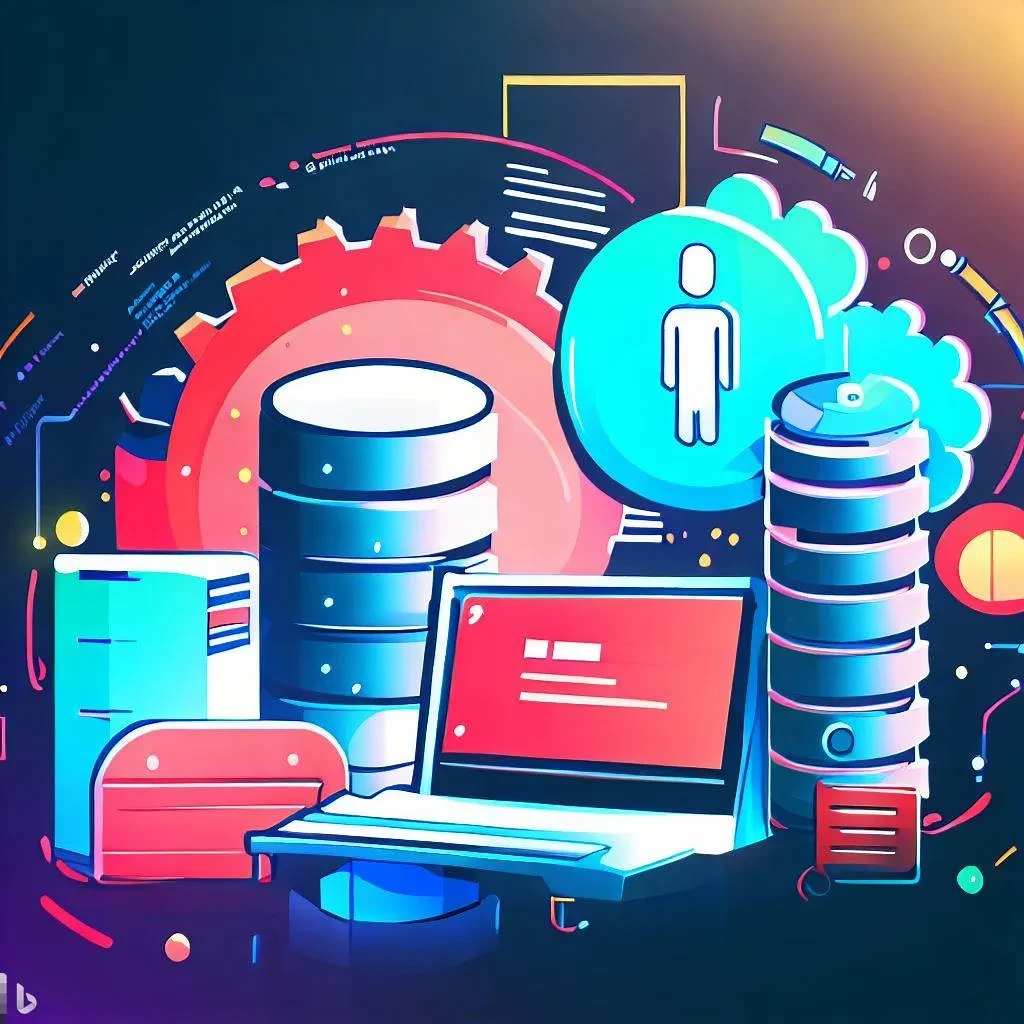
Understanding REST API: A Simple Guide
REST API stands for Representational State Transfer Application Programming Interface. It’s a set of rules that allows software to talk to each other via the internet. REST APIs use standard web methods, which are like different types of requests you can make to access or change data on a server.
The Methods of REST API:
REST APIs mainly involve five methods: GET, POST, PUT, DELETE, and PATCH. Let’s explore each with PHP code examples:
GET Method
Use: To get or retrieve data from a server.
Idempotent: Yes (calling it any number of times produces the same result).
Key Point: GET requests don’t change the server’s data.
PHP Example:
// Using cURL to send a GET request
$curl = curl_init('http://example.com/api/users');
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($curl);
curl_close($curl);
POST Method
Use: To send data to a server to create a new resource.
Idempotent: No (calling it multiple times will create multiple resources).
Key Point: POST requests create new data on the server.
PHP Example:
// Using cURL to send a POST request
$curl = curl_init('http://example.com/api/users');
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, ['name' => 'John', 'email' => '[email protected]']);
$response = curl_exec($curl);
curl_close($curl);
PUT Method
Use: To update existing data or create it if it doesn’t exist.
Idempotent: Yes (the state of the server will be the same after one or multiple calls).
Key Point: PUT can update or create data based on the URL.
PHP Example:
// Using cURL to send a PUT request
$curl = curl_init('http://example.com/api/users/123');
curl_setopt($curl, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($curl, CURLOPT_POSTFIELDS, ['name' => 'Alice', 'email' => '[email protected]']);
$response = curl_exec($curl);
curl_close($curl);
DELETE Method
Use: To remove data from the server.
Idempotent: Yes (after the resource is deleted, subsequent calls do nothing).
Key Point: DELETE requests remove data from the server.
PHP Example:
// Using cURL to send a DELETE request
$curl = curl_init('http://example.com/api/users/123');
curl_setopt($curl, CURLOPT_CUSTOMREQUEST, 'DELETE');
$response = curl_exec($curl);
curl_close($curl);
PATCH Method
Use: For partial updates to data.
Idempotent: It can be, but depends on how it’s implemented.
Key Point: PATCH is used for updating part of the data.
PHP Example:
// Using cURL to send a PATCH request
$curl = curl_init('http://example.com/api/users/123');
curl_setopt($curl, CURLOPT_CUSTOMREQUEST, 'PATCH');
curl_setopt($curl, CURLOPT_POSTFIELDS, ['email' => '[email protected]']);
$response = curl_exec($curl);
curl_close($curl);
Difference Between PUT and POST (and Other Methods):
PUT vs POST
PUT is idempotent: sending the same request multiple times will not change the outcome after the initial request. It’s used for updating resources and can also be used to create a resource at a specific URL if it doesn’t exist.
POST is not idempotent: it will result in the creation of a new resource every time the request is made. It’s used for creating resources where the server controls the new resource’s URL.
PUT vs PATCH:
PUT is used for updating the entire resource.
PATCH is used for partial updates on a resource.
GET vs POST:
GET requests should not change the state of the server. They are used only to retrieve data.
POST can change the server’s state by creating resources.
Common Interview Questions:
Can PUT be used to create a resource?
Yes, PUT can create a resource if it does not exist at the specified URL. Why is POST not idempotent?
Because making multiple POST requests will typically create multiple instances of the same resource.
When to use PATCH instead of PUT?
Use PATCH when you need to update only a part of the resource, not the entire resource.
Are GET requests always safe?
GET requests are considered “safe” in the sense that they do not modify the resource. However, they might still have other effects, like logging or triggering other processes.
Conclusion
REST APIs are an essential part of web development, allowing different software to communicate and exchange data. By understanding and using these methods effectively, you can make your web applications more dynamic and connected. Remember, REST API is all about how you request and send data over the internet. Happy coding!
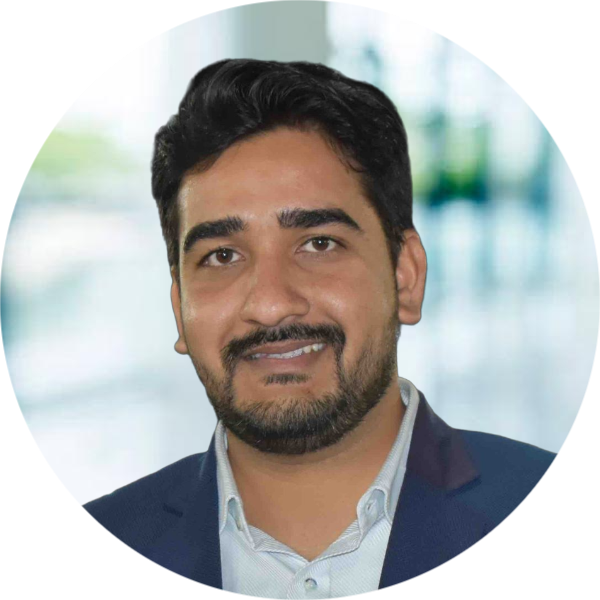
Author: Manish Kumar
I've been working with PHP for over 11 years and focusing on Laravel for the last 6 years. During this time, I've taken part in big projects and gotten to know tools like Laravel Livewire really well. Besides working on projects, I enjoy writing about Laravel on LinkedIn and Medium to share what I've learned. I'm always looking to learn more and share my knowledge with others. Right now, I'm diving into new areas like using Docker to make web apps run better and exploring how to build them using microservices. My goal is to keep learning and help others do the same in the world of web development.
Recent Posts
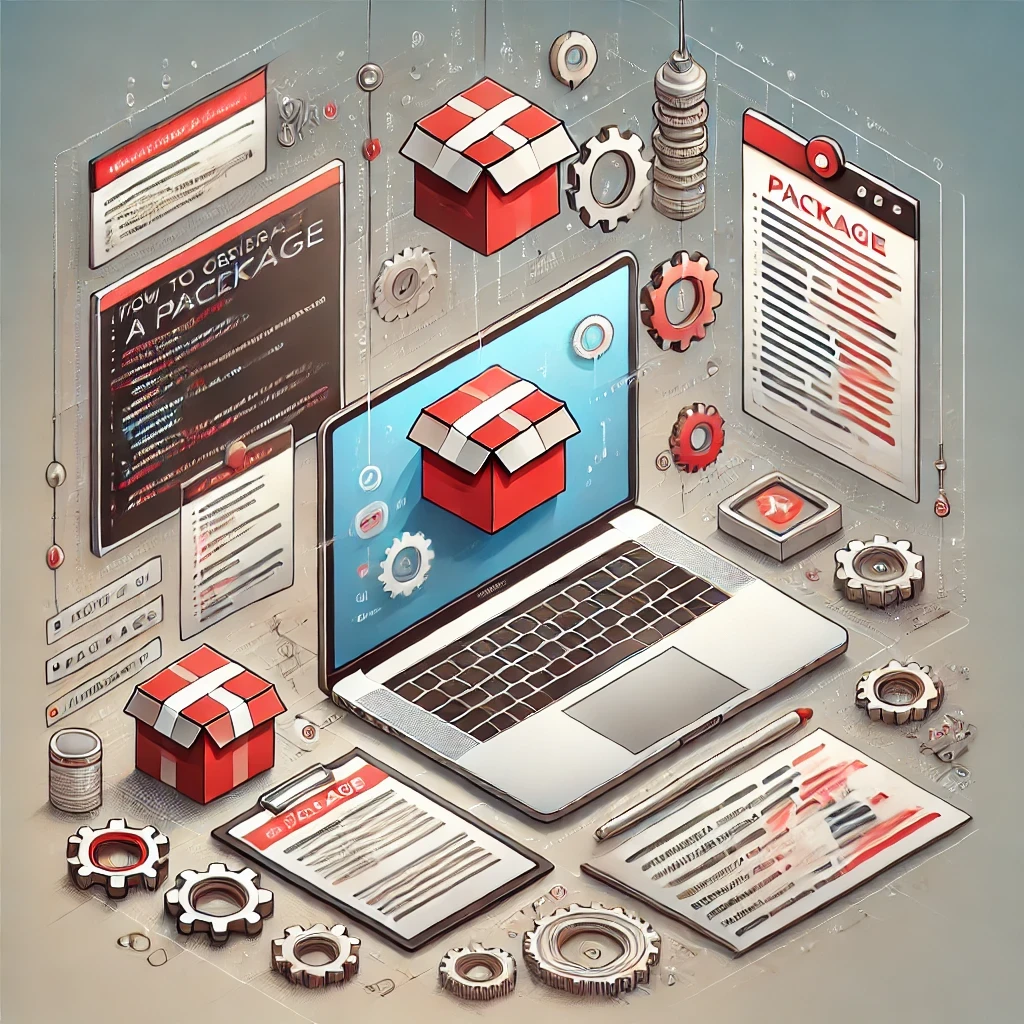
How to Generate a Package in Laravel and Why It's Important
Manish Kumar
22 Jul 2024
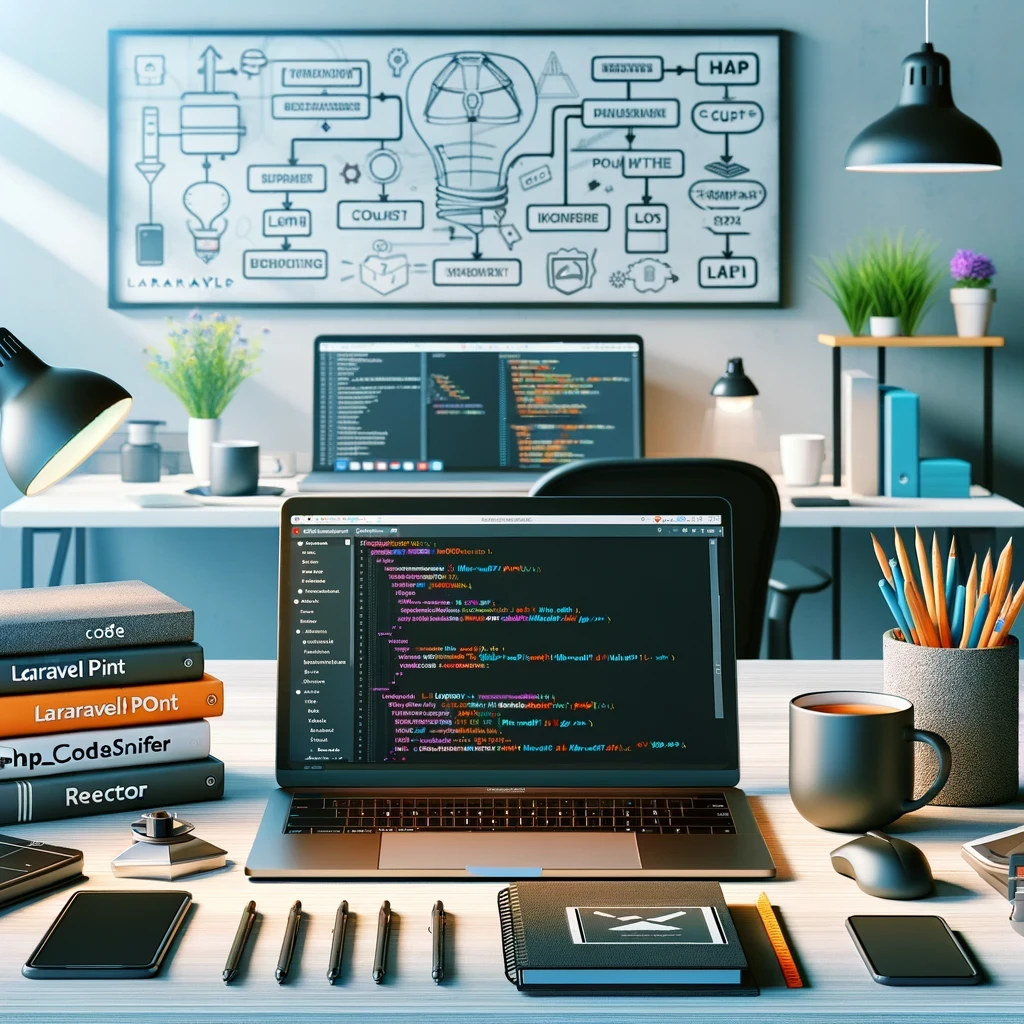
Writing Clean Code in Laravel: Principles and Tools
Manish Kumar
15 Jun 2024
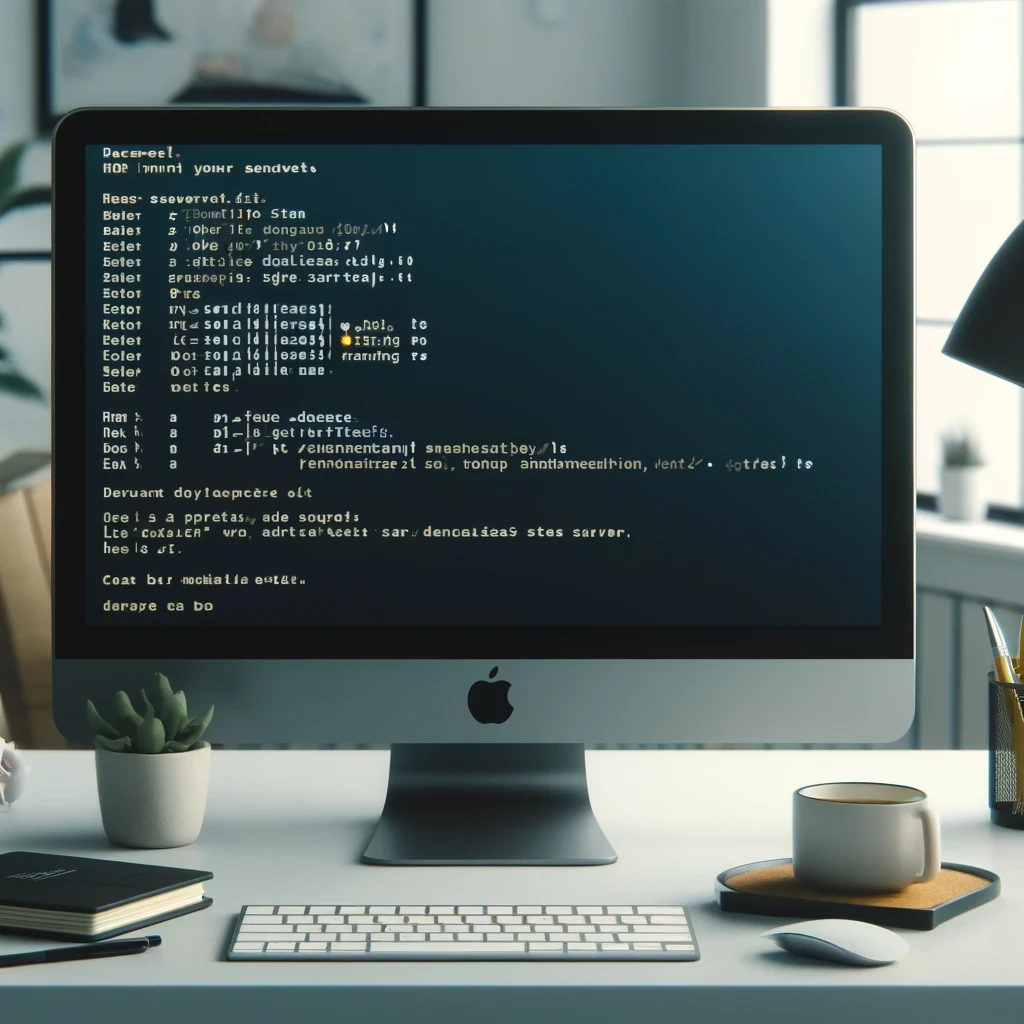
Basic Useful Commands for Developers
Manish Kumar
12 Jun 2024
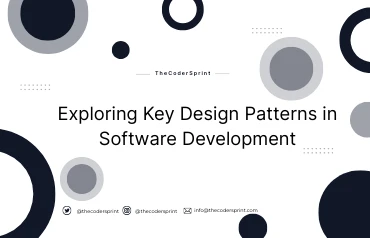
Exploring Key Design Patterns in Software Development
Manish Kumar
29 May 2024
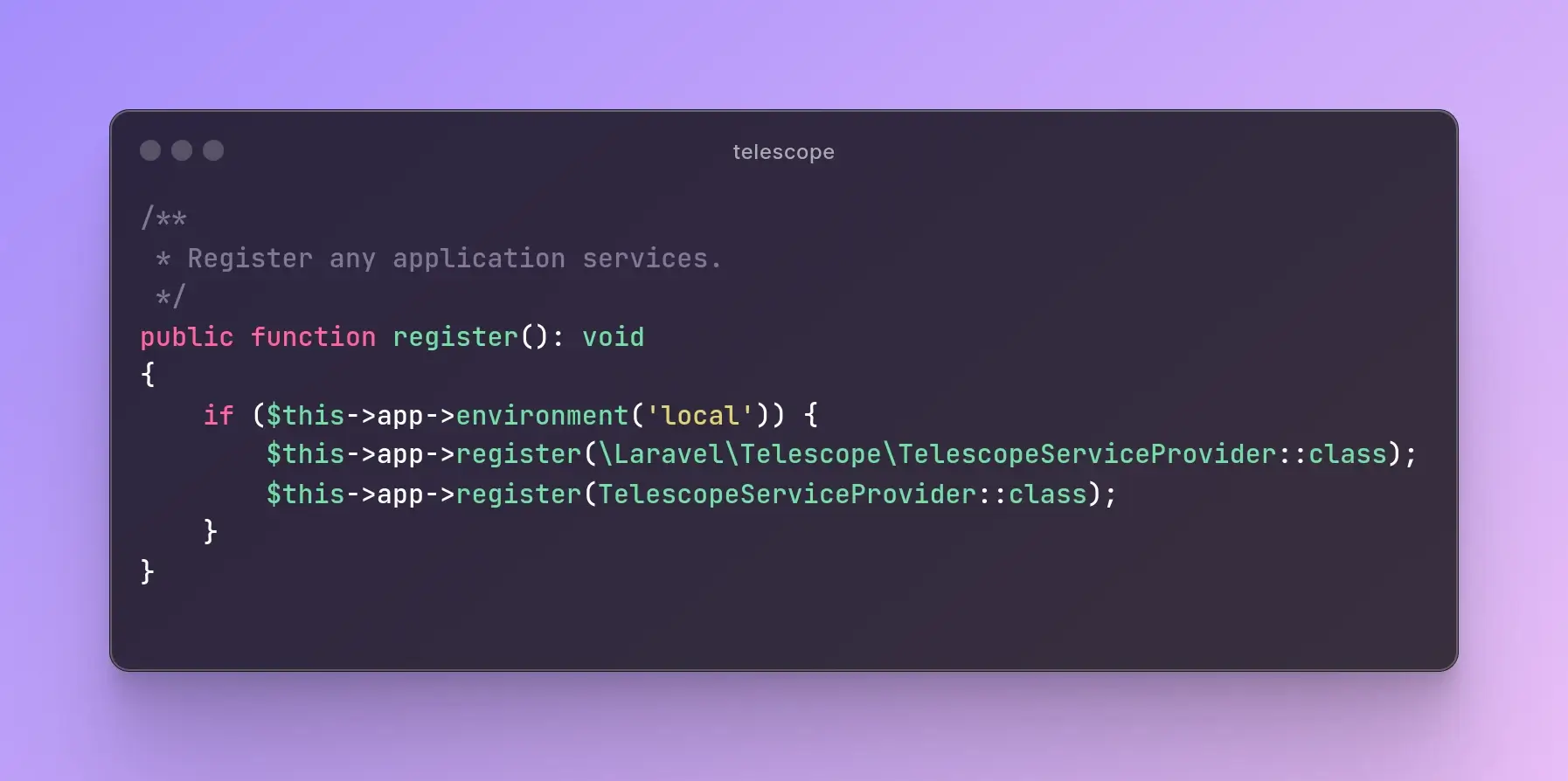
Laravel Telescope: Debugging Made Easy (Part - 2)
Sonu Singh
25 Apr 2024
Subscribe to Newsletter
Provide your email to get email notification when we launch new products or publish new articles