @click="open = !open">Show More</button>
</p>
</div>
@endforeach
</div>
And there you have it! A simple blog built with the TallStack.
TallStack provides a powerful and streamlined way of building modern web applications. Whether you’re building a small project or an enterprise application, TallStack provides the tools and ecosystem to help you succeed. Happy coding!
- Tags:
- #Laravel
- #Tailwind CSS
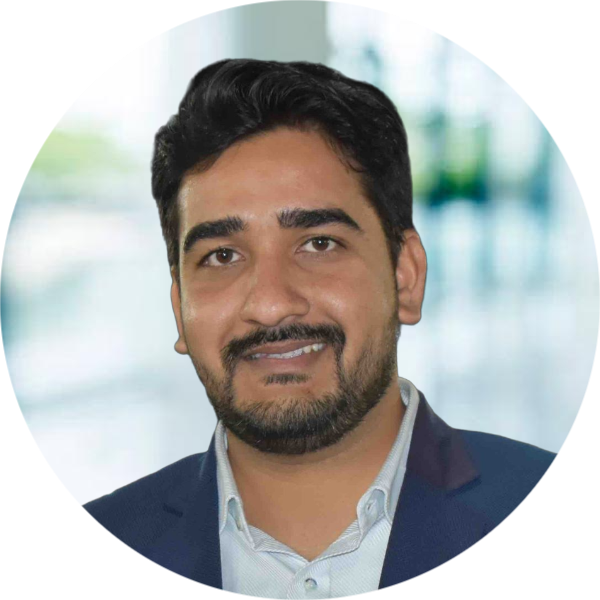
Author: Manish Kumar
I've been working with PHP for over 11 years and focusing on Laravel for the last 6 years. During this time, I've taken part in big projects and gotten to know tools like Laravel Livewire really well. Besides working on projects, I enjoy writing about Laravel on LinkedIn and Medium to share what I've learned. I'm always looking to learn more and share my knowledge with others. Right now, I'm diving into new areas like using Docker to make web apps run better and exploring how to build them using microservices. My goal is to keep learning and help others do the same in the world of web development.
Recent Posts
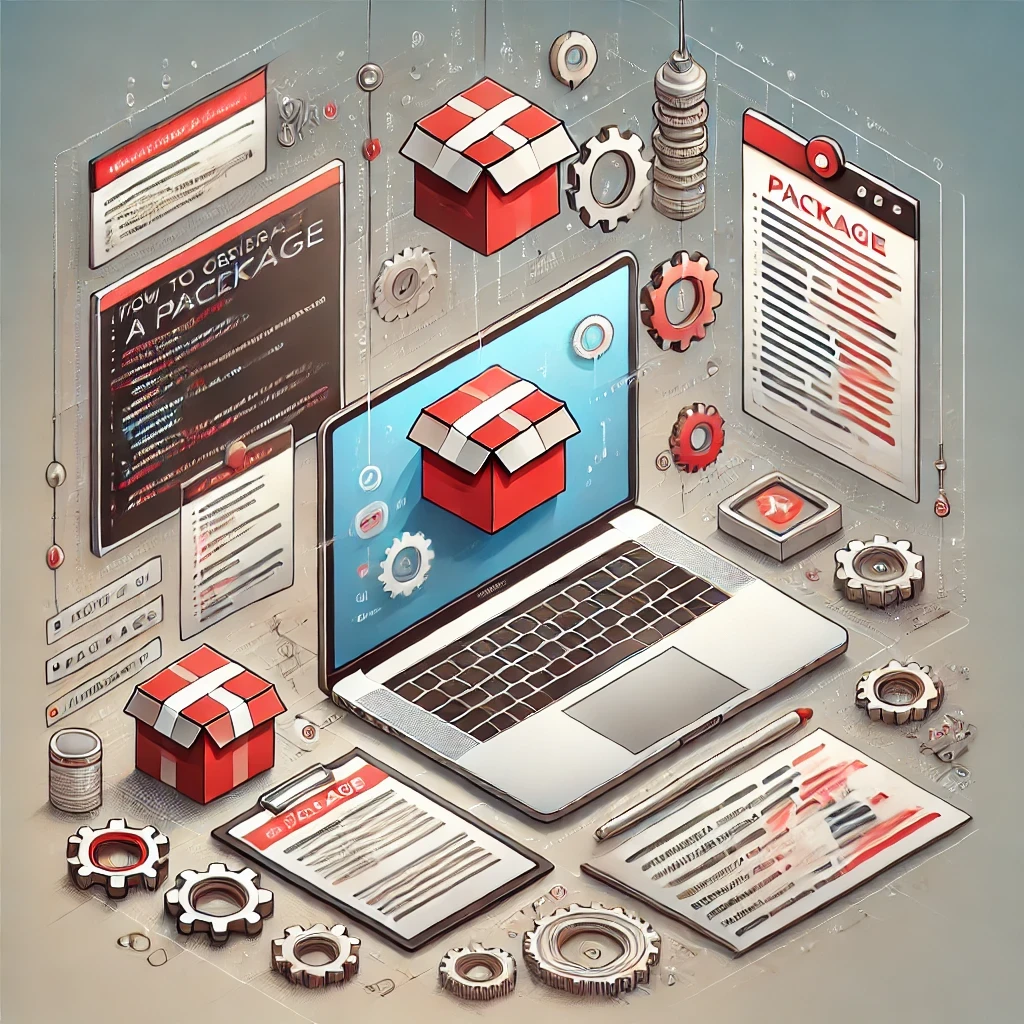
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
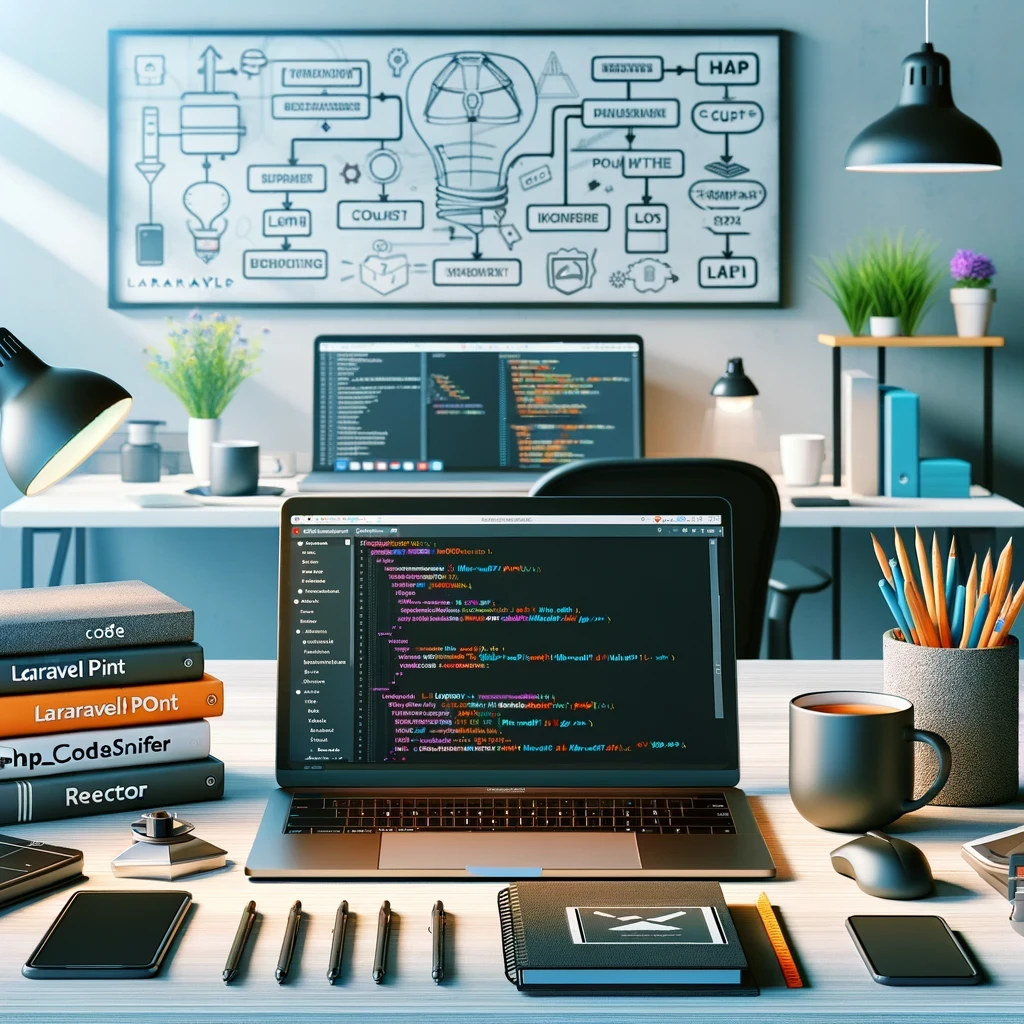
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
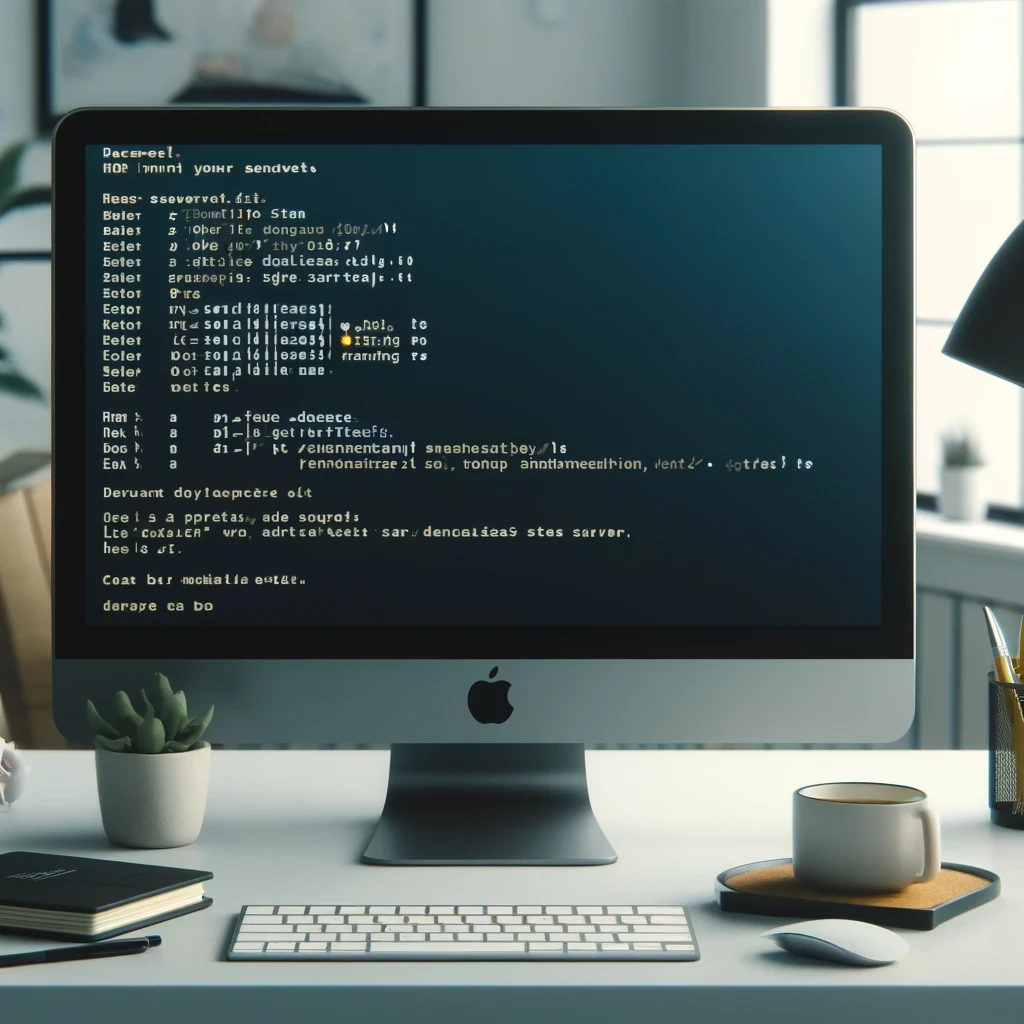
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
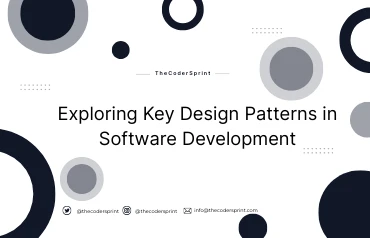
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
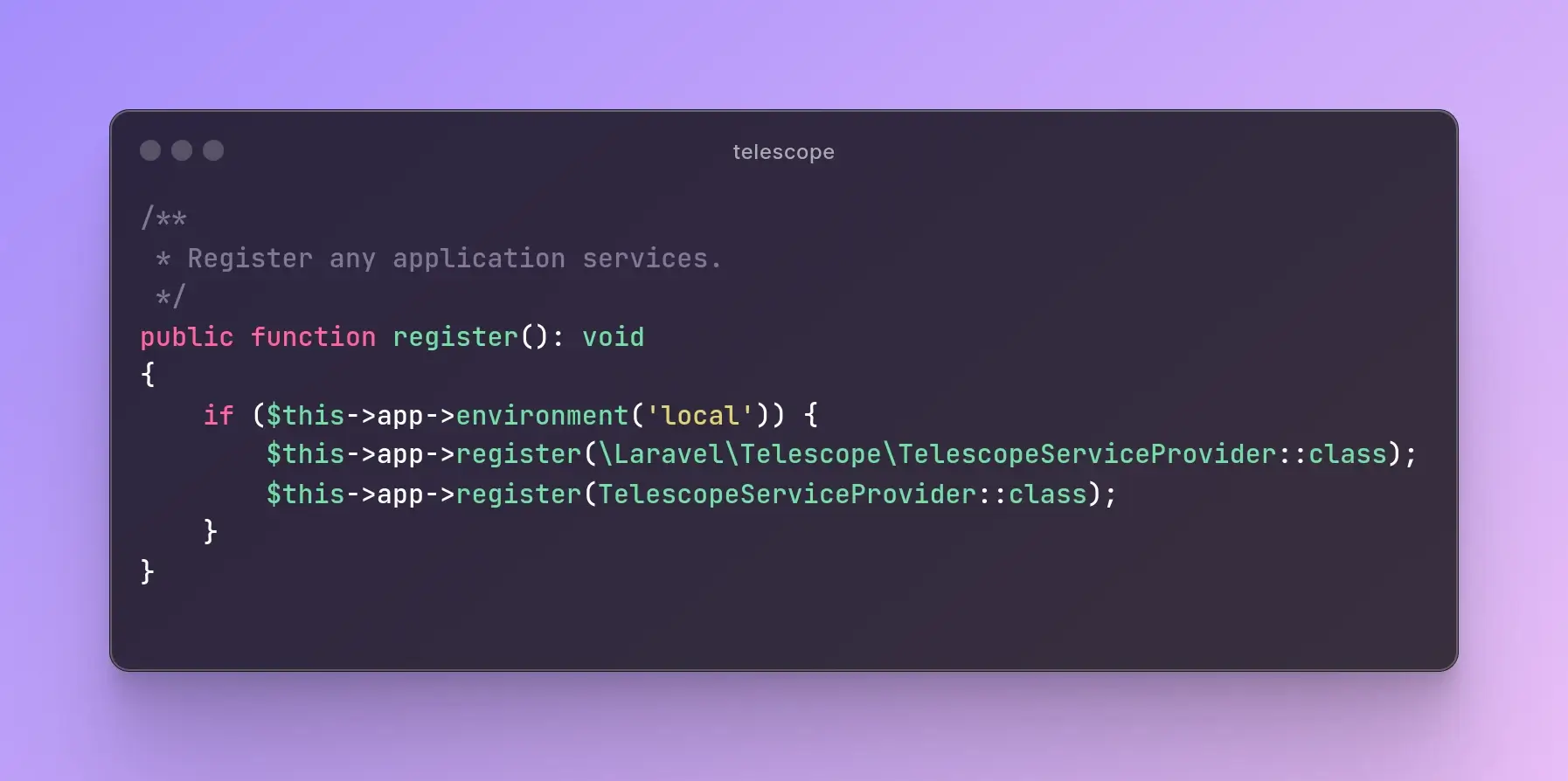
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles